Introduction
Last time we discussed server side rendering and the basic setup and installation steps for using the Angular Universal . Today, we are going to implement the SEO meta tags with Angular Universal in our project.
How To Implement SEO Meta Tags In Angular Universal
Import Angular SEO Meta Services
In order to use angular meta services, we need to import meta and title from @angular/platform-browser angular library, where we can write functions to implement SEO meta services.
Import meta and title from @angular/platform-browser
import { Meta, Title } from '@angular/platform-browser';
Now we can add them to the constructor to use the services. And we’ll add the title and meta description for the individual page.
Add code below in app.component.ts file.
import { Component } from '@angular/core';
import { Meta, Title } from '@angular/platform-browser';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
constructor(private titleService: Title, private metaService: Meta) {}
ngOnInit() {
this.titleService.setTitle('Demo meta tag');
this.metaService.addTags([
{
name: 'keywords',
content: 'Demo Angular Universal Meta Tags',
},
{ name: 'robots', content: 'index, follow' },
{ name: 'author', content: 'Manish Koju' },
{ name: 'viewport', content: 'width=device-width, initial-scale=1' },
{ name: 'date', content: '2022-03-02', scheme: 'YYYY-MM-DD' },
{ charset: 'UTF-8' },
]);
}
}
Now, run these commands
npm run build:ssr
npm run serve:ssr
We can see our new added meta tag when we view source the page
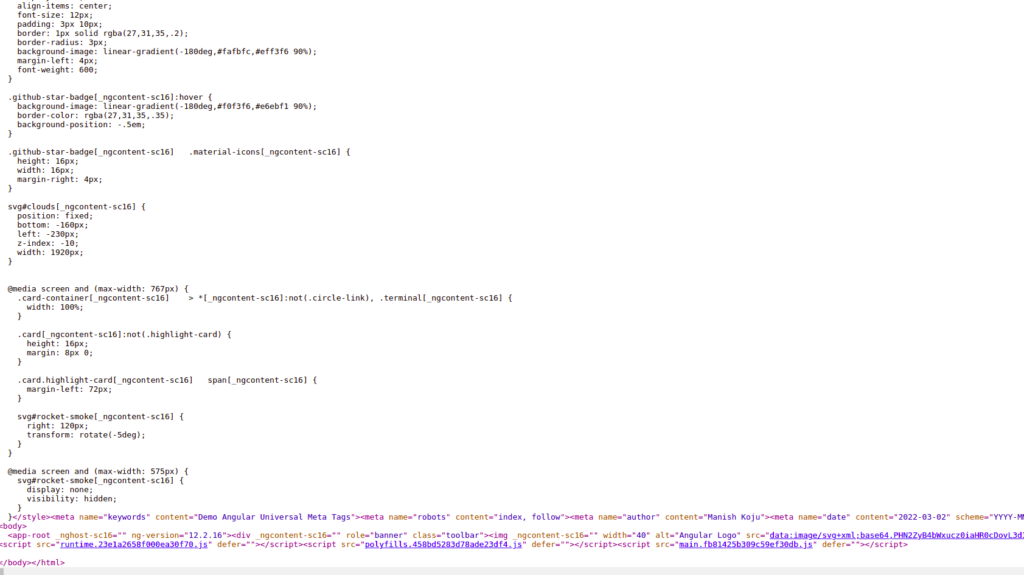
Functions in Meta Service
- addTag(): Used to add one meta tag
- addTags(): Add more than one meta tag in angular component
- getTag(): Gets HTMLMetaElement for a specified meta selector.
- getTags(): Returns array of HTMLMetaElement for the specified meta selector
- updateTag(): It is used to update the existing meta tag by name.
- removeTag(): Used to remove meta tag for the specified selector.
- removeTagElement(): Removes meta tag for the specified HTMLMetaElement
Conclusion
This article explains how to set up Angular Universal to display SEO meta tags in Angular. We have learned to set up page title, meta description, author name, keywords, charset, viewport, and robots. Hence, to make your Angular application SEO friendly, we must first convert it into Server Side Rendering by using Angular Universal.