Let’s learn how to add swagger to an Express application. We will do this by using the npm packages swagger-jsdoc, and swagger-ui-express.
Introduction
Swagger is a tool used for API documentation that uses the Open API specification. Overall it provides and helps to create, test, and visualize RESTful APIs. Swagger is created and deployed separately from the actual application which is used to build the RESTful APIs.
To add swagger to Express Application we need to use two external node packages.
- swagger-jsdoc: This library reads your JSDoc-annotated source code and generates an OpenAPI (Swagger) specification.
- swagger-ui-express: This module allows you to serve auto-generated swagger UI. It serves the swagger documentation in a route.
The swagger-jsdoc
translates the JSDocs annotated with @swagger
or @openapi
and it then converts it into swagger documentation. The swagger-ui-express
serves swagger documentation, in swagger-ui
format.
Prerequisites
Integrating Swagger to An Express Application
Let’s first create a node.js application, and later add the required packages to add swagger to an express application.
## Creating a node application
$ npm init --y
## Installing required packages
$ npm install express swagger-jsdoc swagger-ui-express
## Open your application with your favourite
$ code .
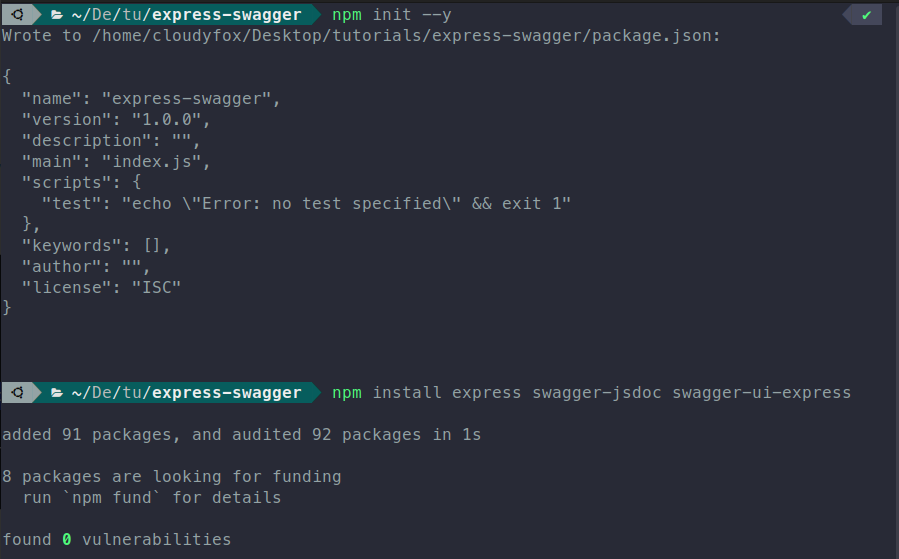
Now that the node application is created, create a index.js
file that is the starting point for the application. Firstly, let’s set up the express application.
//* index.js
const express = require("express");
const swaggerJSDoc = require("swagger-jsdoc");
const swaggerUI = require("swagger-ui-express");
const app = express();
const PORT = process.env.PORT || 3000;
//* Swagger Configuration options
const swaggerOptions = {
swaggerDefinition: {
info: {
title: "Swagger API",
version: "1.0.0",
},
},
apis: ["index.js"],
};
//* setting up the swagger-jsdoc
const swaggerDocs = swaggerJSDoc(swaggerOptions);
//* serving the swagger docs through the swagger-ui-express
app.use("/api-docs", swaggerUI.serve, swaggerUI.setup(swaggerDocs));
/*
---- YOUR ROUTES GO HERE ----
*/
app.listen(PORT, () => console.log(`Application Started on PORT ${PORT}`));
The swagger docs are added, now let up look at adding the swagger code. The swagger code is added on top of each route. Such implementation is shown in the GET
route below.
//* index.js
/**
* @swagger
* /:
* get:
* description: Get
* responses:
* 200:
* description: OK
*
*/
app.get("/", (req, res) => {res.send("OK")});
The swagger code is added in JSDoc format, which basically starts with the keyword @swagger
. The code that goes inside it is in the Open API specification and it is the same code that is written while creating API specification using swagger.
Similarly let’s add the same to each ["POST", "PUT", "DELETE"]
route, which will help us achieve adding swagger to an express application. The finalized code in the file index.js
is presented below.
//* Finalized index.js file
const express = require("express");
const swaggerJSDoc = require("swagger-jsdoc");
const swaggerUI = require("swagger-ui-express");
const app = express();
const PORT = process.env.PORT || 3000;
//Swagger Configuration
const swaggerOptions = {
swaggerDefinition: {
info: {
title: "Swagger API",
version: "1.0.0",
},
},
apis: ["index.js"],
};
const swaggerDocs = swaggerJSDoc(swaggerOptions);
app.use("/api-docs", swaggerUI.serve, swaggerUI.setup(swaggerDocs));
/**
* @swagger
* /:
* get:
* description: Get all Items
* responses:
* 200:
* description: Operation Successful
*
*/
app.get("/", (req, res) => {
res.json([
{
id: 1,
item: "Banana",
},
{
id: 2,
item: "Car",
},
{
id: 3,
item: "Apple",
},
]);
});
/**
* @swagger
* /:
* post:
* description: Create an item
* parameters:
* - item: Item Name
* description: Create new item
* in: formData
* required: true
* type: String
* responses:
* 200:
* description: Operation Successful
*
*/
app.post("/", (req, res) => {
res.status(200).send('Operation Successful');
});
/**
* @swagger
* /:
* put:
* description: Edit Item
* parameters:
* - item: Item Name
* description: Edit Item
* in: formData
* required: true
* type: String
* responses:
* 200:
* description: Updated Successfully
*
*/
app.put("/", (req, res) => {
res.status(200).send("Updated Successfully");
});
/**
* @swagger
* /:
* delete:
* description: Delete item
* parameters:
* - name: EmployeeName
* description: Delete
* in: formData
* required: true
* type: String
* responses:
* 200:
* description: Deleted Successfuly
*
*/
app.delete("/", (req, res) => {
res.status(200).send('Deleted Successfuly');
});
app.listen(PORT, () => console.log(`Application Started on PORT ${PORT}`));
Now that the application is finalized. Finally, let’s run the node application.
## Running the node project
$ node index.js
The result obtained by going into localhost:3000/api-docs
is shown below.
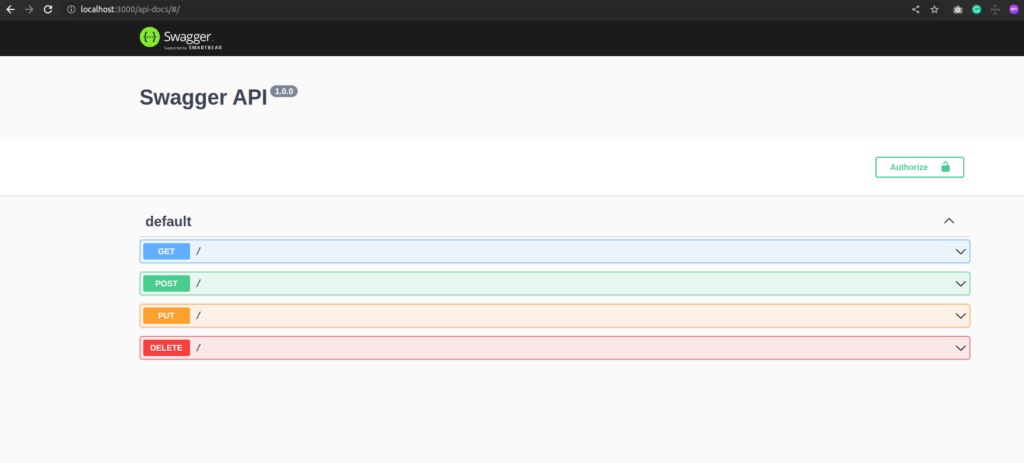
Conclusion
So in this article, we learned and added swagger to an express application, and looked at the result of it. To learn about serving API Spec written in Swagger-based OpenAPI specification using docker look into this article.