In the previous article, we discussed the concepts of the react-router v6. In this article, we will discuss about the nested route and dynamic route in react. We will create a simple react app to understand how to create a nested route and also understand the concept of dynamic route and understand how to create dynamic routes and access the URL parameters using useParams.
What is Nested Route?
Nested route means routing within routes. For example: Lets say we have a page with list of products in the route /products
. When click on the item of a list say product 1 , we need to route to next page, /products
being the parent route. when I click on product 1 we need to route to products/product1
. Lets understand it more clearly with a tutorial of create a nested route.
Step 1: Create a react-app and run it
npx create-react-app tutorial-react-router
npm start
Step 2: Install react-router-dom
npm install react-router-dom@v6
Step 3: Create a new folder named pages inside src directory. And create new files under pages. For this tutorial, i will be creating 5 new files for Home, Products, Product1, Product2 and Product3.
The folder structure look something like this:
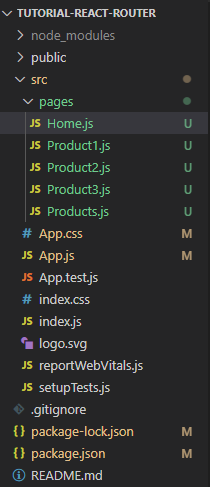
Step 4: Edit the files inside pages folder.
Home.js
import React from "react";
import { Link } from "react-router-dom";
function Home() {
return (
<div>
<h1>this is home page</h1>
<Link to="/products"> <h4>Go to Products page</h4></Link>
</div>
);
}
export default Home;
Products.js
import React from "react";
import { Link } from "react-router-dom";
function Products() {
return (
<div>
<h1>This is products page</h1>
<h3>Select a product to continue...</h3>
<ul>
<li><Link to="/products/product1">Product 1</Link></li>
<li> <Link to="/products/product2">Product 2</Link></li>
<li> <Link to="/products/product3">Product 3</Link></li>
</ul>
</div>
);
}
export default Products;
Product1.js
import React from "react";
function Product1() {
return (
<div>
<h1>This is a page for PRODUCT 1</h1>
</div>
);
}
export default Product1;
Product2.js
import React from "react";
function Product2() {
return (
<div>
<h1>This is a page for PRODUCT 2</h1>
</div>
);
}
export default Product2;
Product3.js
import React from "react";
function Product3() {
return (
<div>
<h1>This is a page for Product 3 </h1>
</div>
);
}
export default Product3;
Step 5: Import the pages in App.js and define routes for them.
import "./App.css";
import { BrowserRouter, Route, Routes } from "react-router-dom";
import Home from "./pages/Home";
import Products from './pages/Products'
import Product1 from "./pages/Product1";
import Product2 from "./pages/Product2";
import Product3 from "./pages/Product3";
function App() {
return (
<div className="App">
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/products" element={<Products />} />
<Route path="/products/product1" element={<Product1 />} />
<Route path="/products/product2" element={<Product2 />} />
<Route path="/products/product3" element={<Product3 />} />
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
The Output looks like :
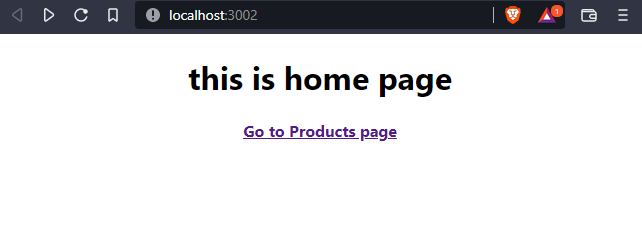
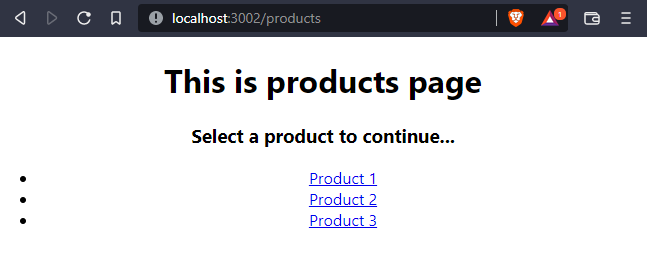
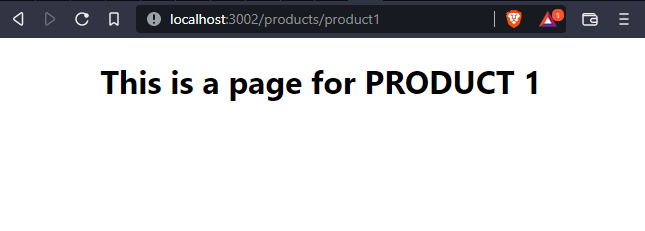
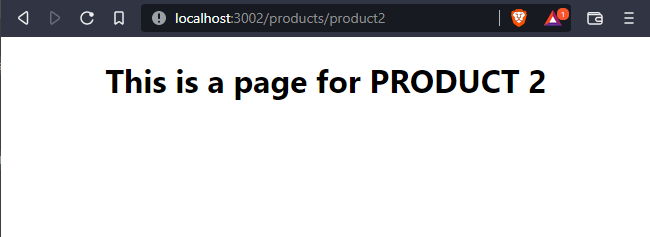
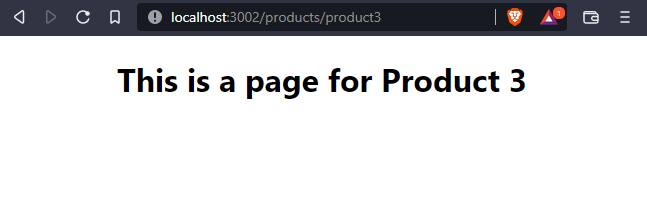
What is Dynamic Routes?
In the above tutorial, there was a limited products so it was possible to define routes for each of them. What if there are large number of products or something like that. Let’s take an example of an ecommerce site. There can be infinite number of products so it becomes impossible to create pages for each of them and define routes for every single products. This is where dynamic routes are used. In dynamic routing, we define dynamic paths and access those paths using useParams
to render the page conditionally. For example dynamic paths can be products/:id
. :id
represents all the id. And using the useParams
, we can access the provided id in the :id. Lets understand with some tutorial.
Step 1: Extending the app from the nested route above, Lets add more products on the products list. You can add as many as you want.
Products.js
import React from "react";
import { Link } from "react-router-dom";
function Products() {
return (
<div>
<h1>This is products page</h1>
<h3>Select a product to continue...</h3>
<ul>
<li><Link to="/products/product1">Product 1</Link></li>
<li> <Link to="/products/product2">Product 2</Link></li>
<li> <Link to="/products/product3">Product 3</Link></li>
<li> <Link to="/products/product4">Product 4</Link></li>
<li> <Link to="/products/product100">Product 100</Link></li>
<li> <Link to="/products/product1000">Product 1000</Link></li>
<li> <Link to="/products/product564654">Product 564654</Link></li>
</ul>
</div>
);
}
export default Products;
Step 2: Edit App .js
import "./App.css";
import { BrowserRouter, Route, Routes } from "react-router-dom";
import Home from "./pages/Home";
import Products from './pages/Products'
import Product1 from "./pages/Product1";
import Product2 from "./pages/Product2";
import Product3 from "./pages/Product3";
import DynamicPage from "./pages/DynamicPage";
function App() {
return (
<div className="App">
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/products" element={<Products />} />
<Route path="/products/product1" element={<Product1 />} />
<Route path="/products/product2" element={<Product2 />} />
<Route path="/products/product3" element={<Product3 />} />
<Route path="/products/:id" element={<DynamicPage/>} />
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
Here, :id
accepts any type of id and redirects the user to the DynamicPage.
Step 3: Create a page that will render dynamic contents using useParams
. useParams
helps to access the URL parameters.
DynamicPage.js
import React from 'react'
import { useParams } from 'react-router-dom'
function DynamicPage() {
const {id} = useParams();
return (
<div>
<h1>This is a dynamic page for {id} </h1></div>
)
}
export default DynamicPage
Output:
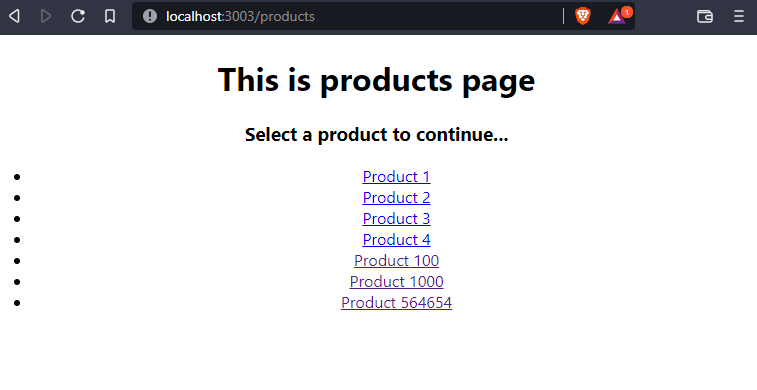
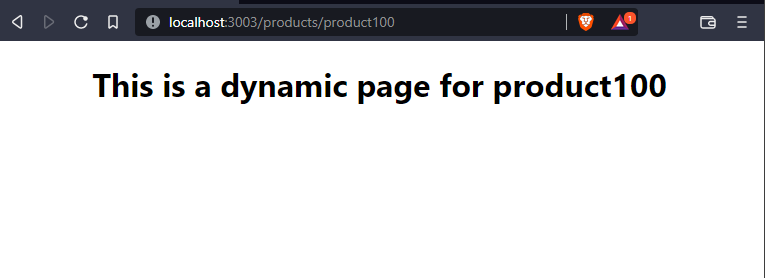
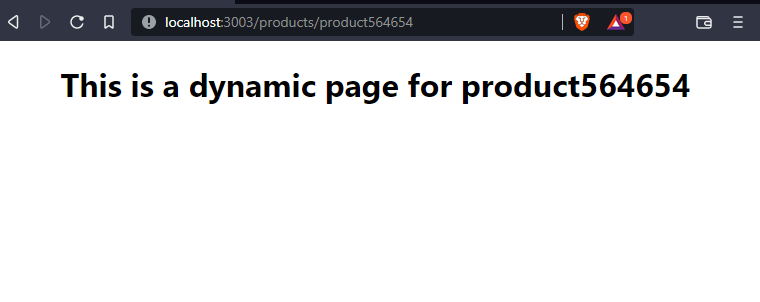
Conclusion
In this article, we discussed about the nested route and dynamic route in react. We learned how to create and use a nested route and dynamic route. We also mentioned the concept of useParams that helps in accessing the URL parameters and helps in rendering the dynamic components according to the path provided in the URL.