Introduction
According to Redis themselves, Redis is an open-source (BSD licensed), in-memory data structure store used as a database, cache, message broker, and streaming engine. Redis provides data structures such According to Redis themselves, Redis is an open-source (BSD licensed), in-memory data structure store used as a database, cache, message broker, and streaming engine. Redis provides data structures such as strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs, geospatial indexes, and streams. You can also implement cache without using Redis but by using node-cache, this is looked into in Learn How to Implement a Cache in Nodejs.
Using Redis as a Cache
It is a fast, open-source, in-memory, key-value data store. Following is the list of benefits of using redis as a cache.
- High availability of data
- Vertical scaling
- Replication and persistence
- Simplicity and ease-of-use
- Performance
Use Cases of Redis
To name a few, these are the use cases of Redis but are not limited to:
- Session Management
- Caching
- Real-time analytics
- Rich media streaming
- GeoSpatial
- Machine Learning
- and many more..
Caching Using Redis
Initializing the Redis Application
## scaffolding a express application
mkdir redis-cache-impl && cd redis-cache-impl
## initializing a node application and installing all the required dependencies
npm init -y
npm i express redis axios
## creating a starting point for the application
touch index.js
## open the project in your preferred code editor
code .
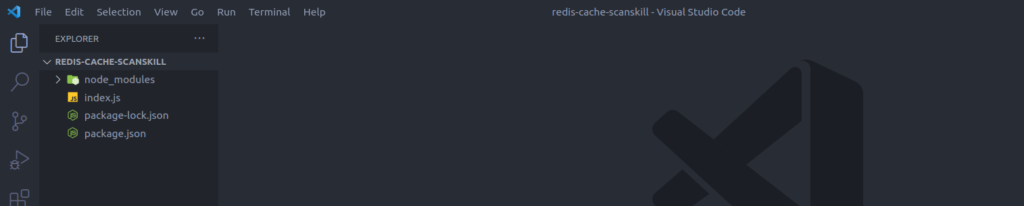
Packages Used:
- redis: Package used for interaction with Redis data store.
- axios: Package used for fetching data.
- express: Express is a Node.js web application framework it is the base for this project
Prerequisites/Requirements:
- Access to Redis data store
- Node installed and basic knowledge of Node.js and Express.js framework
- Basic knowledge of how Axios works
Implementation of Redis Cache
const express = require("express");
const redis = require("redis");
const axios = require("axios");
const app = express();
const requestUrl = "https://jsonplaceholder.typicode.com/posts";
//* configuring and creating redis client
const client = redis.createClient({
host: "127.0.0.1", //* Redis Host URL
port: 6379, //* Redis Host PORT number
password: null, //* Host password null if empty
});
//* connecting to the redis data store
function redisConnection() {
client.connect();
console.log("Connection made with Redis");
}
app.get("/", async (req, res) => {
//* mapping redis key according to request url
const key = req.url;
//* getting data from the cache if cache is present for the given key
const cachedData = await client.get(key);
if (cachedData) {
console.log("!!! Cache Hit !!!");
//* parsing data as data is saved in string format in redis
return res.status(200).json(JSON.parse(cachedData));
}
//* fetching data from the requestUrl
axios
.get(requestUrl)
.then((data) => {
console.log("cache miss");
//* putting data in cache in string format
client.set(key, JSON.stringify(data.data));
console.log("Putting data in cache ...");
return res.status(200).json(data.data);
})
.catch((error) => {
return res.status(500).json(error);
});
});
//* starting the express server and making connection to Redis data store
app.listen(3000, () => {
console.log("Server Started at port 3000");
redisConnection();
});
Output
Sending First Request
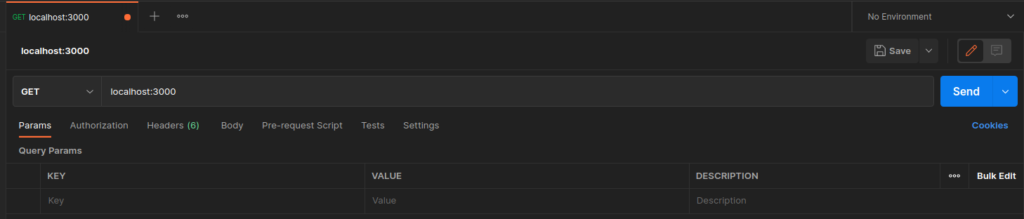
Cache Miss on the first request and putting data in Redis
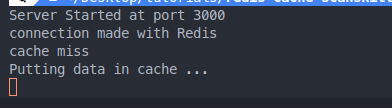
First Request Response
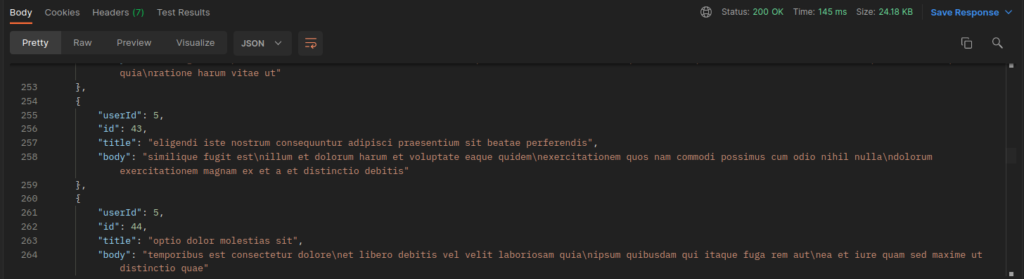
Request Response time
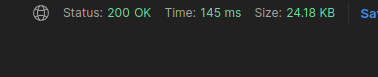
Second Request to the server
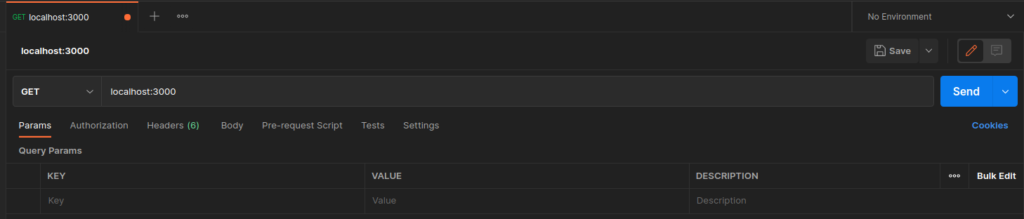
Data found in cache
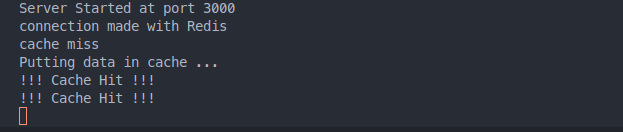
Response after data found in Cache
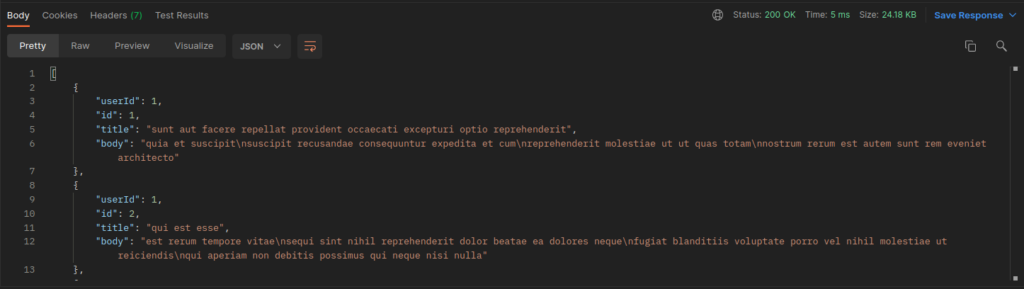
Data Found in Redis Cache response time

From the above Requests made to the server, we can see that the Redis cache reduces time by a huge margin. When the request was sent and the data was not present in the cache it took 145ms to return data back to the user as a response. After the Redis cache was implemented the data was returned in 5ms. From this, we can draw conclusions that the previously listed benefits like high availability of data, vertical scaling, replication and persistence, simplicity and ease of use, and performance were in fact true.
Conclusion
Congratulations! you have successfully learned how to implement caching in the Redis data store using Node.js. We also looked into the benefits of using caching, implemented it, and moreover proved that the listed benefits of performance were true. Now the remaining part of caching is removing data from the cache. So, more articles on Redis are yet to come, on topics like flushing data, deleting data, deleting data based on patterns, and much more.