Introduction
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes Javascript code outside the browser. Node.js helps the developer write code that runs on the server side, making it possible for server-side scripting to be achieved.
Learn how to install Node.js:
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. Let us look at using express-fileupload
middleware to upload files to s3 Bucket.
Express-fileupload
The express-fileupload library is a middleware for the Express framework. It provides an easy way to upload files to a designated location. In this tutorial, we will touch on uploading files to s3 Bucket using express-fileupload
and answer the dilemma on how to upload to S3 Bucket using express-fileupload
in Node.js.
Initializing the application
## scaffolding a express application
mkdir express-file-upload && cd express-file-upload
## initializing a node application and installing all the required dependencies
npm init -y
npm i express express-fileupload aws-sdk dotenv
## creating a starting point for the application
touch index.js .env
## open the project in your preferred code editor
code .
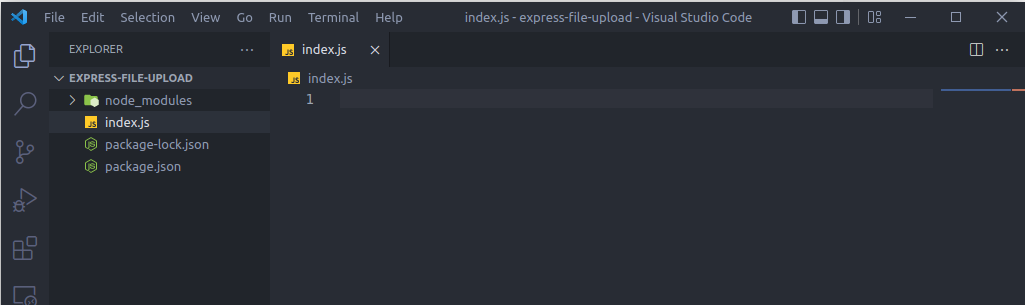
So, now that we have initialized the application let’s move forward with the actual code part of the application, showing us how to upload to S3 Bucket using express-fileupload
in Node.js for the Express framework.
Upload to S3 bucket using express-fileupload
const express = require("express");
const upload = require("express-fileupload");
const AWS = require("aws-sdk");
require("dotenv").config();
const app = express();
const PORT = process.env.PORT || 3000;
// using upload middleware
app.use(upload());
app.use(express.json());
// s3 config
const s3 = new AWS.S3({
accessKeyId: process.env.AWS_ACCESS_KEY_ID, // your AWS access id
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY, // your AWS access key
});
// actual function for uploading file
async function uploadFile(file) {
const params = {
Bucket: process.env.AWS_BUCKET, // bucket you want to upload to
Key: `fileupload/scanskill-${Date.now()}-${file.name}`, // put all image to fileupload folder with name scanskill-${Date.now()}${file.name}`
Body: file.data,
ACL: "public-read",
};
const data = await s3.upload(params).promise();
return data.Location; // returns the url location
}
app.post("/upload", async (req, res) => {
// the file when inserted from form-data comes in req.files.file
const fileLocation = await uploadFile(req.files.file);
// returning fileupload location
return res.status(200).json({ location: fileLocation });
});
// starts the express server in the designated port
app.listen(PORT, () => console.log(`server started at PORT: ${PORT}`));
Now testing the application from the postman.
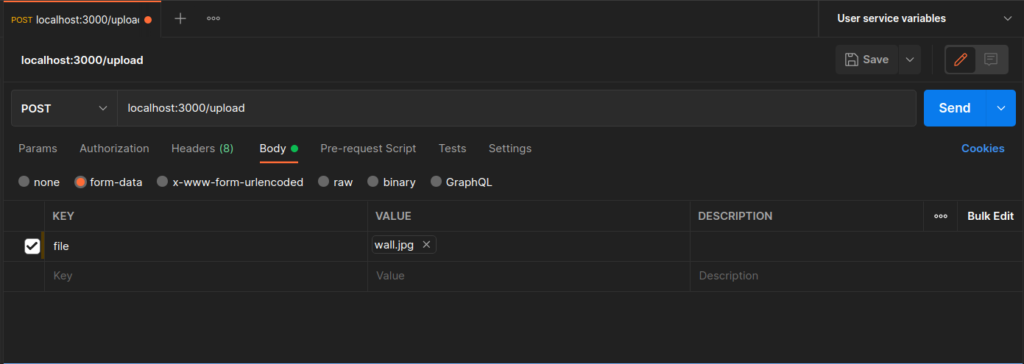
We send the request to the POST
of /upload
from postman.
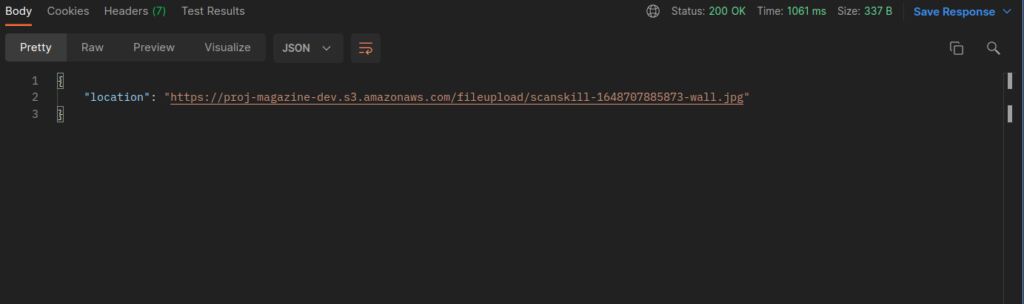
When we click the URL the file opens to download.

The new file is present in the s3 bucket.

The file is present inside the folder

Conclusion
Congratulations! you have successfully learned how to upload files in s3 in Express using express-fileupload
the package. Fulfilling the requirement on how to upload to S3 Bucket using express-fileupload
in Node.js. Now, that we have looked into uploading to an S3 bucket, importing from an S3 bucket is looked into in the beginning parts of this article.