While developing a React App, we sometimes need to get the data in real-time or as soon as it is ready. For this one of the methods can be to hit the API in certain intervals till we get the desired response. but it is not a good solution, So, this is where WebSockets can be implemented. In this tutorial, we will be discussing WebSockets and how to implement WebSockets in React app.
What is WebSocket?
WebSocket is a full-duplex communication protocol that provides a bidirectional connection between the server and a client. That means data can be sent from client to server and server to client. The connection between the client and server remains alive until the server or a client terminates the connection. The current API specification that allows the web applications to use this protocol is WebSockets. It starts from ws:// or wss://.
Also Read: Setup Webhook support on backend with nodejs, lambda and aws api gateway
How to Implement WebSockets in React
This tutorial assumes that you have
- Understanding of react
- Handling API response
- A working react project
Step 1: Install react-use-websocket in your project
npm install react-use-websocket
Step 2: Connection to WebSocket
import "./App.css";
import useWebSocket from "react-use-websocket";
function App() {
//Public API that will echo messages sent to it back to the client
const socketUrl = "wss://www.cryptofacilities.com/ws/v1";
const { sendMessage, lastMessage, readyState } = useWebSocket(socketUrl, {
onOpen: () => {
console.log("connection opened");
},
share: true,
onMessage: (message) => {
//handle response
console.log(message);
},
onClose: () => {
//handle connection termination
console.log("connection closed");
},
//Will attempt to reconnect on all close events, such as server shutting down
shouldReconnect: (closeEvent) => true,
});
return (
<div className="App">
<h1>WebSocket Implementation on React</h1>
</div>
);
}
export default App;
Output

Here, onOpen()
the function executes when the connection to the WebSocket is opened. The onMessage()
function handles the response sent by the server and onClose()
function handles the termination of the connection.
Step 3: Handling the response from the server
import "./App.css";
import useWebSocket from "react-use-websocket";
import { useState } from "react";
function App() {
const [response , setResponse] = useState("");
//Public API that will echo messages sent to it back to the client
const socketUrl = "wss://www.cryptofacilities.com/ws/v1";
const { sendMessage, lastMessage, readyState } = useWebSocket(socketUrl, {
onOpen: () => {
console.log("connection opened");
},
share: true,
onMessage: (message) => {
const data = JSON.parse(message?.data);
setResponse(data.event)
},
onClose: () => {
console.log("connection closed");
},
//Will attempt to reconnect on all close events, such as server shutting down
shouldReconnect: (closeEvent) => true,
});
return (
<div className="App">
<h1>WebSocket Implementation on React</h1>
<h2>response: {response}</h2>
</div>
);
}
export default App;
Output
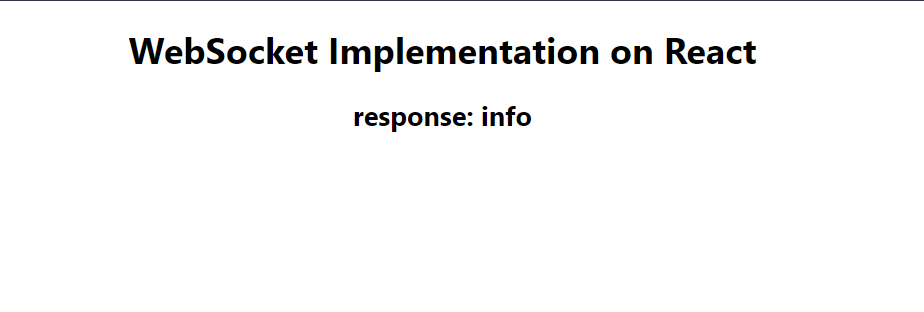
Conclusion
In this tutorial, we learned about the concept of web socket and a tutorial on how to implement WebSocket in a react app. We used react-use-websocket for this tutorial and discussed its function onOpen()
, onMessage()
and onClose()
that handles the connection open, response and connection termination.