In this article, we will learn about the top 10 array functions in javascript. In JavaScript, an array is an ordered list of values. Each value is called an element specified by an index: A JavaScript array has the following characteristics: First, an array can hold values of mixed types. For example, you can have an array that stores elements with the types number, string, boolean, and null.
List of top 10 array functions in javascript
Here is the list of 10 array functions in javascript that are frequently used.
Filter
A filter()
method creates a new array from a given array that consists of only elements that meet the argument method’s condition.
Syntax,
array.filter(callback(element, index, arr), thisValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
const arr = [1, 2, 3, 4, 5, 6];
// item(s) greater than 3
const filtered = arr.filter(num => num > 3);
console.log(filtered); // output: [4, 5, 6]
console.log(arr); // output: [1, 2, 3, 4, 5, 6]
Some
A some()
method checks whether at least one element in an array meets the condition checked by the argument. A true value is returned if the function provided for an element in the array returns true; a false value is returned otherwise. It doesn’t modify the array.
Syntax,
array.some(callback(element, index, arr), thisArg)
Here,
callback
: This is the function to be called for each element of the array which is a required parameter.
element
: This parameter is required which consists of the current element of the array.
index
: This is an optional parameter that consists of the index of the current element of the array.
arr
: This is also an optional parameter that consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
const array = [1, 10, 15, 6 ];
// checks whether an element is less than 5
const fun = (element) => element < 5 ;
console.log(array.some(fun));
// expected output: true
Every
In the every()
method, all elements in an array are tested to see if they pass the condition available inside the provided callback function. It returns a true if all the elements pass the condition else returns false.
Syntax,
array.every(calback(element, index, arr), thisValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
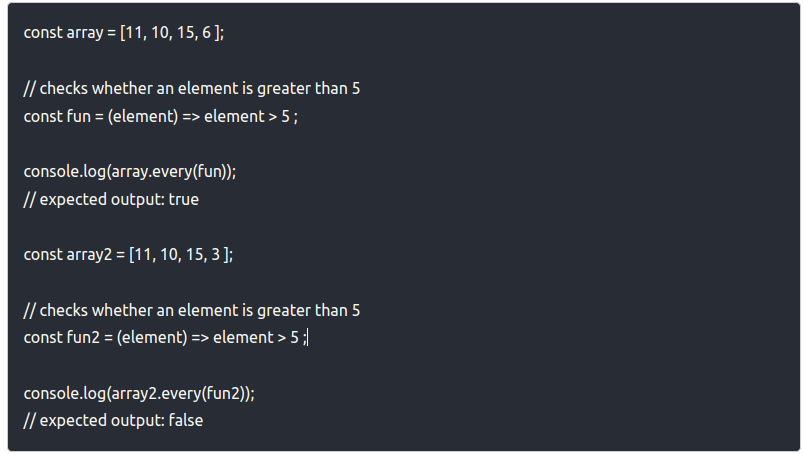
forEach
The forEach()
method is one of the javascript looping methods which is used to loop through all types of arrays. It executes a provided function once for each array element in ascending index order.
Syntax,
array.forEach(callback(element, index, arr), thisValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
const array = [1, 2, 3, 4, 5 ];
array.forEach(function(number) {
console.log(number);
});
//expected output:
1
2
3
4
5
Includes
An array’s includes()
method returns true or false based on whether a certain value is included in its entries.
Syntax,
array.includes(element, start)
Here,
element
: This is a required parameter that holds the value to be searched.
start
: This is an optional parameter that determines the start position to search the value. By default, its value is set to 0.
Example,
const array = [1, 2, 3];
console.log(array.includes(2));
// expected output: true
const pets = ['cat', 'dog', 'bat'];
console.log(pets.includes('rat'));
// expected output: false
Map
With map()
, each element of an array is converted into an array containing the results of the provided function.
Syntax,
array.map(callback(currentValue, index, arr), thisValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
const arr = [1, 2, 3, 4, 5, 6];
// add one to every element
const oneAdded = arr.map(num => num + 1);
console.log(oneAdded); // output [2, 3, 4, 5, 6, 7]
Reduce
JavaScript’s reduce()
method reduces the array to a single value, executes a function for each value, and stores the result in an accumulator.
Syntax,
array.reduce(callback(total, element, index, arr), initialValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
total
: This parameter is optional and consists initialValue or the previously returned value of the function.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and holds a value to be used inside the callback function.
Example,
const arr = []
function sum(arr) {
const reducer = (sum, val) => sum + val;
const initialValue = 0;
return arr.reduce(reducer, initialValue);
}
console.log(sum(arr));
// expected output: 16
Sort
The sort()
method sorts elements of an array in place and returns a reference to the sorted array. By default, it sorts the array in ascending order.
Syntax,
array.sort(function (a,b));
Here,
callback
: This is an optional parameter that defines the sort order.
a
: The first element for comparison.
b
: The second element for comparison.
Example,
const numbers = [100, 1, 30, 4, 21];
numbers.sort((a, b) => a - b);
console.log(numbers);
// expected output: [ 1, 4, 21, 30, 100 ]
Find
A find()
method returns the first element in an array that satisfies the provided testing function. A value that does not satisfy the testing function is returned as undefined.
Syntax,
array.find(callback(element, index, arr),thisValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
const array = [5, 12, 8, 130, 44];
const result = array.find(element => element > 7);
console.log(result);
// expected output: 8
Find index
The findIndex()
methods return the index of the first element that satisfies the condition inside the callback function. The method returns -1 if no elements satisfy the condition.
Syntax,
array.findIndex(callback(element, index, arr), thisValue)
Here,
callback
: This is the function to be called for each element of the array. This parameter is required.
element
: This parameter is required which consists of the current element of the array.
index
: This parameter is optional and consists of the index of the current element of the array.
arr
: This parameter is optional and consists array on which the filter method is called.
thisValue
: This parameter is optional and has a default value undefined
. This parameter holds a value to be used inside the callback function.
Example,
const array = [5, 12, 8, 130, 44];
const result = array.findIndex(element => element > 7);
console.log(result);
// expected output: 1
Conclusion
In this article, you have learned the top 10 array functions in javascript. You can follow this link to learn more about javascript loops.