Let’s learn how to run raw FFmpeg commands in NodeJs. We will achieve this using npm packages fluent-ffmpeg, and @ffmpeg-installer/ffmpeg.
Introduction
FFmpeg is a cross-platform multimedia framework that can decode, encode, stream, filter, and play video and also audio files. FFmpeg likewise supports a wide number of operating system infrastructures like Linux, Mac OS X, BSD, Solaris, Microsoft Windows, etc.
NodeJS has multiple packages to run FFmpeg, but the fluent-ffmpeg
is one of the more popular packages that is used to interact with FFmpeg through NodeJS. The @ffmpeg-installer/ffmpeg
package installs a binary of FFmpeg for the current platform and provides a path and version. Supports Linux, Windows, and Mac OS/X. Thus, the use case of using FFmpeg with NodeJS is many like streaming audio and videos as it is used for in many cases. While we know about it, let’s create the node application to run the FFmpeg commands for getting a clear view.
Prerequisites
- Basic understanding of Nodejs
Run FFmpeg Commands in NodeJS
Let’s first create a NodeJS project and install the required dependencies.
## Creating a node project
$ npm init --y
## installing fluent-ffmpeg and @ffmpeg-installer/ffmpeg npm packages
$ npm install fluent-ffmpeg @ffmpeg-installer/ffmpeg
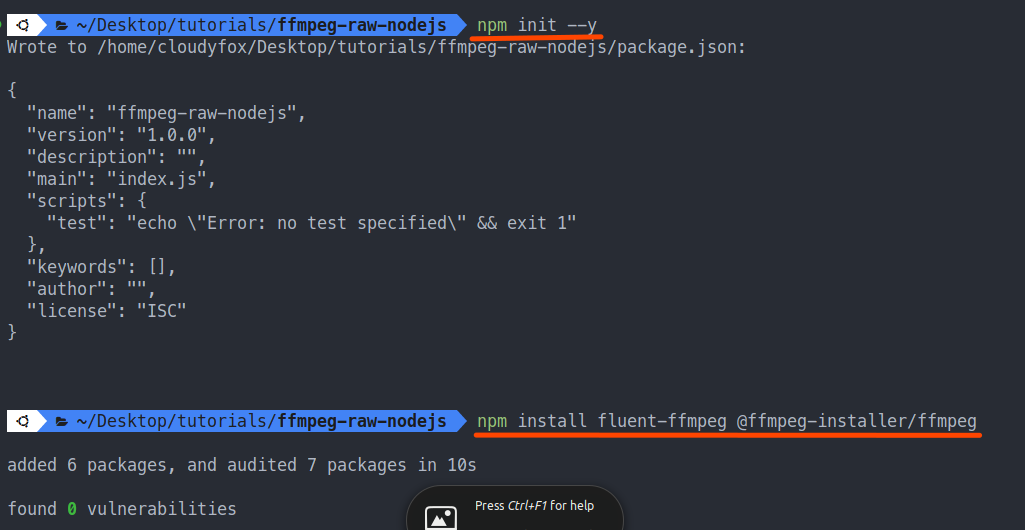
Now, that the project is generated and npm packages are installed let’s create a start point for our application in our case which is index.js
. Also, we will create an input and output directory to keep our input video and output video respectively.
## generate project files and folders
$ touch index.js
$ mkdir input
$ mkdir output
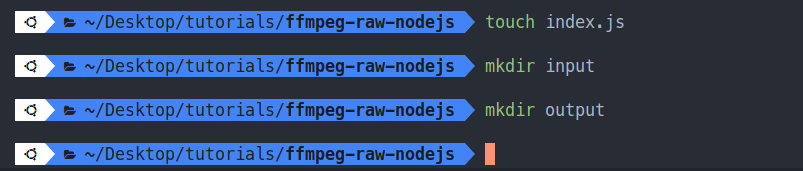
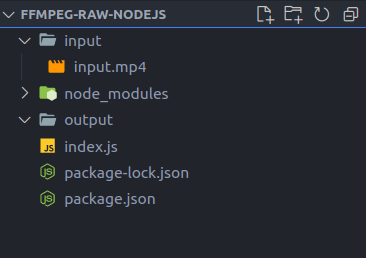
Now, that the project is generated, all the required packages are installed, and the input file is also created. Finally, let’s write the code that runs raw FFmpeg commands in NodeJS.
The FFmpeg code we will execute is ffmpeg -i ./input/input.mp4 -vf "transpose=0" ./output/output.mp4
. This will rotate the input video 90°
and saves it in the output video file.
//* index.js
const ffmpeg = require("fluent-ffmpeg");
const ffmpegInstaller = require("@ffmpeg-installer/ffmpeg");
const ffmpegPath = ffmpegInstaller.path;
ffmpeg.setFfmpegPath(ffmpegPath);
/*
Refer: https://stackoverflow.com/questions/59800915/how-can-i-execute-a-custom-ffmpeg-string-command-with-fluent-ffmpeg
Generates the ffmpeg code from the sent raw code
*/
const executeFfmpeg = (args) => {
let command = ffmpeg().output(" ");
command._outputs[0].isFile = false;
command._outputs[0].target = "";
command._global.get = () => {
return typeof args === "string" ? args.split(" ") : args;
};
return command;
};
//* Raw FFmpeg code is given here, it generates the output file
executeFfmpeg(`-i ./input/input.mp4 -vf transpose=0 ./output/output.mp4`)
.on("start", (commandLine) => console.log("start", commandLine))
.on("codecData", (codecData) => console.log("codecData", codecData))
.on("error", (error) => {
console.log("error", error);
})
.on("stderr", (stderr) => console.log("stderr", stderr))
.on("end", () => {
console.log("Video Generation Complete.");
})
.run();
In the above code, the executeFfmpeg
function parses and returns the command method of the fluent-ffmpeg
package. So, Let’s run the above code and look at the output of the program.
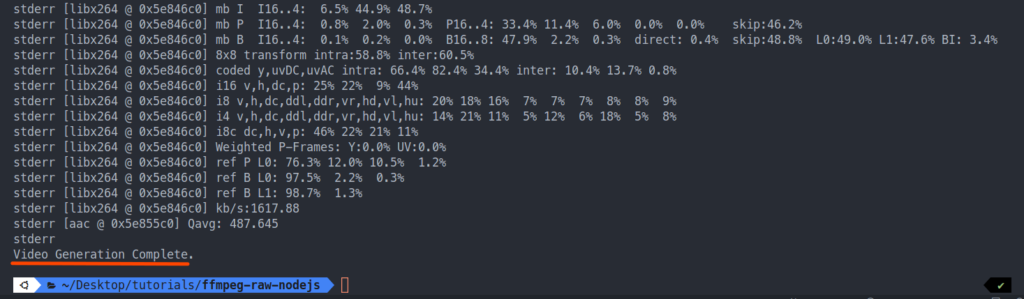
As we can the process is complete and the file is kept in the output directory as output.mp4
.
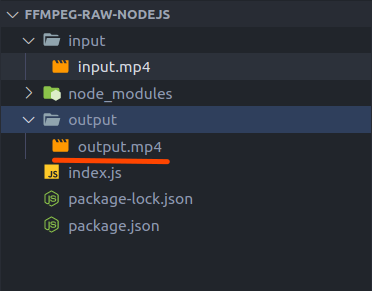
The above video is the output generated by executing the raw FFmpeg command from Node and is present in the output directory of the project. The video of the output is rotated 90°
as we can see in the above video.
Conclusion
So, in this article, we learned about executing raw FFmpeg commands in NodeJS and saw the results of using it. Likewise, you can use the code to run other FFmpeg command to get your desired output.