Introduction
In this article we will be learning about pipe decorator in Angular
Prerequisite
- Basic knowledge of Angular
- Setup of a local development environment. You will need Angular CLI to set up your environment.
Pipe
Pipe is decorator which transform the data before displaying in the template.It is used by using ‘|’ operator.Pipe takes data as input and transforms it input into the desired output.It is simply functions that can be applied directly to an expression in a template to transform it into another format.It allows users to change the format in which data is being displayed on the screen.
Syntax : {{ input
|
pipeName
}}
Types of built in pipe
- DatePipe
- UppercasePipe
- LowercasePipe
- CurrencyPipe
- DecimalPipe
- PercentPipe
DatePipe
DatePipe is used to transform date format according to given date format.
Syntax : {{ input
|
pipeName
}}
Example
Let’s declare variable date which represent today date value in app.component.ts file.
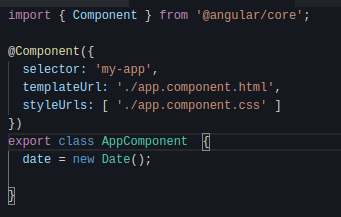
add the code below in app.component.html
file
<div>
Today's Date is:- {{ date | date:'d/M/yyyy' }}
</div>
Here we have used the date pipe which will transform the date in date/month/year format.
Results
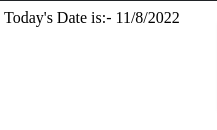
UppercasePipe
UppercasePipe is used to transform string or text to uppercase in the template.
Syntax : {{ value | uppercase
}}
Example
Let’s declare variable with string value in app.component.ts
file.
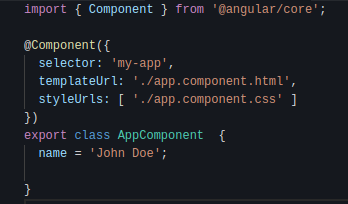
add the code below in app.component.html
file
<div>
Name in Uppercase : {{ name | uppercase}}
</div>
Results

LowercasePipe
LowercasePipe is used to transform string or text to uppercase.This will transform all the letter of string to lowercase.
Syntax : {{ value | lowercase
}}
Example
Here we can use the same name variable which we have defined in app.component.ts
file for the UppercasePipe example.
add code below in app.component.html
file
<div>
Name in LowerCase : {{ name | lowercase}}
</div>
Results

CurrencyPipe
CurrentPipe is used to transform and format numbers into currency strings using locale rules.
Syntax
{{ value | currency [ : currencyCode [ : display [ : digitsInfo [ : locale ] ] ] ] }}
Format of Currency Pipe:
- value: The number to be formatted as a currency strings.
- percent: Keyword which is used with pipe operator.
- digitsInfo: Decimal representation of currency value
- locale : Represents locale format rules.
Example
Let’s declare variable with name price which have currency number value in app.component.ts
file.
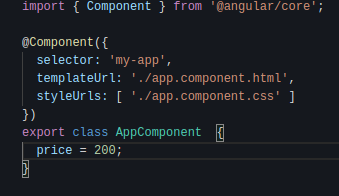
<div>Price: {{price | currency}}</div> //default currency format will be in dollar
<div>Price: {{price| currency:'NPR'}}</div>
<div>Price: {{price | currency:'NPR':'code'}}</div>
<div>Price: {{price | currency:'NPR':'symbol':'4.2-2'}}</div>
<div>Price: {{price | currency:'NPR':'symbol':'4.2-2':'en'}}</div>
DecimalPipe
DecimalPipe is used to transform number into decimal strings using localization.It is used to format decimal numbers. It uses number
keyword with pipe operator.
Syntax
{{ value | number [ : digitsInfo [ : locale ] ] }}
Format of DecimalPipe
value: The number to be formatted as a decimal.
number: Keyword which is used with pipe operator.
digitsInfo: Decimal representation include there parameter.
locale : Represents locale format rules.
Digitinfo parameter
DigitsInfo represent decimal strings in follow format :
{minIntegerDigits}.{minFractionDigits}-{maxFractionDigits}.
minIntegerDigits : Minimum number of integer digits. Default is 1.
minFractionDigits : Minimum number of fraction digits. Default is 0.
maxFractionDigits : Maximum number of fraction digits. Default is 3.
Basic Example
Let’s declare variable with name mynumber in app.component.ts
file.
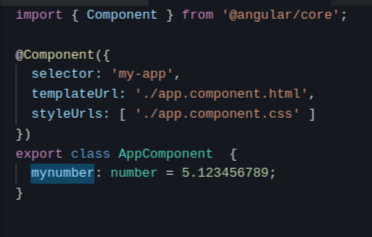
add code below in app.component.html file
<div>
<p>Default Decimal Pipe - {{ mynumber | number }} </p>
</div>
Result
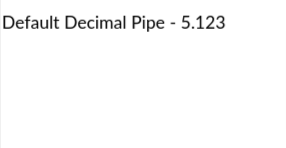
Example with digitinfo
Add code below in app.component.ts
and app.component.html
.
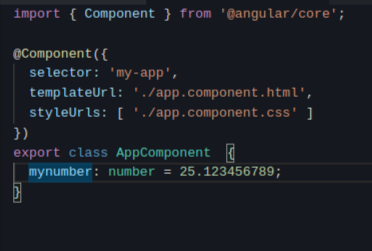
<div>
<p>No format 2.1-3 - {{ mynumber | number: "2.1-3" }}</p><br>
<p>No without Decimal Point - {{ mynumber | number: "2.0-0" }} </p>
</div>
Here we shown two element with digit infor in decimal pipe.The first element with format ‘2.1-3’ which will follow the format below
minIntegerDigits = 2
minFractionDigits = 1
maxFractionDigits = 3
Result
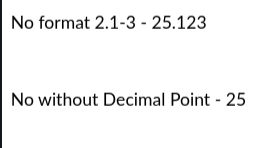
PercentPipe
PercentPipe is use to transforms a number to the percentage string formatted according to locale rules .
In PercentPipe, the value is multiplied by 100 to calculate the percentage, just like NumberPipe.
Syntax:
{{ value | percent [ : digitsInfo [ : locale ] ] }}
format of PercentPipe
- value: the number to be formatted as a percentage.
- percent: Keyword which is used with pipe operator. .
- digitsInfo: A decimal format representation in string format, used to round percentages.
Digits info parameter will be the same which we have described in decimal pipe above.
Example
Add the code below in app.component.html
file.
<p>
Default percentpie - {{0.23 | percent }}
<br>
Format with 1.2-2 - {{0.2345 | percent:'1.2-2'}} <br>
Format with 1.2-2 and roundoff value - {{0.2345678 | percent:'1.2-2'}} <br>
</p>
Result
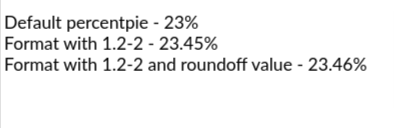
Conculsion
In this article we have learned about angular pipe decorator with their example.