Image manipulation is the art of altering or modifying an image for different purposes using various techniques or using photo editing software. In this article, we will be performing image manipulation using jimp. Jimp also known as Javascript Image Manipulation Program is one of the best image manipulation packages available for node js. It allows you to easily manipulate and transform your images into any required format, style, or dimension. It also optimizes images for minimal file size, ensures high visual quality for an improved user experience, and reduces bandwidth. Jimp only supports the following types:
- @jimp/jpeg
- @jimp/png
- @jimp/bmp
- @jimp/tiff
- @jimp/gif
Prerequisites
- A basic understanding of Node js
How to perform image manipulation using jimp in node js?
Project Setup
Create a directory name image-manipulation
, and change it into that directory using the following command.
mkdir image-manipulation
cd image-manipulation
Inside the image-manipulation
directory, initialize a node js project using
npm init
After the command, a new package.json file will be created in the image-manipulation
folder all the dependencies needed for our project will be added.

Now, let’s install the required dependencies required to manipulate images in node js.
npm install express jimp
This statement will install express and JIMP packages which will be used for routing and image manipulation respectively.
Next, let’s create a index.js
file where we will create a route using which image manipulation will be performed.
const express = require('express')
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.get("/image-manipulate", imageManipulate);
app.listen(3000, function () {
console.log('App listening on port 3000!');
});
The above code will initialize the express framework, set up the server at port 3000, and create a route image-manipulate
that is used to manipulate images.
Image Manipulation
In this step, let’s create a file where we will write the logic for image manipulation using JIMP. Let the name of the file be manipualte.js
which will be located inside our root folder. Add the following code block in the manipulate.js
file.
#manipulate.js
const Jimp = require("jimp");
const imageManipulate = async (req, res) => {
const imgUrl = "https://images.pexels.com/photos/1563356/pexels-photo-1563356.jpeg?cs=srgb&dl=pexels-craig-adderley-1563356.jpg&fm=jpg"; // Sample Image Link
Jimp.read(imgUrl)
.then(img => {
return img
.resize(Jimp.AUTO, 480)
.quality(100) // set JPEG quality
.write('./out.jpg'); // save
})
.catch(err => {
console.error(err);
});
};
module.exports = imageManipulate;
Here, we have used some random images from pixels for testing. Then,
Jimp.read(imgUrl)
: The provided sample images are processed using this command.
resize(Jimp.AUTO,480)
: This command resizes the original image the height to 480 and scales the width accordingly.
quality(100)
: This command determines the quality of the output image where the value should be between 0-100.
write('./out.jpg')
: This command saves the output image in the storage named out.jpg
.
Output after processing:
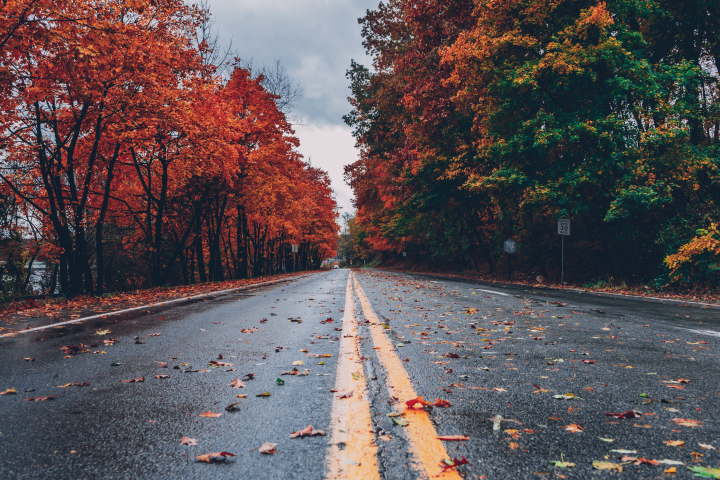
Similarly, we can also add a watermark to the image using jimp. Replace the code in manipulate.js
a file with the following.
#manipulate.js
const Jimp = require("jimp");
const imageManipulate = async (req, res) => {
const imgUrl = "https://images.pexels.com/photos/1563356/pexels-photo-1563356.jpeg?cs=srgb&dl=pexels-craig-adderley-1563356.jpg&fm=jpg";
const watermarkLink = "./watermark.png";
const [image, watermark] = await Promise.all([
Jimp.read(imgUrl),
Jimp.read(watermarkLink),
]);
watermark.resize(image.bitmap.width / 5, Jimp.AUTO);
const xMargin = image.bitmap.width / 2;
const yMargin = image.bitmap.height / 2;
const X = image.bitmap.width - watermark.bitmap.width - xMargin;
const Y = image.bitmap.height - yMargin;
const watermarkImage = image
.composite(watermark, X, Y)
.quality(30)
.write('./watermark_output.jpg');
};
module.exports = imageManipulate;
Here,
- We have used two images
imgUrl
andwatermarkLink
one for normal image and the other for watermark respectively. - Using promise we read both the image from the URL and store it in respective variables
- Then, we resize the watermark and define the position in the original in which the watermark will be added.
- Finally, we merge both images and store the output in the file named
watermark_output.jpg
file.
Image for Watermark

Output after adding a watermark

Conclusion
In this article, we have how to perform image manipulation using jimp in node js. This is just a surface of what jimp is capable of doing. If you want to perform image manipulaion using other packages like sharp you can get knowledge from here and you can follow this link to learn about video manipulation in node js.