Introduction
In this article we will be learning about types of directives in angular.
Prerequisite
- Basic knowledge of Angular
- Setup of a local development environment. You will need Angular CLI to set up your environment.
Directives
Directives in Angular are the class which allow us to add additional behavior and modify existing behaviour to our elements . We can use the directives to manage forms, lists, styles.
Directives are needed when we want to modify the structure of the DOM i.e. add or delete the HTML element or to modify the appearance of the DOM or component.
Types of Directives
- Structural Directives
- Attribute Directive
- Component Directive
Structural Directives
It is used to change structure of DOM by adding and removing DOM elements. This directives have a *
sign before the directive. They are mainly used to create, destroy, hide or disable DOM elements.
Types of Structural Directives
- ngIf
- ngFor
- ngSwitch
ngIf
It is used to display or hide an element based on the condition determined by the expression we pass into the directive.The element will be removed if the result of expression is return false.
Example
Let’s declare name variable with string value ryan in app.component.ts
file.

Add the code below in app.component.html
file.
<div *ngIf="name == 'ryan'">
My name is Ryan
</div>
Here we have added condition to show div element if the value of variable name is ryan.
Results
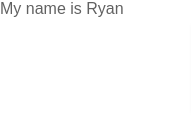
ngFor
In this directive, DOM elements are repeated and one element from an array is passed each time.
It is the structural directive which will add or remove components from the DOM.
ngFor is basically a for loop which is used to create some repeating elements on the DOM.
Example
Let’s declare array variable todos in app.component.ts
file.
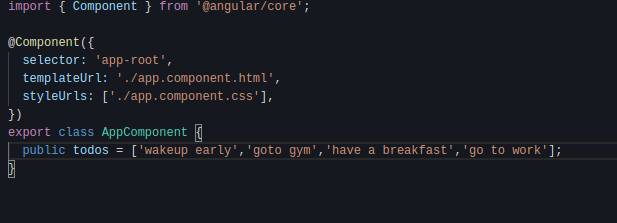
add the code below in app.component.htm
l file.
<div class="list" *ngFor="let item of todos">
<div class="item">{{ item }}</div>
</div>
Here we have repetitive loop to display todo item in div element.
Results

ngSwitch
This directive is allow us to add or remove the element depending on a match expression.
It is used to render different elements depending on a given condition.
It contains three separate directives. ngSwitch
, ngSwitchCase
& ngSwitchDefault
.
Example
let’s declare variable with name myName and which have value 1 in app.component.ts file.
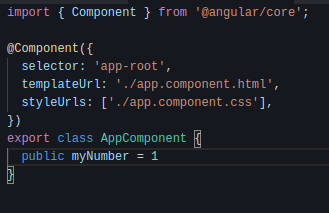
And add the code below in app.component.html
file.
<div class="container" [ngSwitch]="myNumber">
<div *ngSwitchCase="'1'">Number is 1</div>
<div *ngSwitchCase="'2'">Number is 2</div>
<div *ngSwitchCase="'3'">Number is 3</div>
<div *ngSwitchDefault>Number is something else</div>
</div>
Here we have add the condition to show the text based on the value of the myNumber variable value.
Since the value is 1 now, the div element with text Number is 1 will be visible.
Results

Attribute Directive
Attribute directives are used to apply conditional style to elements, to show or hide elements, or to dynamically change a component’s behavior. It can change the appearance or behavior of an element.
ngStyle
It is used to add or modify the style of DOM based on the some condition.we can use ngStyle
directive to change the style of the element.
Example
Add the code below in app.component.html
file.
<div [ngStyle]="{'background-color': 'blue','color':'black', 'font-size': '24px', 'font-weight': 'bold'}">
Sample text
</div>
Here we have added the style with properties background color,color,font size and font weight.
Results
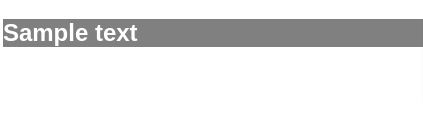
Conditional ngStyle Example
We can also added css properties based on the result of the expression.
Let’s declare the variable with name userList in the app.component.ts
file.

Here we have defined userList with their id,name and role.
Add the code below in app.component.html
file.
<div class="list" *ngFor="let item of userList">
<div class="item" [ngStyle]="{'color': item.role == 'admin' ? 'green' : 'blue'}">
{{ item.name }} - {{item.role}}
</div>
</div>
Here we displayed the list of user with their name and role and added the conditional ngStyle
directive to show the color according to role.If the user is admin ,font color will be green and else color will be blue.
Results
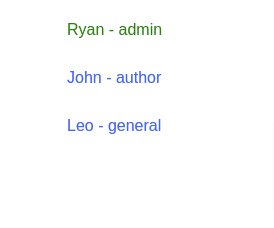
ngClass
The NgClass directive, represented by a ngClass attribute in your HTML template which allows us to dynamically set and change the CSS classes for a DOM element.It can be used in a variety of ways to change styles and hide or show content.We can see the class name when we inspect the DOM element.
//basic usages
<div [ngClass]="'first-element'">
ngClass example
</div>
Conditional ngClass Example
We can add class based on the condition and after that we can add the style as required in the DOM element.Let’s add new variable with named color in app.component.ts
.
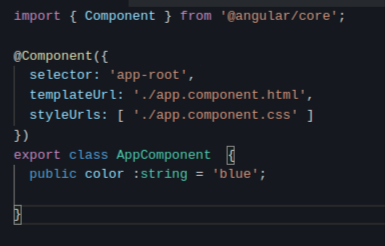
Add the code below in app.component.css
and app.component.html
file.
//app.component.css
.bg-blue {
background-color: blue;
color: white;
}
.bg-default {
background-color: grey;
color: black;
}
div{
margin: 40px;
}
//app.component.html
<div [ngClass]="color == 'blue' ? 'bg-blue' : 'bg-default'">
Conditional NgClass Example
</div>
Here we have added the css for bg-blue and bg-default class in app.component.css and added the class by color value.According to our condition if color variable value is blue then bg-blue class will be added else bg-default will be added to DOM element.
Result
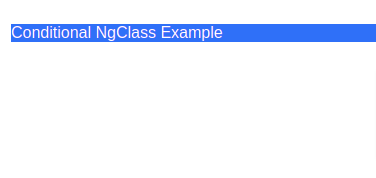
Component Directive
Component are directives in the template that must be declared in Angular modules similarly to component declearations.It is special directives with a template where as structural and attribute doesn’t have any template.Controllers and templates are part of a component directive. It contains details of how the component should be processed, instantiated and used at runtime.
Conclusion
From this article we have learned about the directives and its types in angular.