In this article, we will be discussing react hook form. We will be covering the basic understanding of react hook form and its implementation.
What is React Hook Form?
React Hook Form is a library that helps to handle and validate forms. It is a small library without any dependencies. It reduces the number of codes compared to other libraries and prevents unnecessary re-rendering. React Hook Form provides a hook called useForm()
that consists of methods and props like register, handleSubmit, and errors. They would handle the inputs, submit functions and handle errors.
React Hook Form vs Formik
In one of our previous articles, we discussed formik, also a react form library. One of the major issues of formik is that it re-renders the component on every change and also it requires quite a significant amount of boilerplate codes. React Hook Form isolates a component and avoids other components from unnecessary re-rendering which will improve the performance. Formik requires third-party libraries to validate the form whereas React Hook Form has a built-in feature for validation.
Implementing React Hook Form
Let’s create a simple form using react hook form.
Step 1: Install react-hook-form
npm install react-hook-form
Step 2: Import useForm From react-hook-form Package
import { useForm } from "react-hook-form";
Step 3: Declare useForm Methods Inside Your Component
const {register, handleSubmit, watch, reset, formState: { errors }} = useForm();
useForm()
hook returns objects containing some properties.
For this tutorial, we will be using a register, handleSumbit, watch, reset, and formState. register
helps to register an input field to react hook form and store its value. we also validate an input field inside register
. handleSubmit
handles the submission of form data. It is passed as a value to onSubmit props. watch
is used to watch the value of an input field by passing its name. reset
resets the form input fields. formState
contains information about the entire form state. errors
returns the object of errors in input fields that occurred due to validations defined in the register
.
Step 4: Create a Form
import React from "react";
import { useForm } from "react-hook-form";
function Form() {
const {register, handleSubmit, watch, reset, formState: { errors }} = useForm();
console.log(watch("name")); // watch input value by passing the name of it
return (
<div className="d-flex flex-column align-items-center">
<h3>React Hook Form</h3>
{/* "handleSubmit" will validate your inputs before invoking "onSubmit" */}
<form
onSubmit={handleSubmit((data) => console.log(data))}
className="d-flex flex-column col-4 gap-2 text-align-center"
>
{/* register your input into the hook by invoking the "register" function and
redux include validation with required or other standard HTML validation rules*/}
<input
type="text"
{...register("name", { required: "This field is required" })}
placeholder=" Name"
/>
{/* errors will return when field validation fails */}
{errors?.name?.message && (
<div className="text-danger">{errors.name.message}</div>
)}
<input
type="text"
{...register("phone", {
required: "This field is required",
minLength: { value: 10, message: "Invalid Number" },
maxLength: { value: 10, message: "Invalid Number" },
})}
placeholder=" Phone Number"
/>
{errors?.phone?.message && (
<div className="text-danger">{errors.phone.message}</div>
)}
<input
type="password"
{...register("password", { required: "This field is required" })}
placeholder=" Password"
/>
{errors?.password?.message && (
<div className="text-danger">{errors.password.message}</div>
)}
<button type="submit">Submit</button>
<input type="button" value="Reset Form" onClick={() => reset()} />
</form>
</div>
);
}
export default Form;
Here, we have created input fields for Name, Phone, and Password and buttons for submit and reset. We have validated the input fields using required, minLength, and maxLength and displayed the errors.
Output
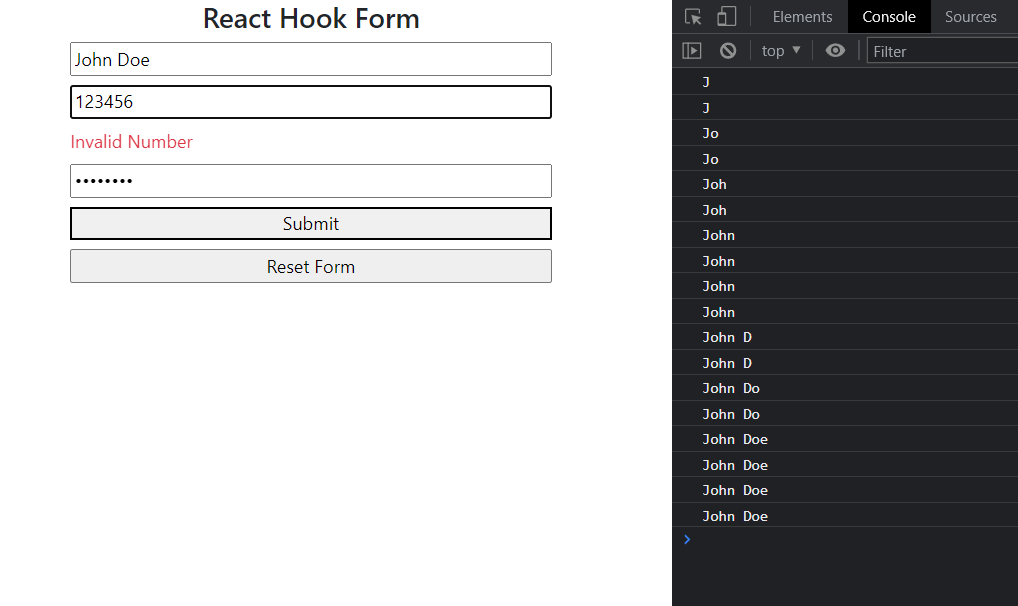
How to Implement Validation Rules in React Hook Form.
Some of the basic validation rules that supported in react hook form are: required, min, max, minLength, maxLength, pattern. We will be covering one by one for each of them below.
required
It accepts boolean which, if true, indicates that the input value is required to submit the form. You can also assign a string to return an error message in the errors
object.
<input
{...register("name", {required: true})}
/>
//OR
<input
{...register("name", {required: "This field is required"})}
/>
min
It declares the minimum value to accept for the input.
<input
type="number"
{...register("age", {
min: {
value: 3,
message: 'error message'
}
})}
/>
max
It declares the maximum value to accept for the input.
<input
type="number"
{...register('age', {
max: {
value: 10,
message: 'error message'
}
})}
/>
minLength
It declares the minimum length of the value to accept for the input.
<input
{...register("name", {
minLength: {
value: 4,
message: 'error message'
}
})}
/>
maxLength
It declares the maximum length of the value to accept for the input.
<input
{...register("name", {
maxLength : {
value: 20,
message: 'error message'
}
})}
/>
pattern
It declares the regex pattern for the input.
<input
{...register("test", {
pattern: {
value: /[A-Za-z]{3}/,
message: 'error message'
}
})}
/>
More validation rules can be found on here.
Conclusion
In this article, we discussed the basic understanding of react hook form and its implementation. We now understand that it is easier to manage and validate a form using react hook form library. More on react hook form can be found on its official site.