Let’s learn how to create a simple rest API in Node.js using AWS Lambda.
Introduction
As the world is moving towards serverless computing, AWS Lambda is Amazon Web Services offering to the general masses, that provides an event-driven compute service that lets you run your code/ backend service without the hassle of managing servers. There are multiple framework offerings provided by different companies and cloud providers some include:
- AWS Amplify
- Zappa
- Architect
- Claudia.js
- AWS Lambda
Major benefits of using AWS lambda:
- It can be triggered by more than 200 AWS services
- It can scale from 1 to 2 events per day to more than thousands of events per second
- It is cost-effective as you only pay for the compute time used by your code
- It runs concurrently
- It has low latency
So, let’s look at creating an API using Lambda. Though there is a better alternative to this, which is API Gateway, for this article, let’s focus on creating REST API in Node.js using AWS Lambda.
Prerequisites
Generating a Function
To generate a function search for lambda in the AWS dashboard search section.

Click on Lambda and go to the lambda dashboard.

Click on the Create function to create a lambda function, then select Node.js. Choose the Use a blueprint option as shown below.
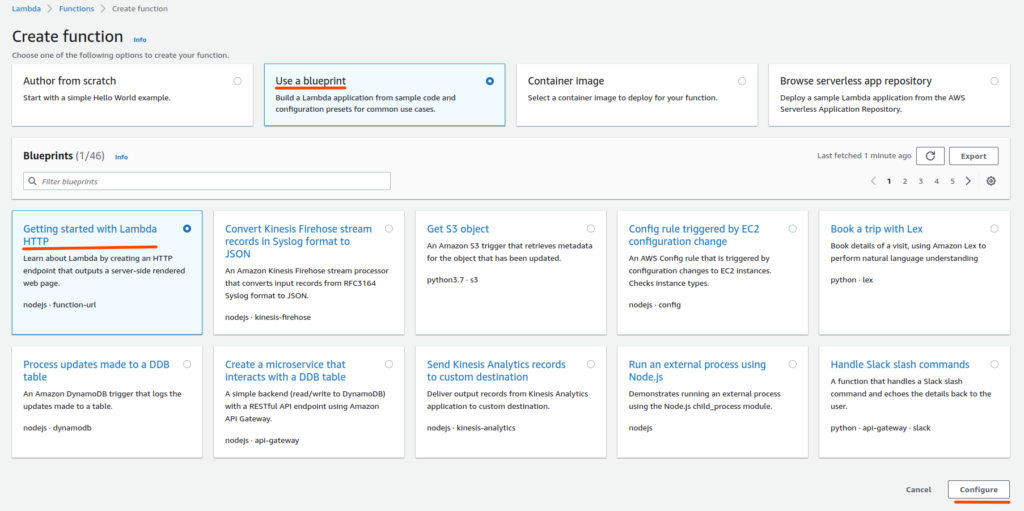
In the blueprints, section select the option “Getting started with Lambda HTTP” option, then click on configure. Then comes the page where you set up the function.
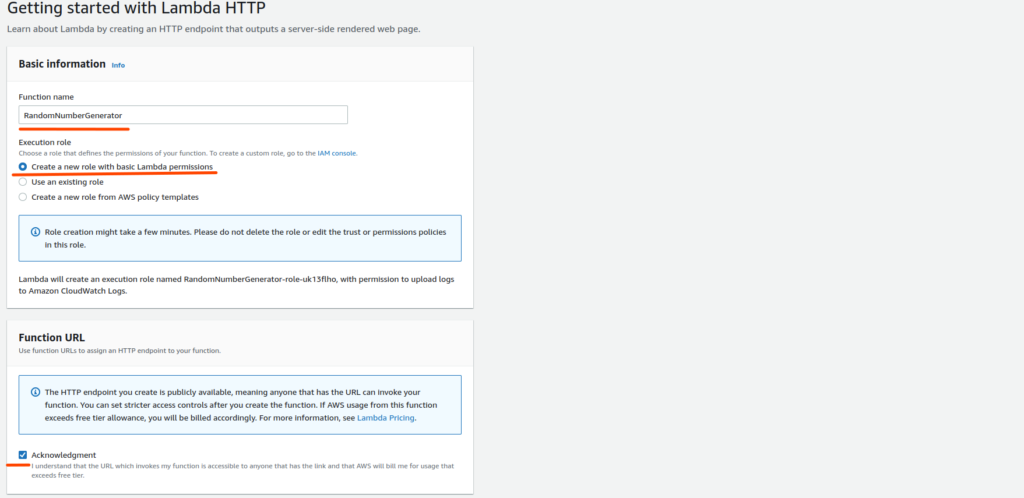
As shown in the above image give a name to the function, and select “Create a new role with basic Lambda permissions”. Then in the Function URL section select the Acknowledgement after reading the given prompt below the Acknowledgement section.
Then scroll to the bottom of the page and click on the “Create Function” button.
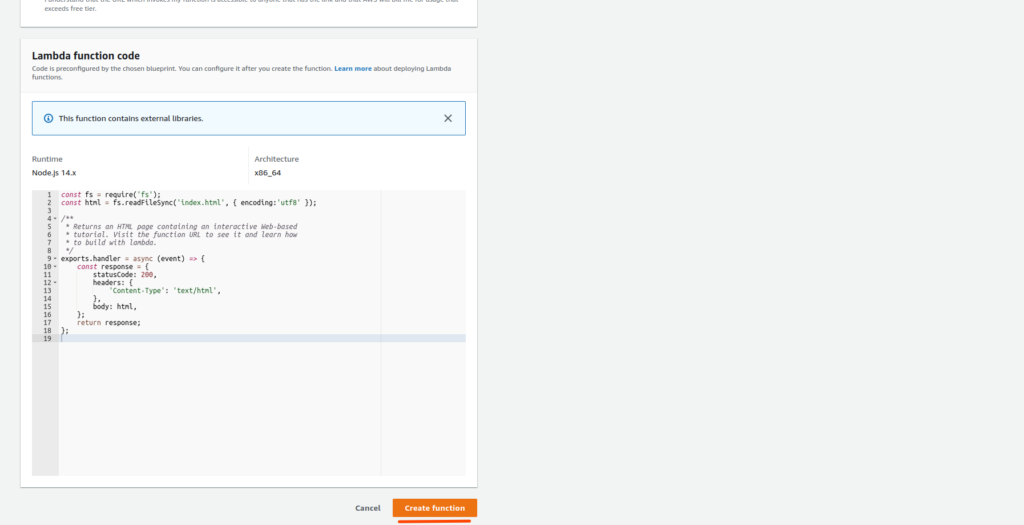
After the function is created, you are routed to the generated function page.
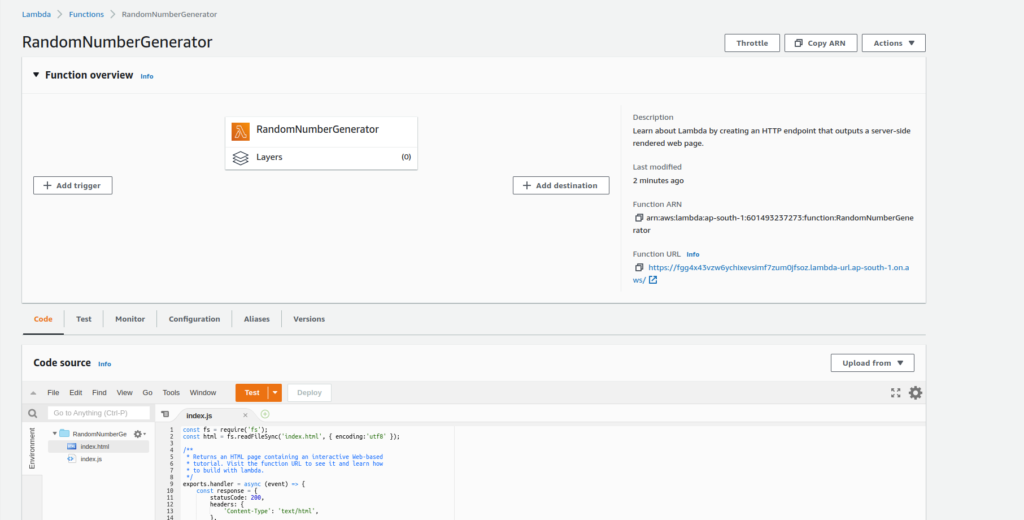
Editing the Lambda
The generated Lambda function can be edited now, so let’s edit and create a function that generates a random number between 1 to 100.
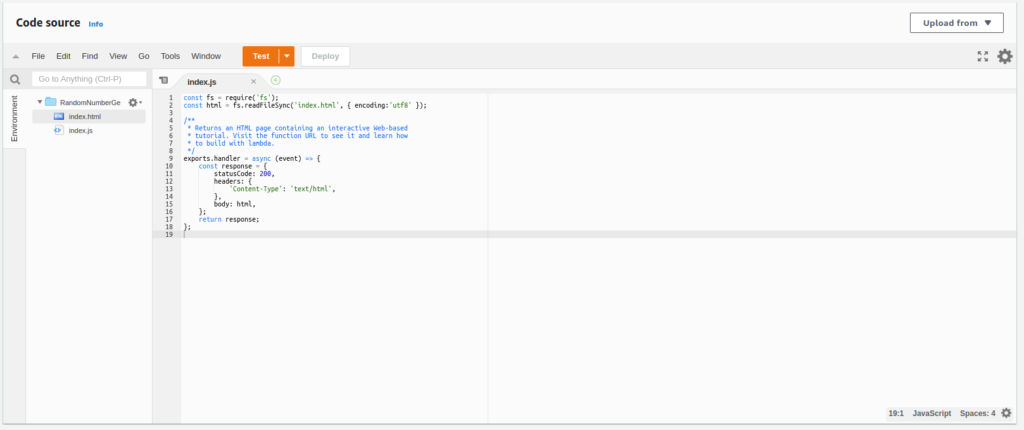
Lambda to generate a random number
//* RandomNumberGenerator lambda
exports.handler = async (event) => {
let x = Math.floor((Math.random() * 100) + 1);
const response = {
statusCode: 200,
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(x),
};
return response;
};
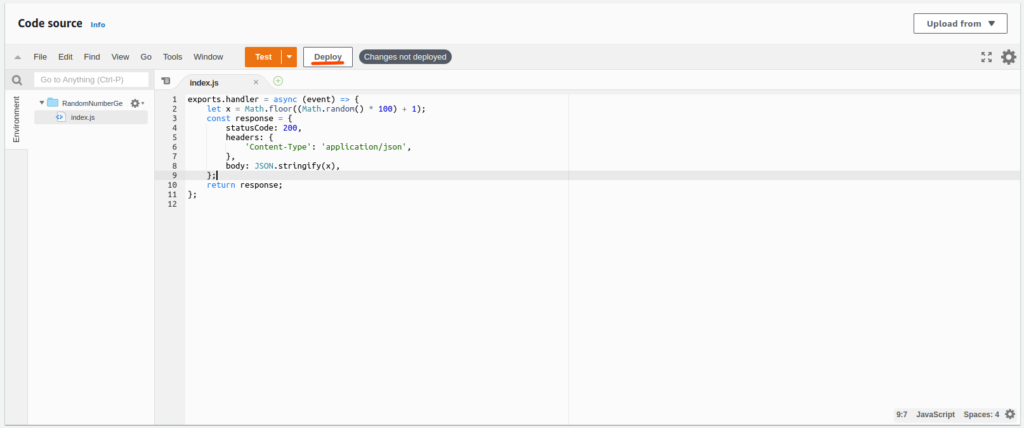
After editing the code deploy the changes.
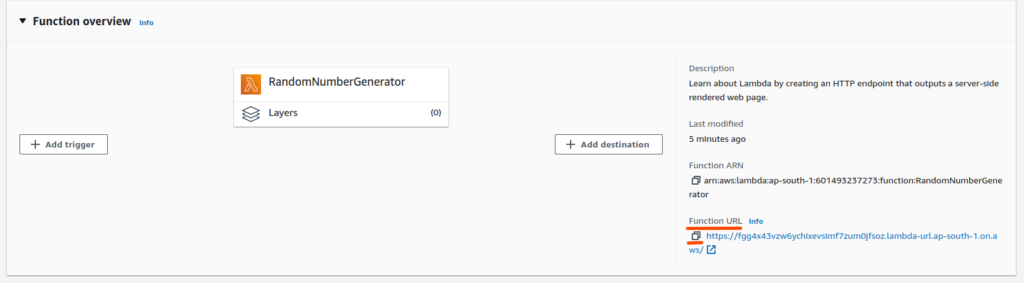
To trigger the lambda copy the function URL as shown in the above image. Trigger the API through a web browser or from the postman.

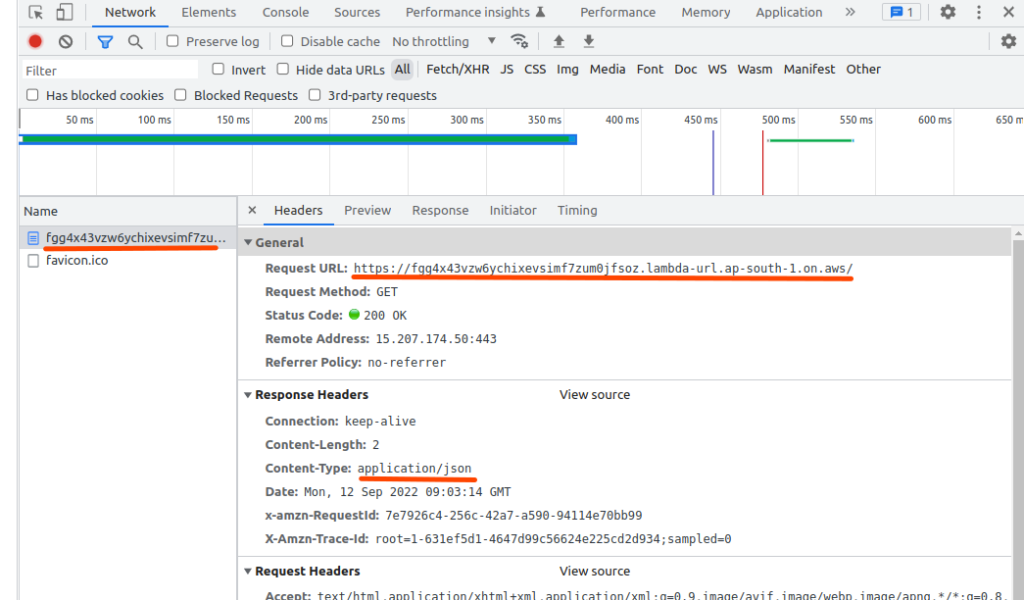
The above shows the response received after triggering the API. So, that’s how you REST API in Node.js using AWS Lambda.
Furthermore, we can look at the logs of the function in CloudWatch service, so let’s look at that.
Cloudwatch
The CloudWatch keeps the logs of all the Lambda invocations. It is put in a group with the name RandomNumberGenerator which is the same as the created lambdas name.
When the log group is selected it shows the number of logs that the lambda has been triggered.

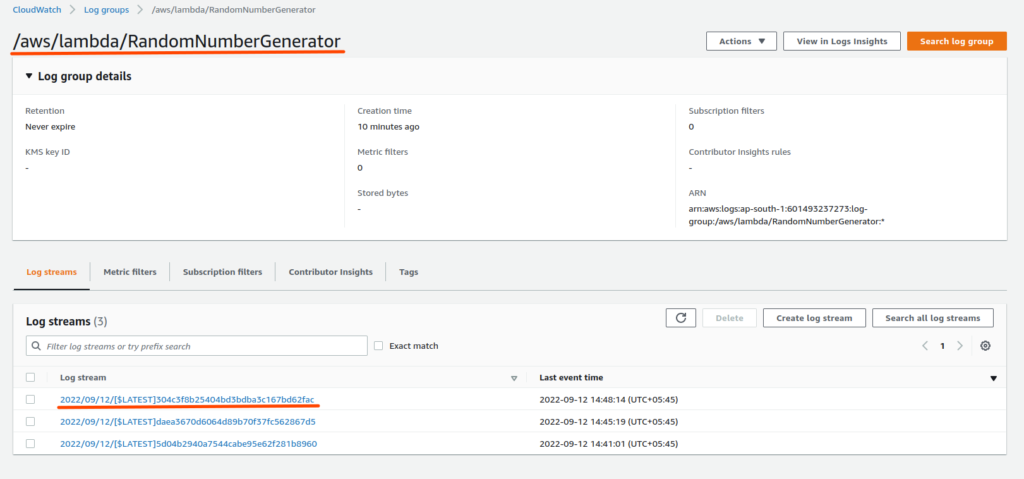
Let’s select a specific log in the log group.
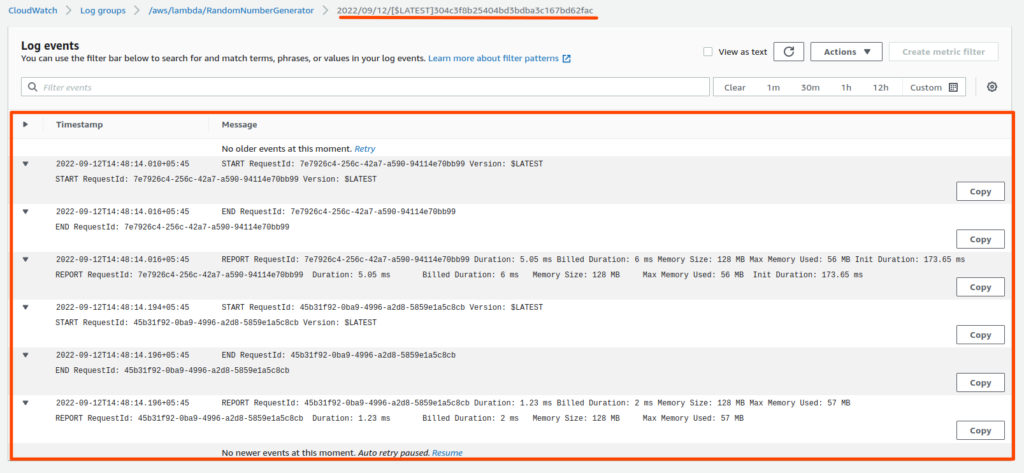
The log shows the start of the lambda and its RequestId, end the end of the request with billed duration, memory consumed, and initialization duration.
Conclusion
So, in this article, we learned, how to create a REST API in Node.js using AWS Lambda through the AWS console.