This article will show how to build a progressive web app using React.
What is a Progressive Web App (PWA)?
Progressive Web App is a web app built using the web technologies like HTML, CSS, JavaScript, etc that can behave both as a web page and a mobile app. It gives the feel and functionality of a mobile app as well as a web page.
The progressive web app must have a web manifest file served with a secure HTTPS connection and must register a service worker.
The app should be responsive so that it can be used on any screen-size device and the appearance should also look like a normal app.
Benefits of PWA
The benefits of a progressive web app (PWA) are:
- They can function both like web pages and normal native apps.
- They can be built with common web technologies.
- They are lightweight and load faster.
- They can work offline too.
- They can be installed easily.
- Developers can push new updates without waiting for any approvals
- They allow the implementation of different mobile features, such as push notifications.
- They are discoverable via search engines.
Examples of PWA
Some of the impressive Progressive Web App examples are:
Building a Progressive Web App using React
Now, let’s begin the tutorial to build a progressive web app using React. We will be making a simple counter app and build it as a progressive web app.
Step 1: Create a React App with cra-template-pwa
Template
npx create-react-app app-name --template cra-template-pwa
The project structure looks like this:
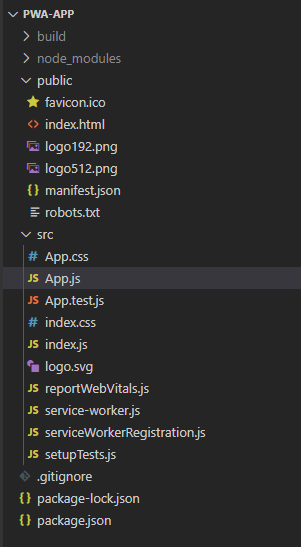
The files that are new from a normal react app structure are: service-worker.js
, serviceWorkerRegistration.js
and manifest.json
.
service-worker.js
runs in the background once the PWA starts and helps the app to work offline.
serviceWorkerRegistration.js
tells whether or not the service worker was successfully registered.
manifest.json
is a configuration file where we can customize the properties of a progressive web application such as icons, names, and colors to use when the application is displayed.
Step 2: Create a Component
Here we will be creating a counter component for this tutorial.
App.js
import React, { useState } from "react";
import "./App.css";
function App() {
const [count, setCount] = useState(0);
return (
<div className="app">
<h1>Counter App</h1>
<button onClick={() => setCount(count + 1)}>+</button>
<span>{count}</span>
<button onClick={() => setCount(count - 1)}>-</button>
</div>
);
}
export default App;
App.css
.app{
text-align: center;
}
button{
padding: 10px 20px;
font-size: 28px;
margin: 0px 10px;
}
span{
font-weight: 700;
font-size: 36px;
}
Step 3: Run the App
npm start
The Output of the App is
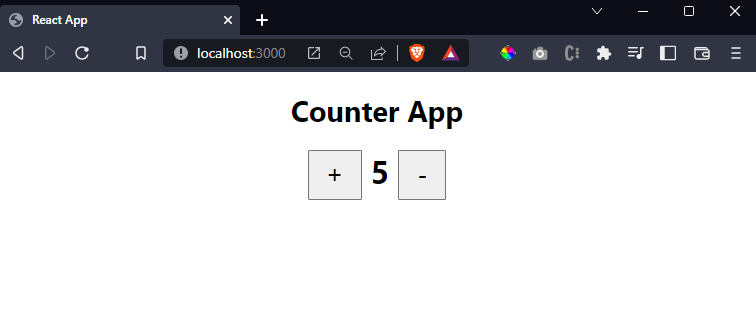
These are the normal steps to create and run a react app. Now, we need to follow a few more steps to make a progressive web app.
Step 4: Change the Service Worker Registration
In the index.js
file, we need to register the service worker by changing the line serviceWorkerRegistration.unregister()
to serviceWorkerRegistration.register()
. This makes the app work offline and load faster.
serviceWorkerRegistration.register();
Step 5: Deploy the App to a Static Server
Now, We need to deploy the app to a static server using serve
package to see the difference. We can install the serve
package using:
npm install serve
Then, build the application and deploy it using serve. This step should be repeated every time we need to deploy the changes to PWA.
npm run build
npx serve -s build
We can see a message like this in the terminal after the app is deployed.
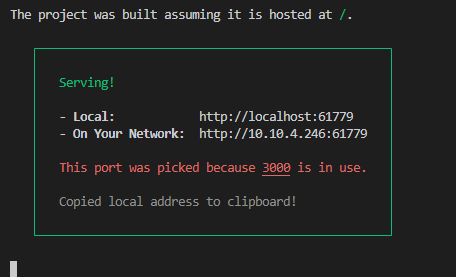
Follow the provided url and the application looks like this:
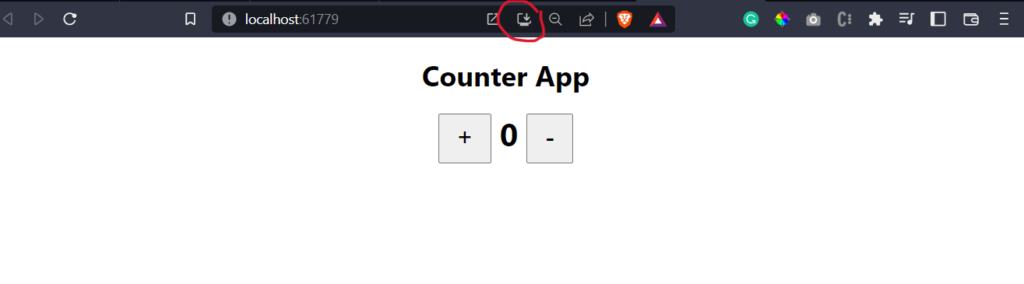
The output looks similar to the above output of react app. But this progressive web app provides a download button that helps to download the PWA to our device.
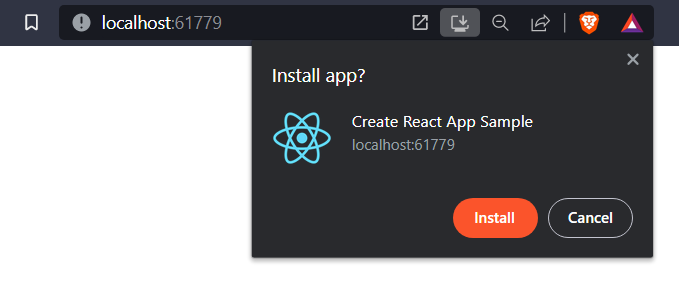
If we install the app and open it. We can see a native app version of our application.
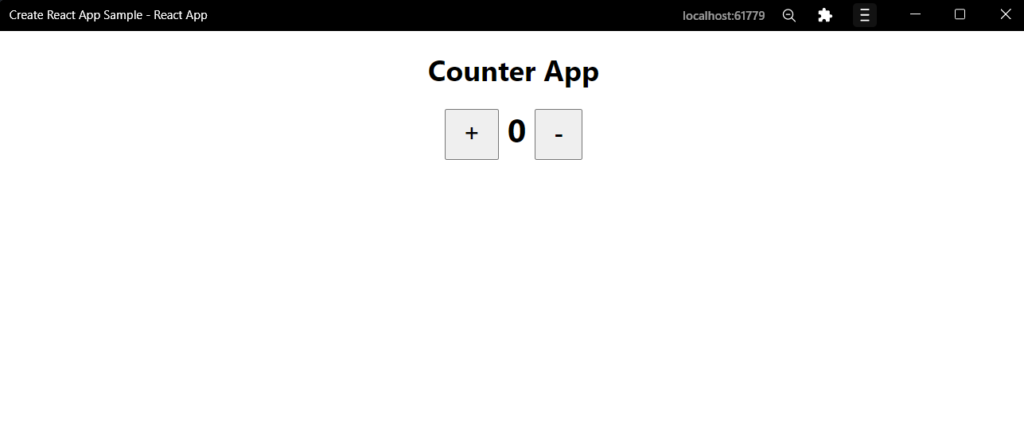
We can test the performance of our PWA using Lighthouse. It runs a series of audits against the page, and then it generates a report on how well the page did.
Conclusion
In this article, we discussed the progressive web app, also known as PWA. We learned the basic concepts of PWA and a tutorial on how to build a PWA using React.