Let’s learn how to implement a cache in Nodejs using a node-cache package. The caching will be done without the use of redis. Though if you want to learn how to implement caching in Nodejs using the redis datastore. You can look at this article Implement Redis as Cache in Node.js.
Introduction
Before getting into the integration of caching in Nodejs, let’s first learn what a cache is. A cache is basically a fast storage layer, which can send data back to the client without any processing. In caching there are two concepts to remember first one is “cache hit” and the next is “cache miss”. A cache hit occurs when data that the user is looking for is found in the cache storage. A cache miss occurs when the data that the user is looking for is not found in the cache.
let’s look at how caching on the server side generally works.
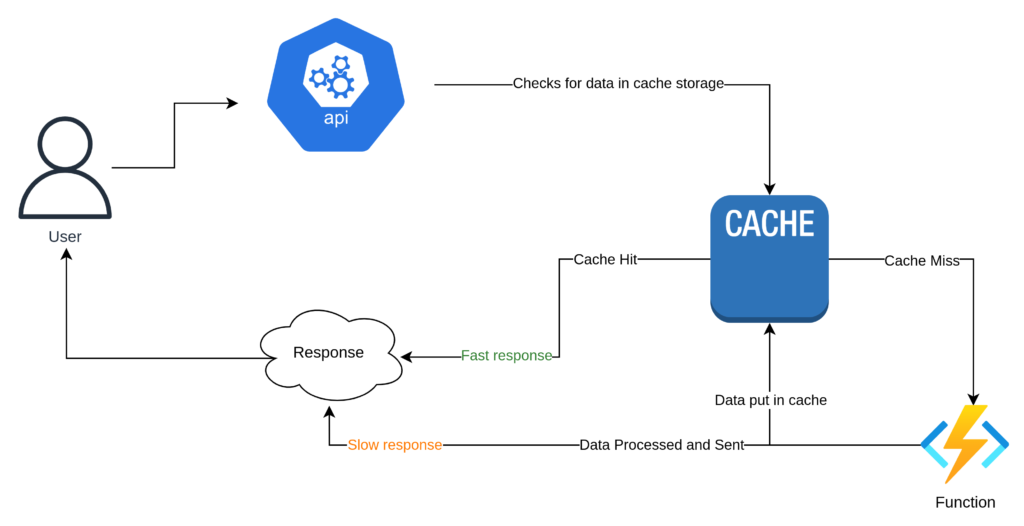
From the representation we can see, when the user invokes an API, it first checks the cache storage regarding the availability of data. If the data is found on the cache it returns data. In the case where there is no data found in the cache, it runs the function which was to run if there was no caching involved. After the function runs it gets the data, puts it in the cache, and returns it back to the user. When the cache is missed, the response time is slow, because of the multiple steps involved. But when the cache is hit the response is fast because of the straight cache to response, without any other processing elements.
Prerequisites
- Basic knowledge of Nodejs
Implement a Cache in Nodejs
To implement a cache in Nodejs we will be using a package called node-cache
. Let’s first initialize a node project. Secondly, install the required packages, and finally start the implementation.
## Creating directory for the node project
$ mkdir caching-node
$ cd caching-node
## Initializing a node project
$ npm init --y
Wrote to /../../../caching-node/package.json:
{
"name": "caching-node",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
## Installing required npm packages
$ npm install node-cache express axios
added 66 packages, and audited 67 packages in 2s
8 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
## Creating a starting point for the application
$ touch index.js
Now that the project is initialized, packages are installed, and a starting point for the application index.js
is created. Open the project with your favorite code editor. Let’s first create an express application by importing all the required packages.
//* index.js
const express = require("express");
const axios = require("axios");
const nodeCache = require("node-cache");
const app = express(); //* Initializing express
const cache = new nodeCache(); //* Initializing the cache
const PORT = process.env.PORT || 3000; //* Server port
const requestUrl = "https://jsonplaceholder.typicode.com/posts"; //*
//* all the routes go here
app.listen(PORT, () => console.log(`Server started on PORT ::${PORT}`));
Now, that the express application is created, let’s first look at how data is put in the cache, and retrieving it from the cache.
Inserting and Retrieving from the Cache
We will be implementing it in the get route.
//* Inserting and Retrieving from the cache
app.get("/", (req, res) => {
const cachedData = cache.get("/");
//* Data is present inside the cache
if (!!cachedData) {
console.log("Cache Hit");
return res.status(200).json(cachedData);
}
//* Data is not present inside the cache
console.log("Cache Miss");
axios
.get(requestUrl)
.then((data) => {
console.log("Putting data in cache ...");
const responseData = data.data;
//* Putting data inside the cache
cache.set("/", responseData);
return res.status(200).json(responseData);
})
.catch((error) => {
return res.status(500).json(error);
});
});
The data retrieval from the cache is done from the get()
method provided by the node-cache
package. The get method takes a key as a parameter. If it gets the data then it returns the data which was put in that key previously.
Inserting data into the cache is done by the set()
method. It takes in (key, object, ttl)
as a parameter. The key is used for retrieval, and deleting of the value from the cache. The object is the cached data. Finally, we see ttl
it tells how long the data will be present in seconds inside the cache before expiring. Let’s look at the result of running the above.
Result of Inserting and Retrieving data from the Cache
Route Hit: http://127.0.0.1:3000/
or localhost:3000/
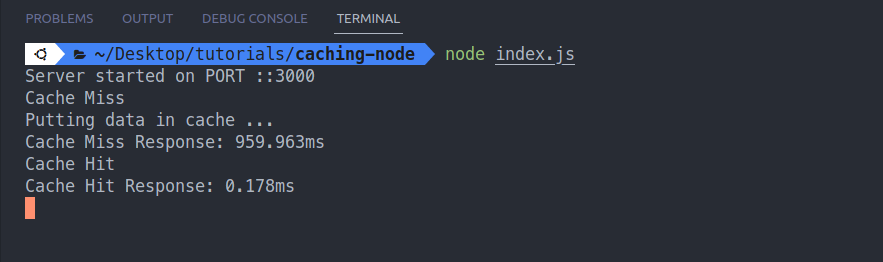
We can see from the obtained result we can see that when data is present in the cache the response time is rapid. When data is not present inside the cache it takes 959ms
to retrieve and return the response back to the client. In cases where data is present inside the cache, the time taken for processing and response is 0.173ms
which is much faster.
Deleting from the Cache
We have looked into inserting data into the cache and retrieving it. Now, let’s look into deleting data from the cache. We will be implementing it in the delete route.
//* deleting data from the cache
app.delete("/", (req, res) => {
console.log("Deleting from the cache");
cache.del("/");
return res.status(200).send("deleted");
});
For deleting data present in the cache the del()
method is used. This takes in a key as a parameter and removes the object or data present in that key. This basically removes data from the key if it finds else it does nothing. So, let’s hit the route and test is the cache is removed or not, by caching data then removing it, then again fetching to see if the cached data is found or not.
Result of Deleting data from the cache
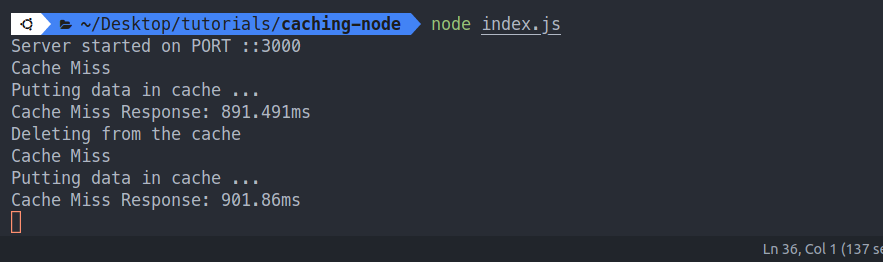
From the above figure, we can see, when we first cache the data. Then afterward we delete the key where the data is present. Then again we hit the get route, and from it, we see that the data is not present in the cache.
Flushing from the Cache
Flushing means removing all data from the cache. When the cache is flushed not a single key-value pair remains inside the cache.
cache.flushAll();
Using this flushes all cache data from the cache.
Conclusion
So, in this article, we touch on implementing a cache in Nodejs using the node-cache
package. We looked at adding, retrieving, deleting, and flushing data from the cache.