Prerequisites
- Basic Understanding of JavaScript You can learn JavaScript from the JavaScript Tutorial.
- Node.js installed locally
Introduction
TypeScript is a superset of TypeScript is a strongly typed, object oriented, compiled language. It was designed by Anders Hejlsberg (designer of C#) at Microsoft. TypeScript is both a language and a set of tools. TypeScript is a typed superset of JavaScript compiled to JavaScript. In other words, TypeScript is JavaScript plus some additional features. It’s purpose is to add strict static typing to JavaScript, so as to ensure type correctness, and eliminate bugs that stem from type mismatches.Typescript files have a .ts
extension.
Why TypeScript?
- TypeScript is fast, simple, easy to learn
- TypeScript supports JS libraries & API Documentation
- It is similar to JavaScript, and it uses the same syntax and semantics.
- TypeScript works with existing JavaScript frameworks and libraries without any problem.
- TypeScript Code can be converted into plain JavaScript Code
- Better code structuring and object-oriented programming techniques
- It supports Object Oriented Programming concepts like classes, interfaces, inheritance, etc.
To get started with TypeScript, We’ll need the following tools:
Node
Node.js is the environment in which the TypeScript compiler runs.
Download and install node js from this link
After the node js is installed in our operation systems.Enter the command below in terminal to check the node version
node -v
TypeScript compiler
It is a Node.js module which will compiles TypeScript into JavaScript
We need to install TypeScript by using command below in our terminal
npm install -g typescript
after the installed ,We can check its version by using the command below
tsc -v
It will return the version like this
Version 4.6.4
Text Editor
We can use any text editor like notepad +,sublime,vim,visual studio etc.
I will use Visual Studio as my preferred text editor
Download and install visual studio in your local machine frome here.
Example
Let’s create new folder with the name typescript-example and create app.ts file
Open the folder in code editor and add the code below in app.ts file
let subject: string = 'Typescript example';
console.log(subject);
Here we have decleare the variable subject which is string type.
Not let’s compile this app.ts file to javascript by using command below in project directory.
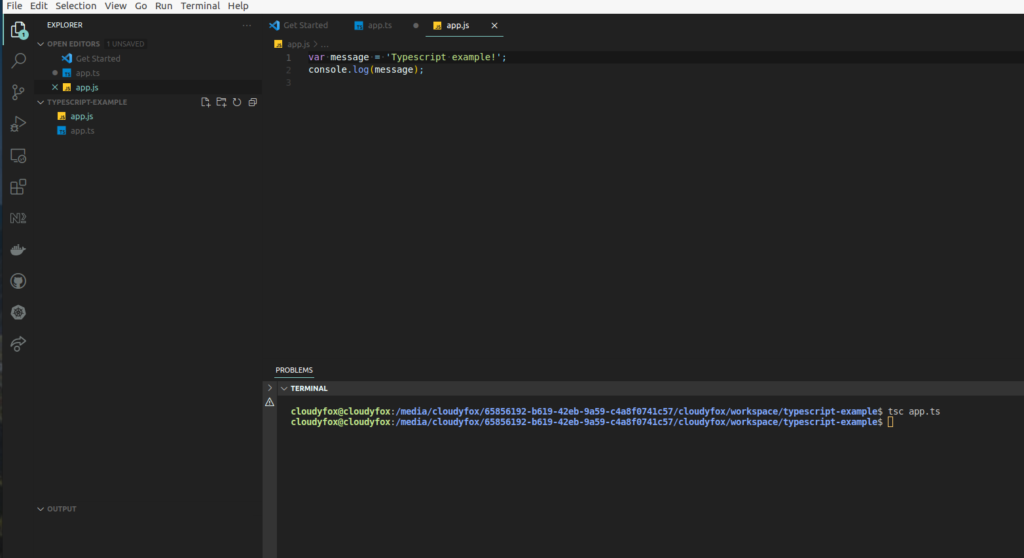
Here we can see the new file app.js has been generated by the TypeScript compiler.
We can run the app.js file in node.js using the following command:
node app.js
Result below will be displayed in the terminal

Run TypeScript Example in Web Browsers
We will run the typescript-example project in our web browsers.First we need to create the index.html
file and add the code below in the that file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>TypeScript Example</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
Here we have create the simple html file where we have import our app.js
file
Update code in app.ts
file with code below.
let subject: string = 'Typescript example!';
// create a new paragraph element
let paragraph = document.createElement('p');
paragraph.textContent = subject;
// add the paragraph to document
document.body.appendChild(paragraph);
console.log(subject);
Here,We have created the paragraph tag and added the text content from our subject variable .
Then,we have append that paragraph tag
Compile app.ts
file using command below.
tsc app.ts
We can see the changes made in app.js file after compiling
var subject = 'Typescript example!';
// create a new paragraph element
var paragraph = document.createElement('p');
paragraph.textContent = subject;
// add the paragraph to document
document.body.appendChild(paragraph);
console.log(subject);
Now let’s open the index.html file in our browser.We will see paragraph has been added in index.html file.
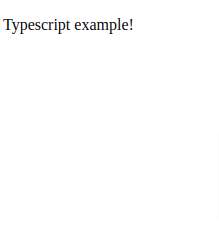