What is form validation?
Form validation is a method to ensure that the data submitted by user meets specific criteria. The common practice of validating a form in react.js is to create a separate state for every input field and then creating a validation function and error condition for every one of them. It is a good method for the form with less validation conditions.
What is Formik?
Formik helps you to write the three most annoying parts of building a form: handling states, Validation and error messages, Handling form submission. Formik uses initialValues, validationSchema and onSubmit to handle these. The values of the input fields are captured automatically and is stored in the values of the Formik. The input fields are identified with their unique names that should be provided by the user.
What is Yup?
Yup is a JavaScript object schema validator. It helps us create custom validation rules.
Simplest way to use Yup:
- Define object schema and its validation.
- Create validator object using Yup with expected schema and validation.
- Use Yup utility function “validate” to verify if object are valid(satisfies schema and validations).
Form validation with Formik and Yup in React.js
Lets create a simple form and validate it using formik and yup. and we will begin it by creating the react app and installing the formik and yup.
npx create-react-app my-form
npm i formik
npm i yup
import React from "react";
import { Formik, Form } from "formik";
import TextField from "../components/Form/TextField";
import {validateForm} from "../validation/validateForm";
function Formikform() {
return (
<div>
<Formik
initialValues={{
firstName:"",
lastName:"",
email:"",
phone:"",
password:"",
}}
validationSchema={validateForm}
onSubmit={(values) => console.log("form values ==>" , values)}
>
{(formik) => (
<Form>
<TextField label={"First Name"} name={"firstName"} type={"text"} />
<TextField label={"Last Name"} name={"lastName"} type={"text"} />
<TextField label={"Email"} name={"email"} type={"text"} />
<TextField label={"Phone Number"} name={"phone"} type={"text"} />
<TextField label={"Password"} name={"password"} type={"password"} />
<button type="submit" className="btn btn-primary mt-2">Submit</button>
</Form>
)}
</Formik>
</div>
);
}
export default Formikform;
The TextField is a custom created component of the input field. The code for the TextField can be seen below:
import React from 'react'
import {ErrorMessage , useField} from 'formik'
function TextField({label , ...props}) {
const [field , meta] = useField(props);
return (
<div className='mt-2'>
<label htmlFor={field.name}>{label}</label>
<input className= {`form-control shadow-none mt-1 ${meta.touched && meta.error && 'is-invalid'}`} {...field} {...props} autoComplete='off'/>
<ErrorMessage component='div' name={field.name} style={{color:"red" , fontSize:"12px"}}/>
</div>
)
}
export default TextField
The validation schema is created using yup. The name provided in the formik should match the names used in the validation schema.
import * as Yup from "yup";
export const validateForm = Yup.object().shape({
firstName: Yup.string()
.min(2, "Too Short!")
.max(50, "Too Long!")
.required("Firstname is required"),
lastName: Yup.string()
.min(2, "Too Short!")
.max(50, "Too Long!")
.required("Lastname is required"),
phone: Yup.string()
.required("Phone number is required")
.matches(
/^(\+?\d{0,4})?\s?-?\s?(\(?\d{3}\)?)\s?-?\s?(\(?\d{3}\)?)\s?-?\s?(\(?\d{4}\)?)?$/,
"Invalid phone number"
),
email: Yup.string().email().required("Email is required"),
password: Yup.string()
.required("Password is required")
.min(6, "Password is too short - should be 6 chars minimum"),
});
The form looks like:
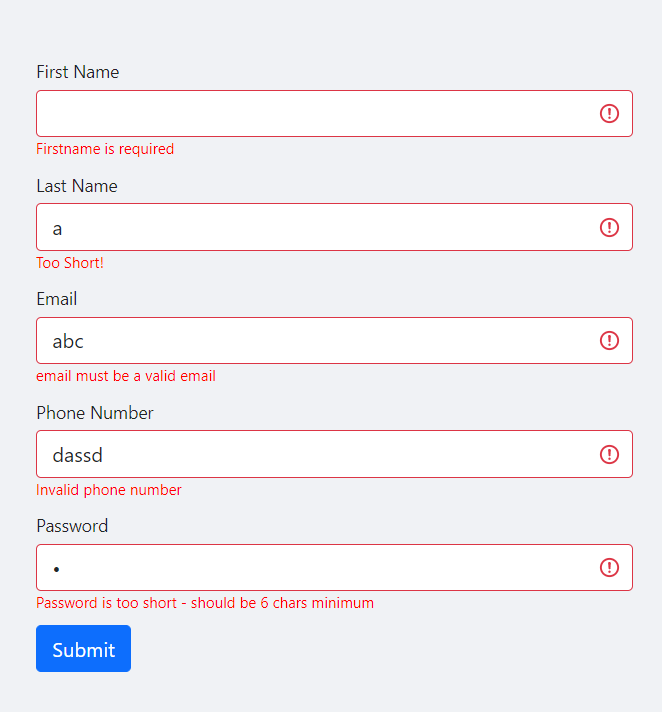
Here, we can see that the provided values do not satisfy the conditions provided in the yup validation schema so it gives the error as provided by the validation schema. Only when the validation conditions are fulfilled, we can submit the form and the form values can be handled in the onSubmit function of the Formik as show in the image below.
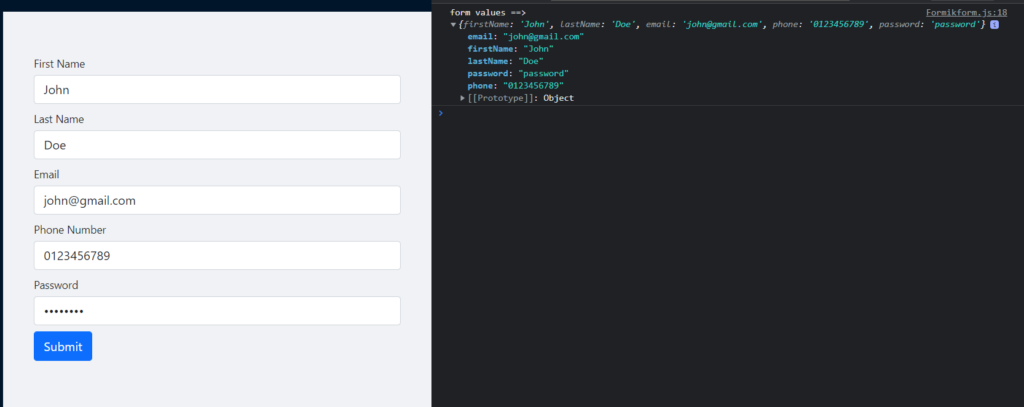
Conclusion
In this article we learned how to validate a form using formik and yup in React.js. The Formik handles the form using initialValues to store the initial state of the input field values , validationSchema for validation condtions (using yup) and onSubmit to handle the values of the form.