In this tutorial, we will see how to send emails from a react app using EmailJS without a server/backend. EmailJS is an email service that helps to send emails using client-side technologies only. No server is required. We just need to connect EmailJS to one of the supported email services, create an email template, and use our Javascript library to trigger an email.
Now let’s begin the steps to send emails from a react app using EmailJS.
Step 1: Create an account in EmailJS
You can create an account in EmailJS through its sign-up page.
Step 2: Add Preferred Email Service
After the sign-in/sign-up step, you will be redirected to the dashboard. Now, you need to add an email service of your choice. You can add an email service through the Email Services tab and then click on the Add New Service as shown in the screenshot below.
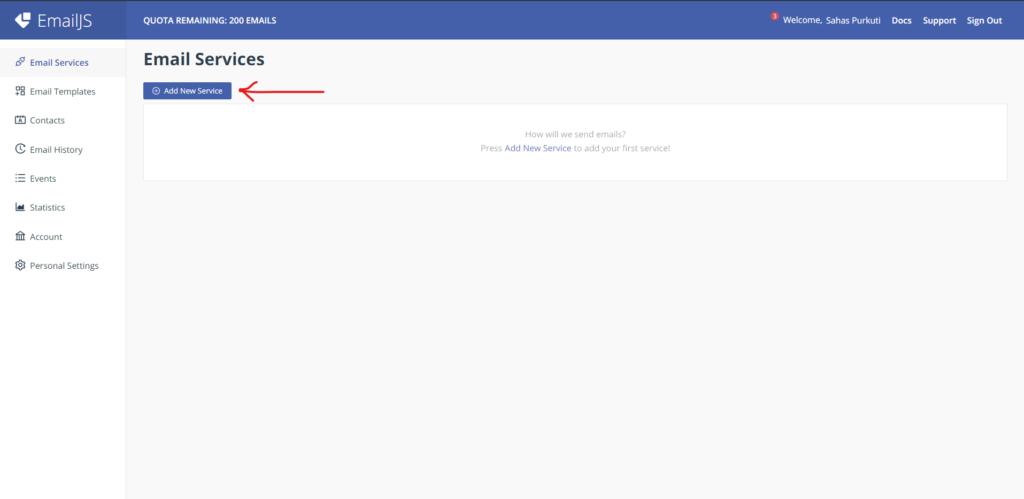
I will be choosing the Gmail service. Then, You need to connect to an email account where the emails will be sent.
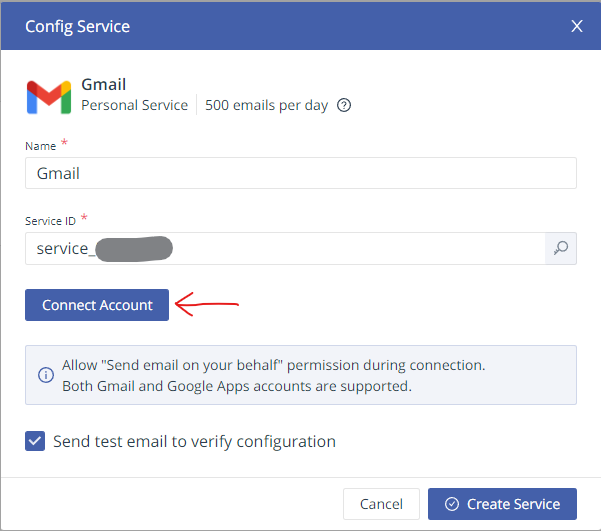
After connecting the account, click on the Create Service button.
Email service is created and connected and can be seen on the Email Services Tab.
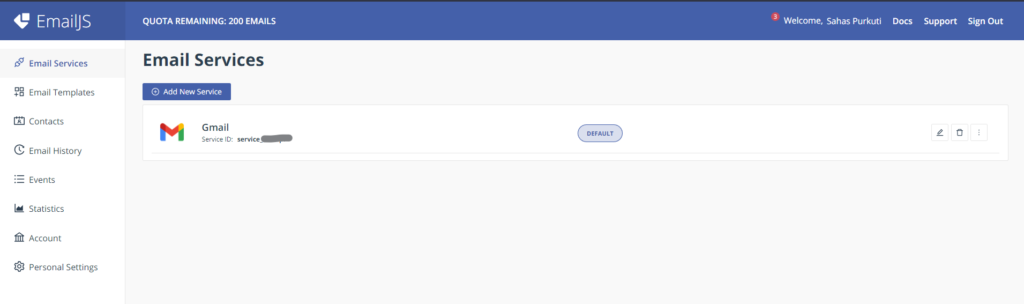
Step 3: Create an Email Template
Email templates can be created through the Email Templates tab. Then, Click on the Create New Template button.
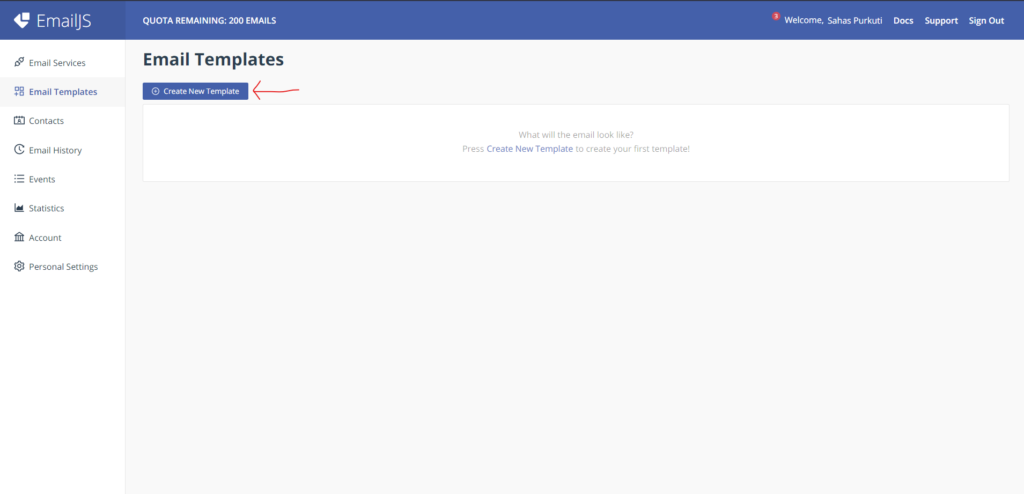
Here, On the Content tab, you can create your desired template for receiving an email.
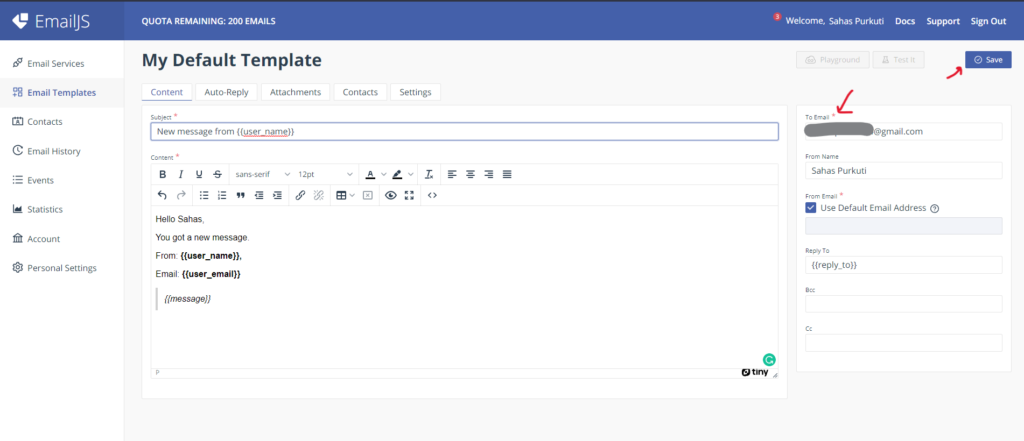
The Content tab of the Email Templates offers us to edit the subject of email in Subject field, content of the email in Content field, and receiver Email address in the To Email field. The values like {{user_name}}
and {{user_email}}
displays the dynamic texts that are provided by the user through the form. For this, the keys {{user_name}}
and {{user_email}}
should be created in the corresponding input fields in the form. Then, click on the Save button to save changes.
Sample Email Template:
Hello Admin, You got a new message. From: {{user_name}}, Email: {{user_email}}, {{message}}
The created templated are displayed in the Email Templates homepage.
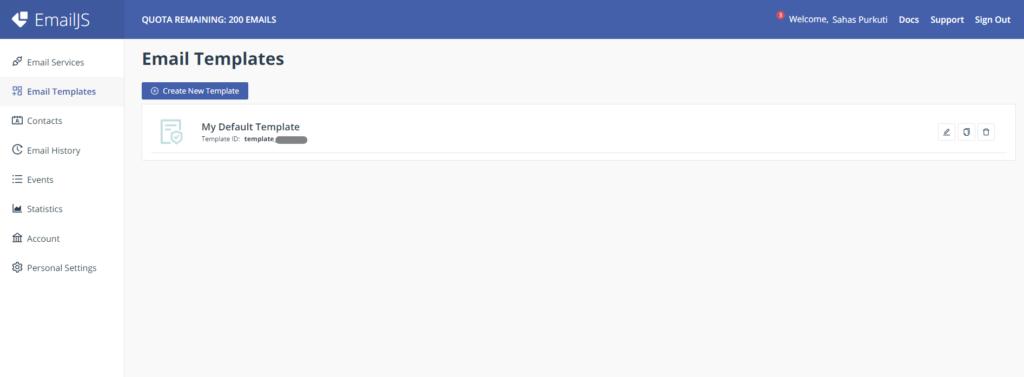
Step 4: Create Auto-Reply Email Template
This is an optional step. The auto-reply email template can be created through the Auto-Reply tab. You need to enable the Auto-Reply Form. The fields are similar to the Content tab but here the subject and content are visible in the user’s email. Then, click on the Save button to save changes.
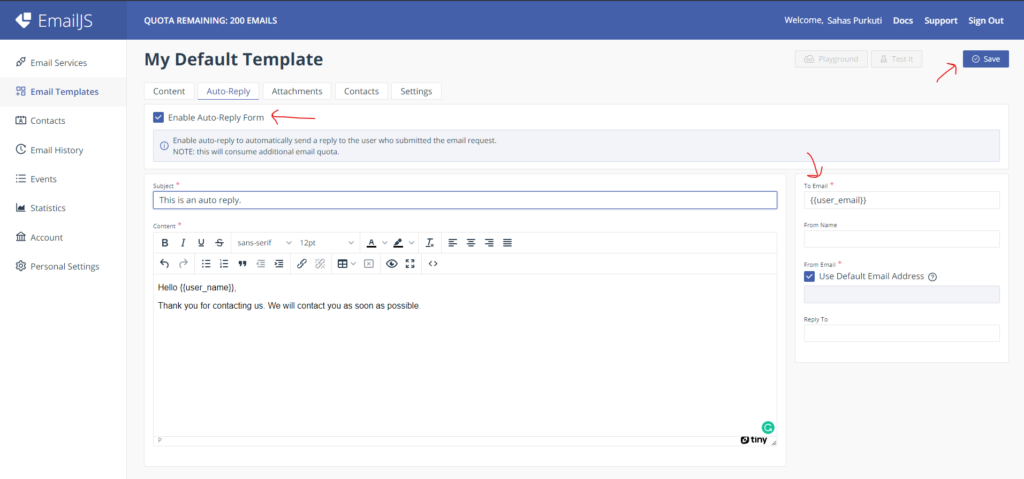
Sample Auto-Reply Email Template:
Hello {{user_name}}, Thank you for contacting us. We will contact you as soon as possible.
Step 5: Install EmailJS Package
Now, we need to set up our react application to send emails from a react app using EmailJS. So, We need to install the EmailJS package.
npm install @emailjs/browser
Step 6: Import emailjs and Create a Form
import React, { useRef } from 'react';
import emailjs from '@emailjs/browser';
export default function ContactUs(){
const form = useRef();
const sendEmail = (e) => {
e.preventDefault();
emailjs.sendForm('YOUR_SERVICE_ID', 'YOUR_TEMPLATE_ID', form.current, 'YOUR_PUBLIC_KEY')
.then((result) => {
alert("message sent succesfully");
}, (error) => {
alert("failed to send message");
});
};
return (
<form ref={form} onSubmit={sendEmail}>
<label>Name</label>
<input type="text" name="user_name" />
<label>Email</label>
<input type="email" name="user_email" />
<label>Message</label>
<textarea name="message" />
<input type="submit" value="Send" />
</form>
);
};
emailjs provides a sendForm()
function. It accepts SERVICE_ID
, TEMPLATE_ID
, form and PUBLIC_KEY
. The SERVICE_ID can be found on the Email Services page, TEMPLATE_ID
can be found on the Email Templates page, and PUBLIC_KEY
can be found on the Account page in the API keys section. The form element is selected using the useRef()
hooks.
Output
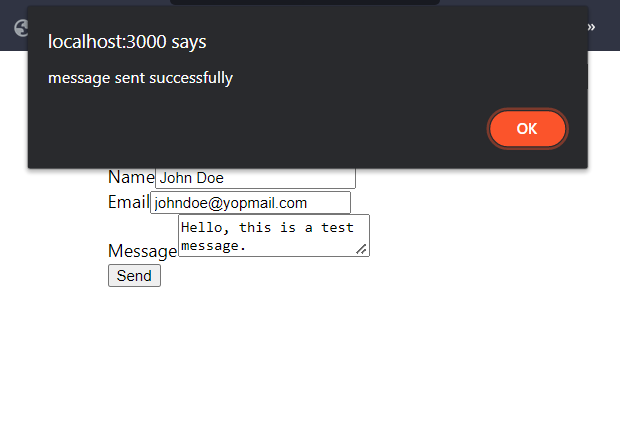
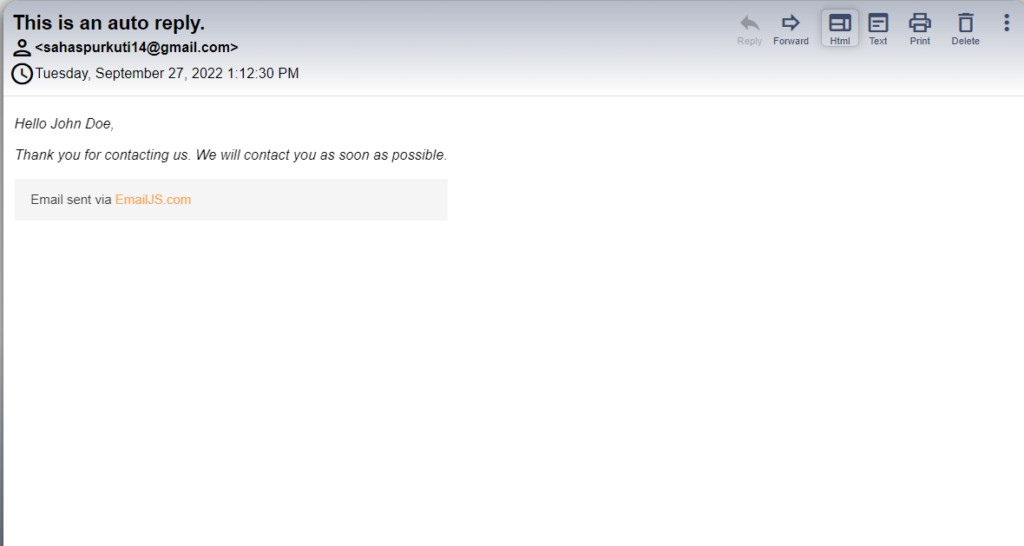
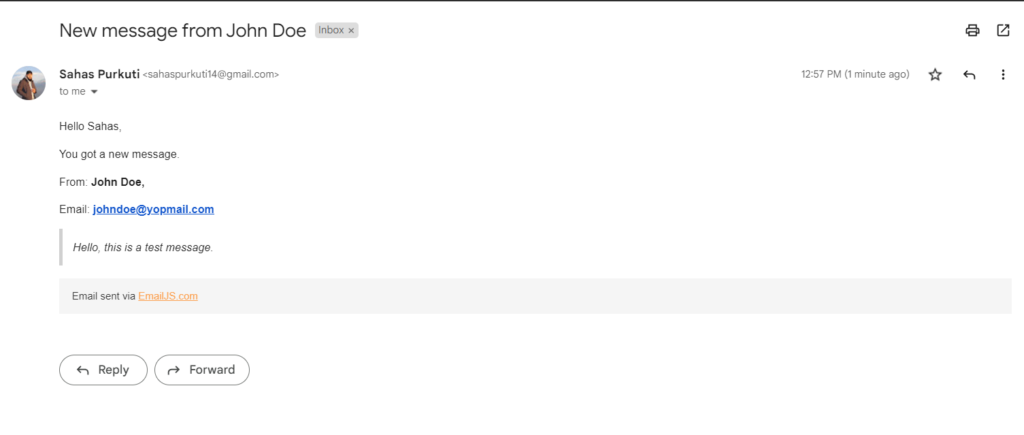
Conclusion
In this tutorial, we discussed how to send emails from a react app using EmailJS. We sent an email from the react app contact form to the admin using EmailJS without any backend or server.