In this article, we will learn how to generate sitemap using node js. Node.js (Node) is a platform for executing JavaScript code server-side, which is ideal for developing applications that require a persistent connection between the browser and the server and is commonly used in real-time applications like chats, news feeds, and push notifications.
The sitemap contains information about the pages, videos, and other files on your site, including their relationships. This file is read by search engines like Google so they can crawl your site more efficiently. In addition to providing valuable information about your site’s files and pages, a sitemap tells Google where they can find the important pages and files.
Prerequisites
- A basic understanding of Node JS
How To Generate Sitemap Using Node JS?
Project Setup
Create a directory named node-sitemap-generate
and change it into that directory using the following command.
mkdir node-sitemap-generate
cd node-sitemap-generate
Inside the node-sitemap-generate
directory, initialize a node js project using
npm init
After the command, a new package.json file will be created in the node-sitemap-generate
the folder which will contain the following block
#package.json
{
"name": "sitemap-generate",
"version": "1.0.0",
"description": "How to generate sitemap using node js",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"xml2js": "^0.4.23"
}
}
Now, let’s install the required dependencies required to generate a sitemap.
npm install xml2js
This will install xml2js modules which will be used to parse XML to JS objects using which we will be able to add a new URL of our website in our sitemap file.
Now, let’s create a sample sitemap.xml file that will contain the urlset. The file will contain the following data in the beginning.
<urlset xmlns="https://www.sitemaps.org/schemas/sitemap/0.9" xmlns:news="https://www.google.com/schemas/sitemap-news/0.9" xmlns:xhtml="https://www.w3.org/1999/xhtml" xmlns:image="https://www.google.com/schemas/sitemap-image/1.1" xmlns:video="https://www.google.com/schemas/sitemap-video/1.1">
<url>
<loc>YOUR-SITE-URL</loc>
<changefreq>daily</changefreq>
<priority>0.3</priority>
</url>
</urlset>
Next, let’s create a index.js
file where we will write the logic using which the new URL will be appended in the above file every time we execute the index.js file.
const fs = require('fs');
const xml2js = require('xml2js');
async function sitemap() {
const data = fs.readFileSync('./sitemap.xml', 'utf8');
const newData = {
loc: [
'NEW-URL'
],
changefreq: ["daily"],
priority: ["0.3"],
};
const xmlObj = await xml2js.parseStringPromise(data);
xmlObj.urlset.url.push(newData);
console.log(xmlObj.urlset.url);
const builder = new xml2js.Builder({
xmldec: { version: "1.0", encoding: "UTF-8" },
});
const xml = builder.buildObject(xmlObj);
fs.writeFileSync(`./sitemap.xml`, xml);
}
sitemap();
Here, we have performed the following task.
- We read the content of the sitemap file and prepare the new URL data to be added to the sitemap file. Here, the
changefreq
andpriority
are optional parameters that define how frequently the page is likely to change and the priority of the URL respectively. The priority values can be between 0.0 to 1.0. - Then, using xml2js extension we will parse the XML file into a js object so that we can easily manipulate it.
- After that, we append the new data into the js object and create the XML data using the builder object. The newly created XML is then written in the sitemap.xml file using the writeFileSync command provided by node JS.
- Now, every time you execute the
index.js
file the data you prepare will be appended in the sitemap.xml file as shown below
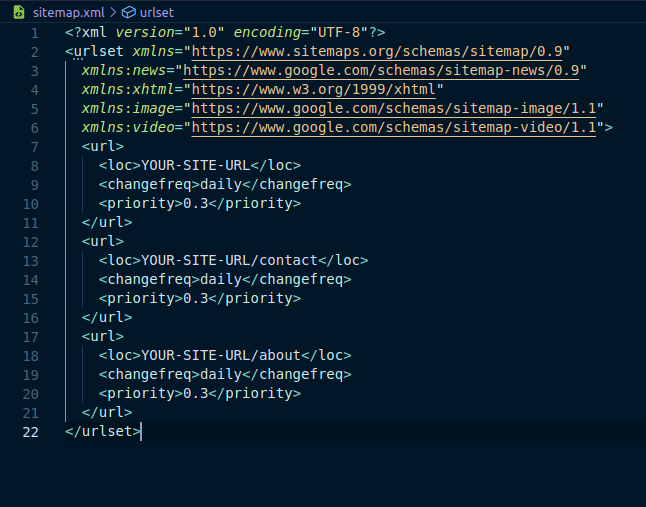
Conclusion
In this article, we have learned how to generate sitemap using node js. This is one of the approaches using which we can generate the sitemap. In this approach, you do not need to access the database every time the sitemap listing URL is executed. You can follow this link to learn more about node js.