Let’s learn how to create REST API in node.js using AWS API Gateway. We will look at connecting API Gateway with a Lambda function.
Introduction
AWS API Gateway is a fully managed service that enables developers to easily design, publish, maintain, monitor, and protect APIs of any size. APIs serve as the “front door” through which apps access data, business logic, or functionality from your backend services. AWS API Gateway allows you to construct RESTful APIs and WebSocket APIs for real-time two-way communication applications. API Gateway supports containerized and serverless workloads, as well as web applications.
In this article, we are going to look at connecting AWS API Gateway with a Lambda function. Through this, we are going to look at creating REST API in Node.js using API Gateway.
Prerequisites
AWS API Gateway
Creating an API
Firstly search for API Gateway on the AWS consoles dashboards search bar, and click on it. Clicking on it will redirect you to the Amazon API Gateway dashboard.
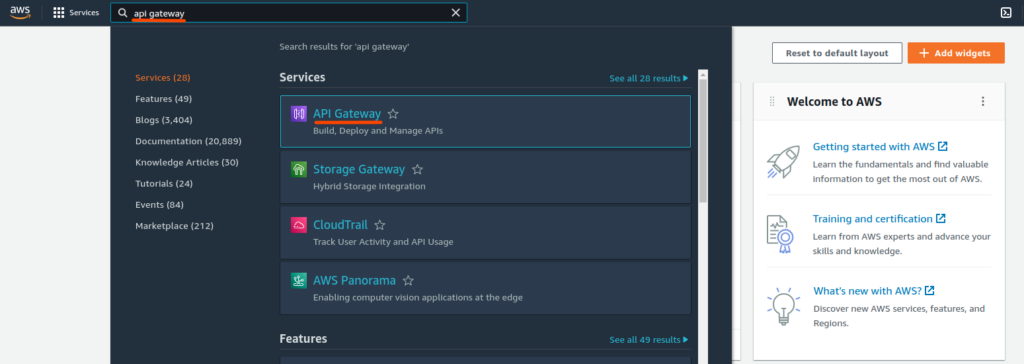
There are multiple options to choose from as shown in the image below.
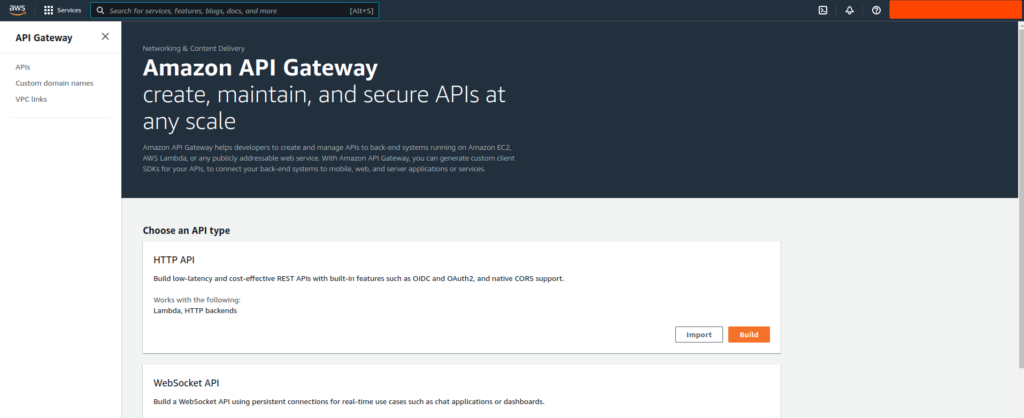
Let’s select the REST API, and click on the build button. After completing the steps, you will be redirected to the create page.
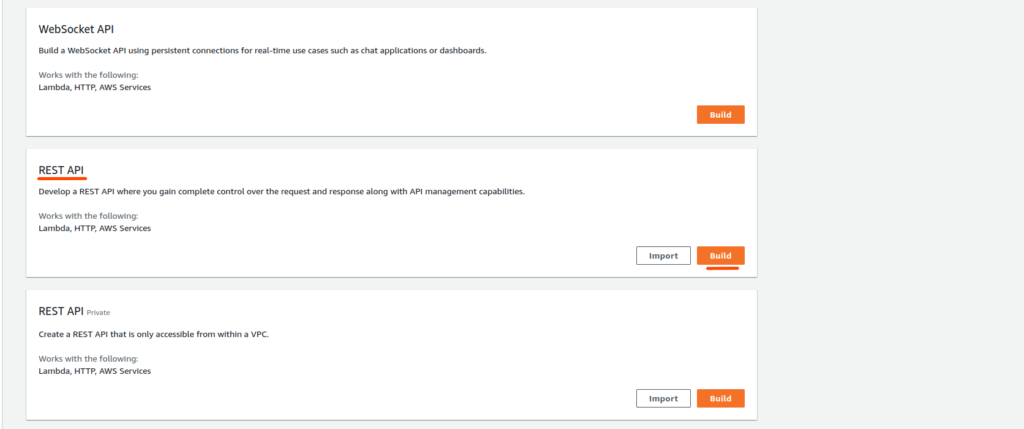
Choose the REST
option in the choose the protocol section, and in the create new API section choose the option New API
. Give the API a name, and description and leave other options as it is as shown in the image below.
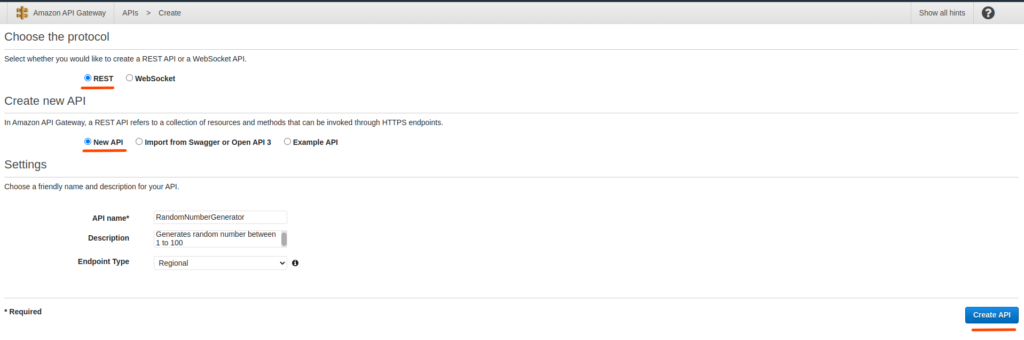
After The created API is done, you are redirected to the API Gateways API page, where you can create endpoints like GET, POST, PUT, DELETE, etc.
Creating HTTP Method GET
Let’s create a GET method, and set it up together with a Lambda.
For that click on the create method option present inside the actions dropdown as shown below.
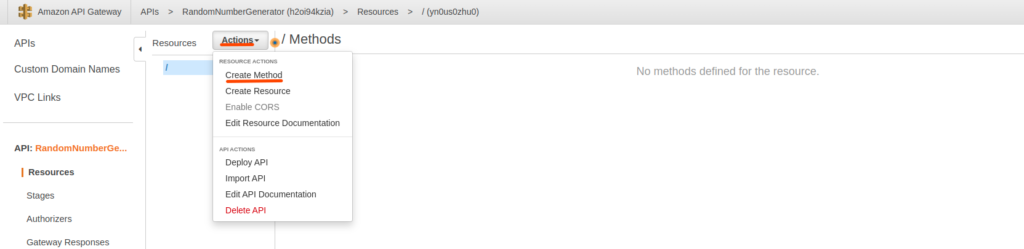
Then choose the GET option as shown in the image below, then click on the button with a checkmark, which is present on the right side of the dropdown.

Afterward, you can set up the newly created method. In this article, we are going to trigger a Lambda through the API Gateway, so in the case of this article we are going to choose the integration type as Lambda Function
.
Using this option we can connect it to a pre-existing lambda function, or we can create a new one.
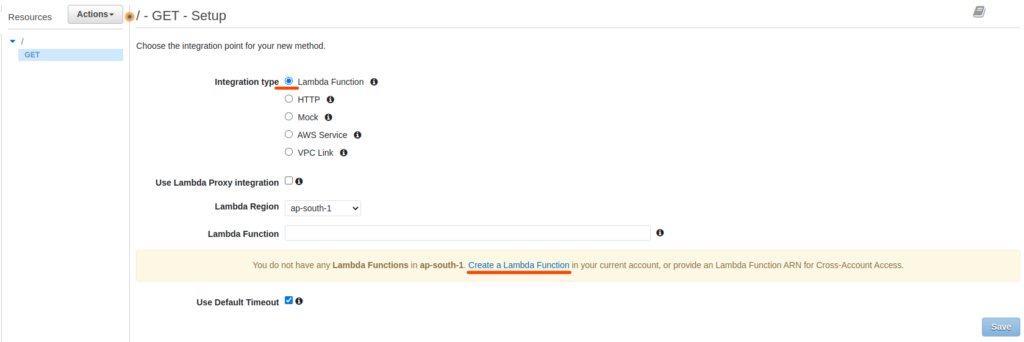
We will create a new Lambda function, and the steps are listed in the topic below.
Connecting HTTP Method with a Lambda
For connecting the Lambda to the API Gateway let us create a lambda from scratch.
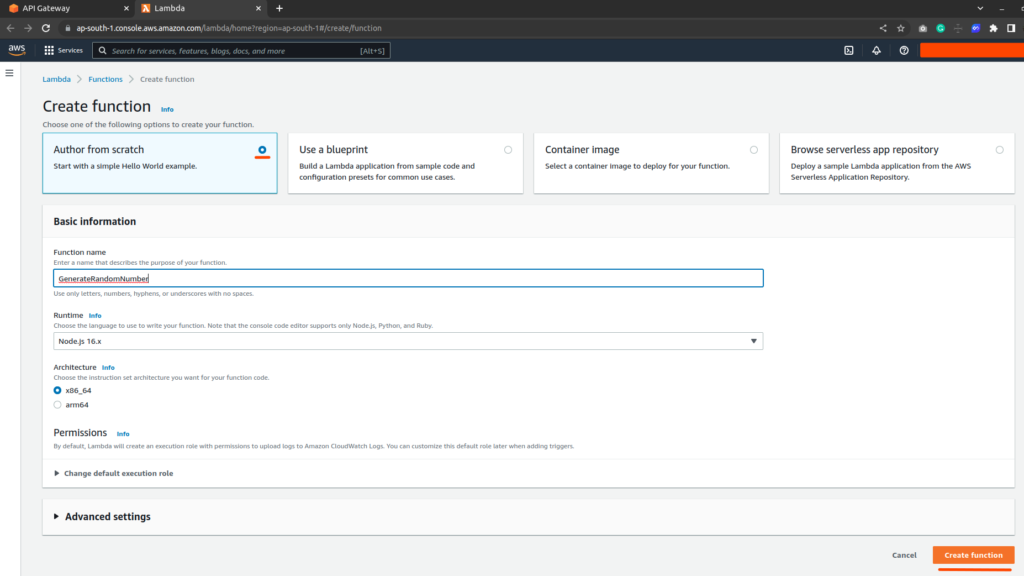
According to your need, you can select the functions name, node version, and the architecture it will run on.
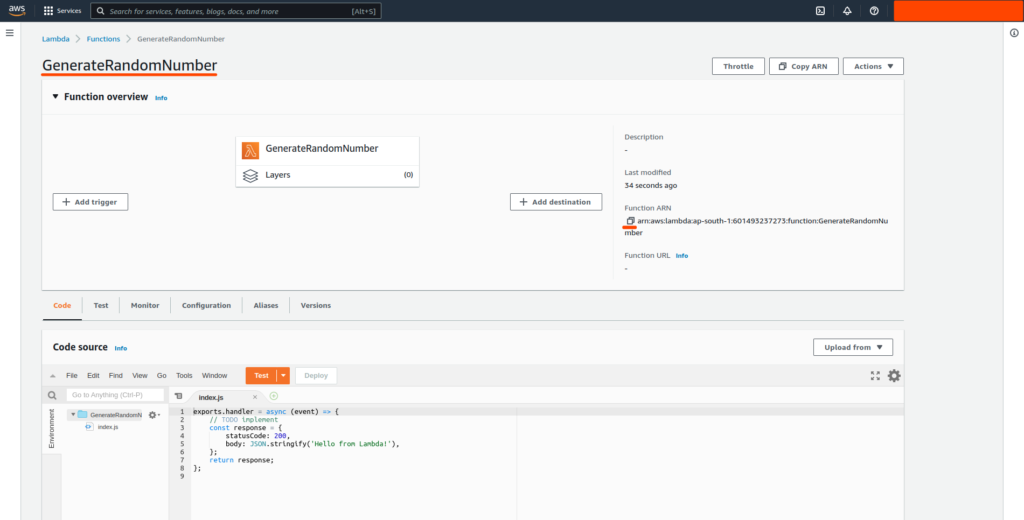
Now, you can edit the code according to your need, and deploy it as shown in the image below.
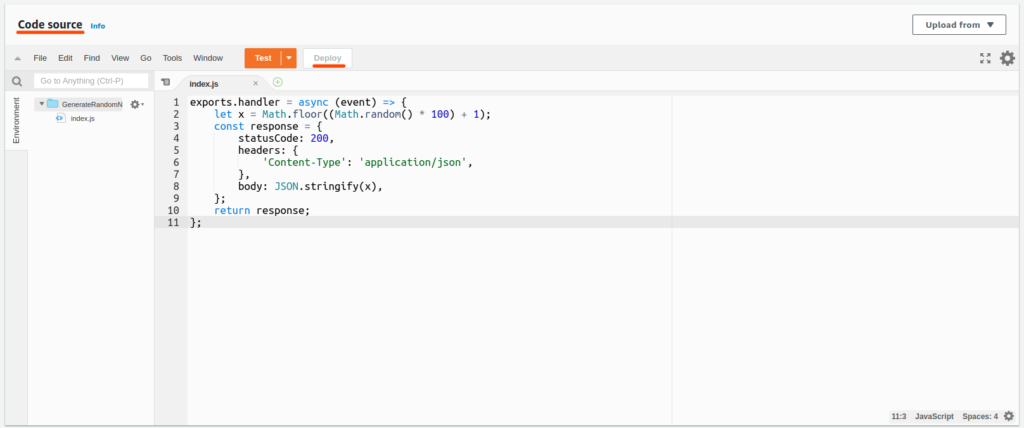
The code edited to generate a random number is.
Edited Code
//* GenerateRandomNumber lambda
exports.handler = async (event) => {
let x = Math.floor((Math.random() * 100) + 1);
const response = {
statusCode: 200,
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(x),
};
return response;
};
The above code will generate a random number between 1 to 100.
After Deploying the changes to the lambda, copy the Function ARN as shown in the image below.
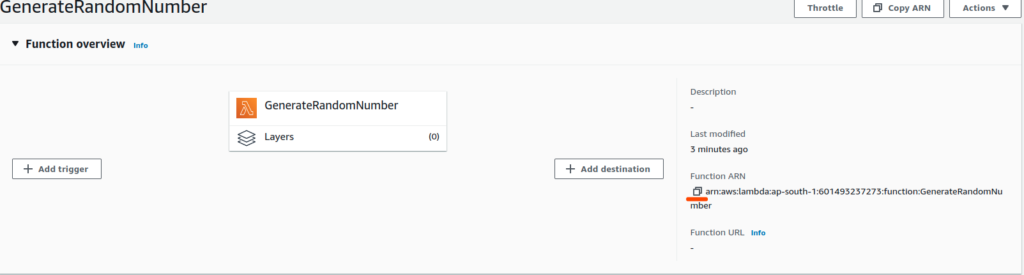
After copying the Function ARN paste it into the REST API we were creating as shown in the image below, and give permission if it asks for it.
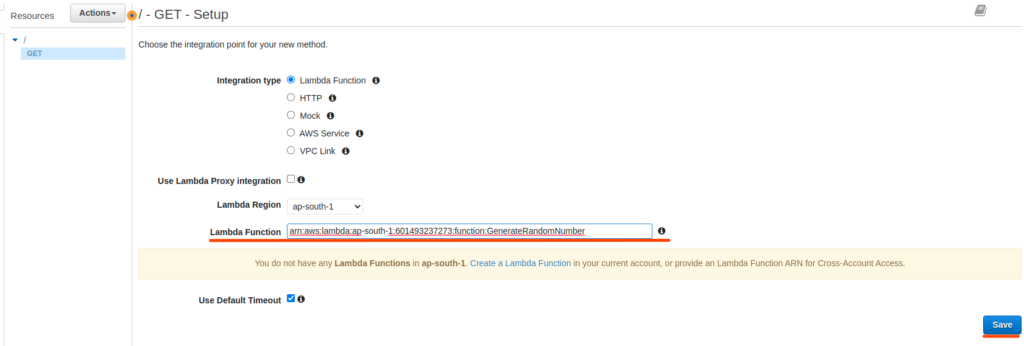

After giving permission for API Gateway to invoke the Lambda function, we can test and deploy the API.
At this point in the article, we are at the halfway point of achieving our goal of creating REST API in Node.js using AWS API Gateway.
Testing the connected Lambda
Before Deploying the function let’s test it. Click on the test button as shown in the image.
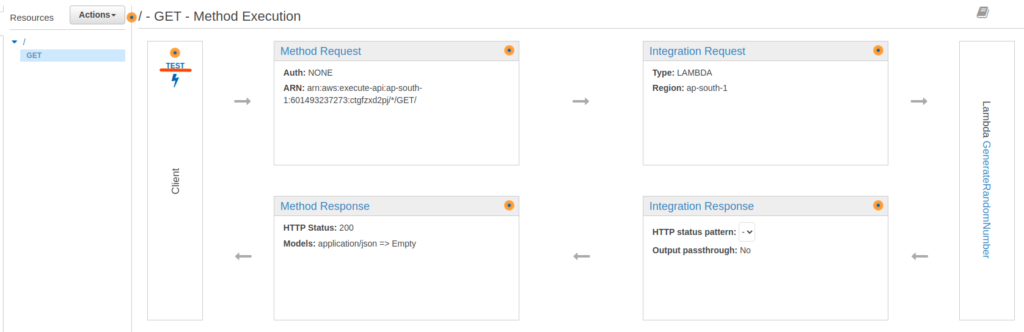
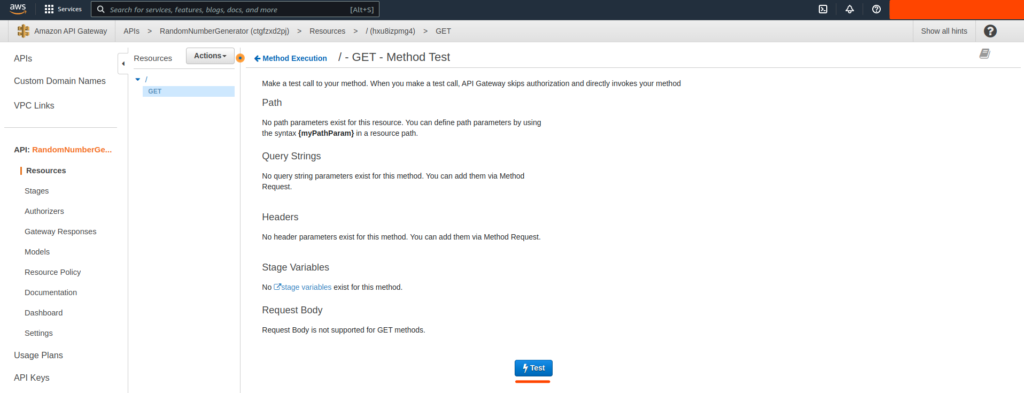
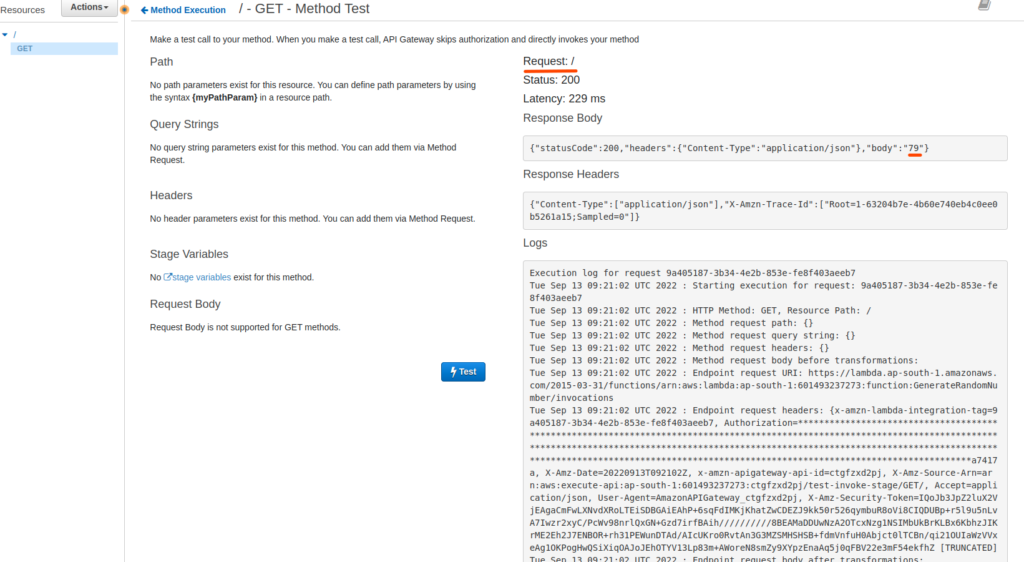
The result is back in the console. It shows the response body, response header, and logs the function has generated.
Deploying the lambda
For the API to run it need to be deployed, it is done after API is tested, and the deployment is done by going into actions and clicking on the Deploy API
option.
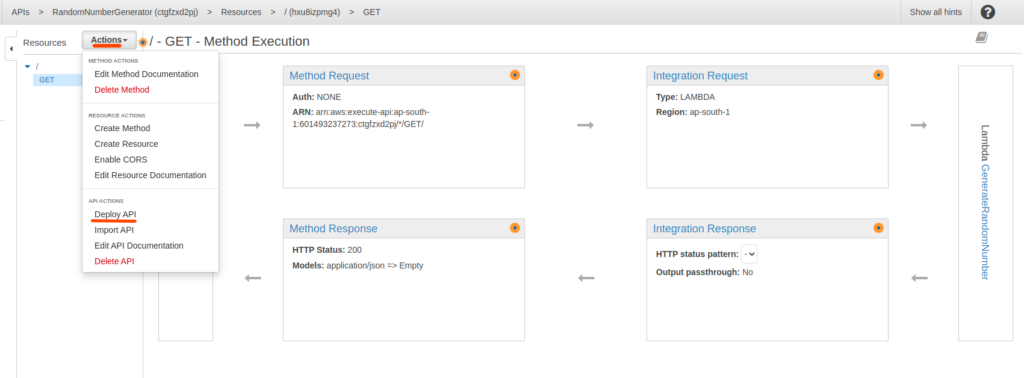
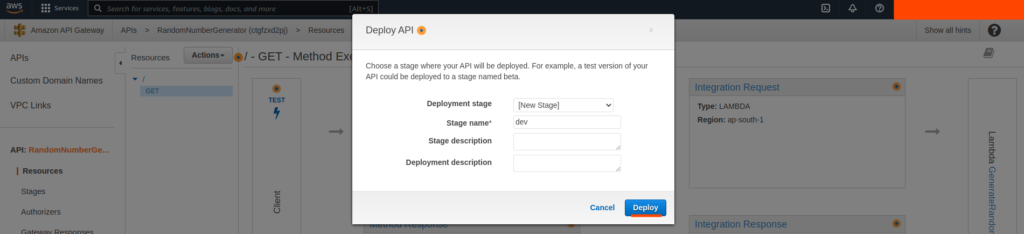
The API requires a stage name, and after deploying to a stage, an invoke URL is generated. This URL later handles the requests.
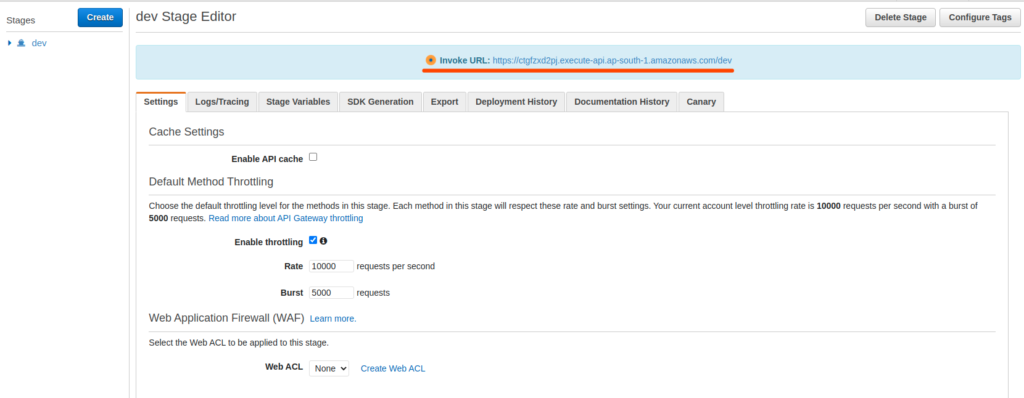
After the deployment of API is complete. This is the invoke URL, which when requested will invoke the GenerateRandomNumber lambda and return the response of the invoked lambda.
Invoking the API Gateway URL
Let’s copy the URL and invoke it through a web browser, or through Postman.

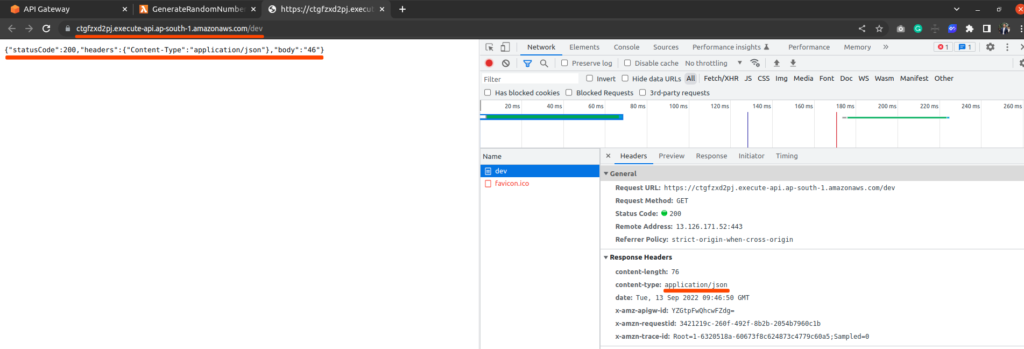
The results are returned in the form of a JSON object, we can confirm it through the return content-type
. We have succeeded in creating a REST API in node.js using AWS API Gateway.
HTTP Methods in Lambda
A single REST API can have multiple endpoints which are made through the API Gateway. This is shown in the images down below.
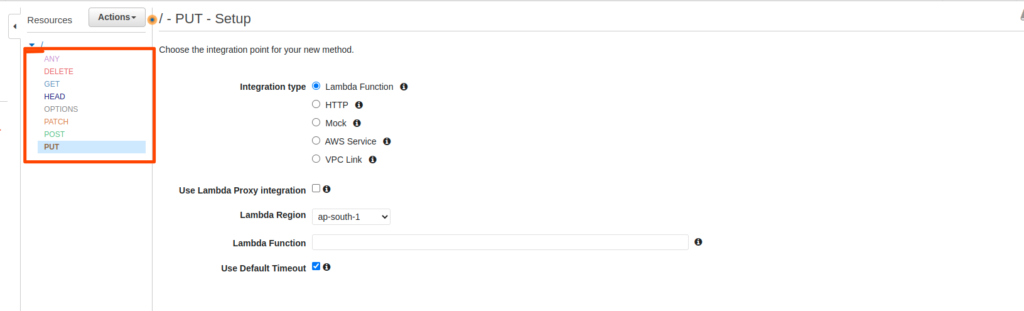
The Methods that can be present inside are:
- ANY
- GET
- POST
- PUT
- DELETE
- HEAD
- OPTIONS
- PATCH
Each of these methods can have a lambda attached to it, making one REST API able to contain multiple functionalities.
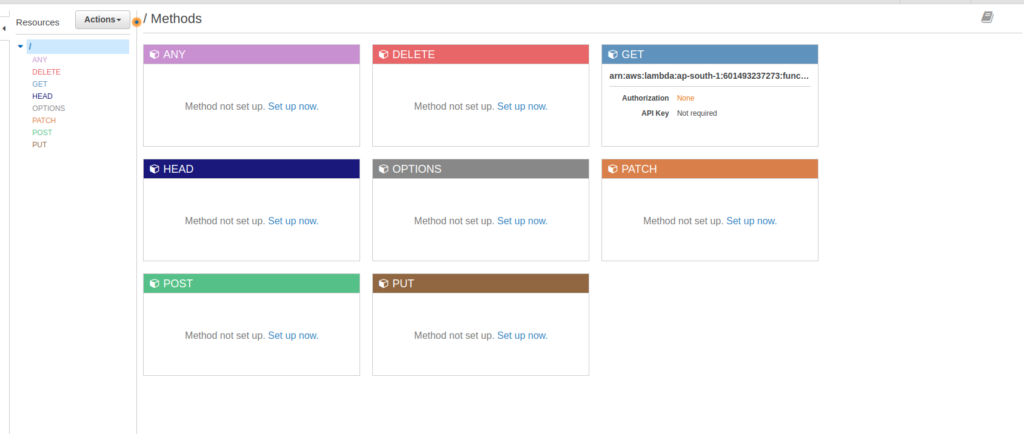
The above shows the Methods that can be present inside a single REST API endpoint. So, that’s how you create REST API in Node.js using AWS API Gateway.
Furthermore, we can look at the logs of the function in the CloudWatch service, so let’s look at that.
Cloudwatch
The CloudWatch keeps the logs of all the Lambda invocations. It is put in a group with the name GenerateRandomNumber which is the same as the created lambdas name.
When the log group is selected it shows the number of logs that the lambda has been triggered.
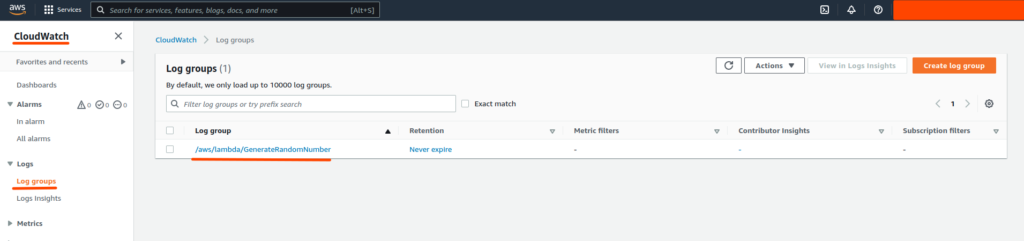
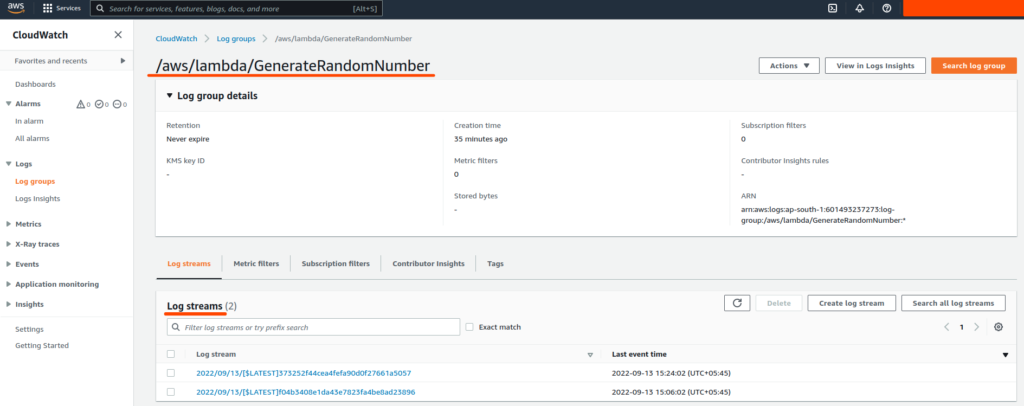
Let’s select a specific log in the log stream.
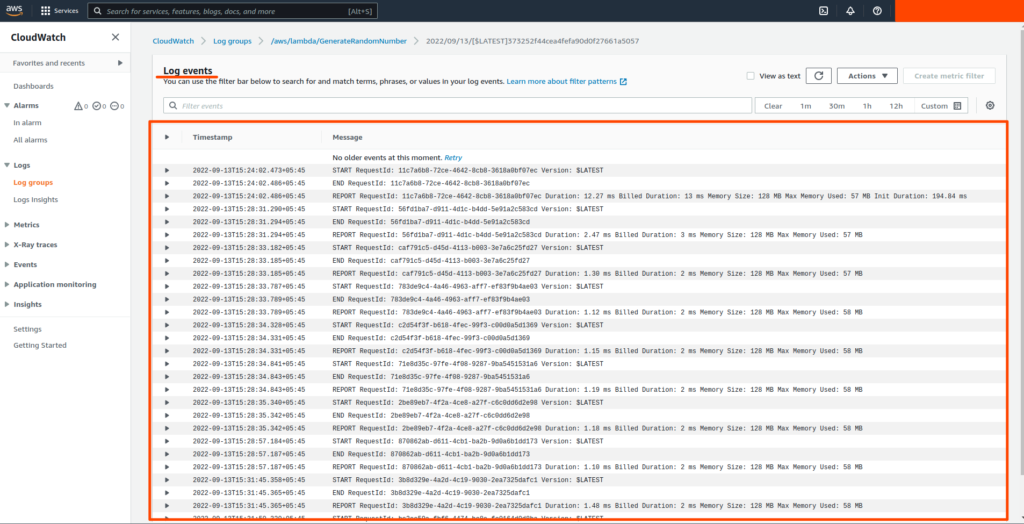
Finally, the log shows the start of the lambda and its RequestId, end the end of the request with initialization duration, billed duration, and memory consumed.
Conclusion
So, in this article, we learned, how to create a REST API in Node.js using AWS API Gateway through the AWS console.
Learn about creating a simple REST API in Lambda Here
.