In this tutorial, we will be discussing form validation in React. Validation is the process of checking if the value given by the user is valid or not for the requested data. In the forms, we usually need to validate the name, email, password, phone, etc to ensure that the provided values are valid.
Prerequisites
- React form and
useState
hooks. - JavaScript operators and if else if statements.
Create a Sign-Up Form
We will create a simple sign-up form using bootstrap. So, we need to install bootstrap in the existing project then import it into the App.js file and create a form.
npm install bootstrap
import React from "react";
import "bootstrap/dist/css/bootstrap.min.css";
export default function App() {
const handleSubmit = () => {
alert("submitted");
};
return (
<form onSubmit={handleSubmit} className="col-10 m-4 p-2">
<h2>Sign Up Form</h2>
<div className="form-group mt-2">
<label>Name</label>
<input type="text" setEmail className="form-control" />
</div>
<div className="form-group mt-2">
<label>Email address</label>
<input type="text" className="form-control" />
</div>
<div className="form-group mt-2">
<label>Phone</label>
<input type="text" className="form-control"/>
</div>
<div className="form-group mt-2">
<label>Password</label>
<input type="password" className="form-control"/>
</div>
<input type="submit" value="Sign Up" className="mt-2 w-100 p-1" />
</form>
);
}
Output
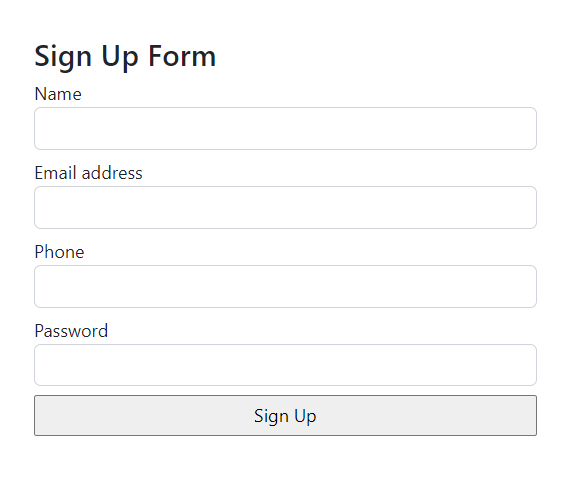
Implementing Form Validation in React
Now we need to implement form validation in React after the user clicks submit button. For this, we need to store the form field values and check if it is valid or not and if they are not valid, suitable error message should be displayed under its field.
import React, { useState } from "react";
import "bootstrap/dist/css/bootstrap.min.css";
export default function App() {
const [name, setName] = useState("");
const [email, setEmail] = useState("");
const [phone, setPhone] = useState("");
const [password, setPassword] = useState("");
const [errorName, setErrorName] = useState("");
const [errorEmail, setErrorEmail] = useState("");
const [errorPhone, setErrorPhone] = useState("");
const [errorPassword, setErrorPassword] = useState("");
const emailRegEx =
/^(([^<>()[\]\.,;:\s@\"]+(\.[^<>()[\]\.,;:\s@\"]+)*)|(\".+\"))@(([^<>()[\]\.,;:\s@\"]+\.)+[^<>()[\]\.,;:\s@\"]{2,})$/i;
const passwordRegEx =
/^(?=.*[a-z])(?=.*[A-Z])(?=.*[0-9])(?=.*[!@#\$%\^&\*])(?=.{8,})/;
const phoneRegEx =
/^\s*(?:\+?(\d{1,3}))?[-. (]*(\d{3})[-. )]*(\d{3})[-. ]*(\d{4})(?: *x(\d+))?\s*$/;
const handleSubmit = (e) => {
e.preventDefault();
let isValid = true;
// form validation in React
//check if name is empty
if (!name) {
setErrorName("This field cannot be empty");
isValid = false;
}
//check if email is empty
if (!email) {
setErrorEmail("This field cannot be empty");
isValid = false;
}
// check if email is valid
else if (!email.match(emailRegEx)) {
setErrorEmail("Invalid Email");
isValid = false;
}
//check if email is empty
if (!phone) {
setErrorPhone("This field cannot be empty");
isValid = false;
}
// check if phone number is valid
else if (!phone.match(phoneRegEx)) {
setErrorPhone("Invalid Phone Number");
isValid = false;
}
//check if password is empty
if (!password) {
setErrorPassword("This field cannot be empty");
isValid = false;
}
// check if password contains atleast 8 characters including 1 uppercase letter and a special character
else if (!password.match(passwordRegEx)) {
setErrorPassword("Invalid Password");
isValid = false;
}
// if form is valid then submit form
if (isValid) {
alert("Validation OK");
}
};
return (
<form onSubmit={handleSubmit} className="col-10 m-4 p-2">
<h2>Sign Up Form</h2>
<div className="form-group mt-2">
<label>Name</label>
<input
type="text"
value={name}
onChange={(e) => {
setName(e.target.value);
setErrorName("");
}}
className="form-control"
/>
<span className="text-danger small">{errorName}</span>
</div>
<div className="form-group mt-2">
<label>Email</label>
<input
type="text"
value={email}
onChange={(e) => {
setEmail(e.target.value);
setErrorEmail("");
}}
className="form-control"
/>
<span className="text-danger small">{errorEmail}</span>
</div>
<div className="form-group mt-2">
<label>Phone</label>
<input
type="text"
value={phone}
onChange={(e) => {
setPhone(e.target.value);
setErrorPhone("");
}}
className="form-control"
/>
<span className="text-danger small">{errorPhone}</span>
</div>
<div className="form-group mt-2">
<label>Password</label>
<input
type="password"
value={password}
onChange={(e) => {
setPassword(e.target.value);
setErrorPassword("");
}}
className="form-control"
/>
<span className="text-danger small">{errorPassword}</span>
</div>
<input type="submit" value="Sign Up" className="mt-2 w-100 p-1" />
</form>
);
}
Here, we used the useState
hooks to store the values of the form fields during onChange
event. We created another state for storing the error messages of every field. We also called another function during onChange
event to clear the error message of that field.
After the user clicks the Sign Up button, the values of the form fields are checked. If all the values are valid, then the form is submitted else an error message is shown below the field whose value is not valid.
First of all, the fields are checked if they are empty or not. If they are empty, “This field cannot be empty” is displayed under the empty fields as an error message. If the field is not empty and some value is provided then that value is checked if it is valid or not.
We used regex to check the validation of the values. Regular expressions (regex) are special strings representing a pattern to be matched. If a value does not match the regex pattern then the error message is displayed.
Output
Conclusion
In this tutorial, we discussed form validation in React. We created a simple sign-up form using bootstrap. We checked if the fields are empty and validated the form field values using regex.