The purpose of this article is to explain how to export files in Laravel 9 step by step. If you are working with anything related to the reporting, we often need to download that data in excel or CSV format. Using Laravel excel we can easy to download the data in excel or CSV format.
The Laravel Excel package simplifies exports and imports by wrapping PhpSpreadsheet with a manageable and elegant solution. The PhpSpreadsheet library is written in pure PHP and includes classes for reading and writing spreadsheets such as Excel and LibreOffice Calc. The Laravel excel package is one of the best and easiest available packages to import and export data in multiple file formats. Some of the available formats are : xlsx
, csv
, ods
, xls
and html
.
Prerequisites
- A basic understanding of Laravel
How To Export Files in Laravel 9?
Project Setup
First, let’s create a Laravel 9 project using the following command.
composer create-project laravel/laravel:^9.0 file-export
Now, install the dependencies required for file export.
composer require psr/simple-cache:^2.0 maatwebsite/excel
Create a database and modify the env file according to your database configuration like below:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=test_db
DB_USERNAME=test_user
DB_PASSWORD=test_password
Creating sample data for export
Let’s create dummy data using tinker which will later be used for export.
php artisan tinker
User::factory()->count(10)->create()
This will create a random 10 dummy user data in the database according to the configuration provided in the .env
file.
Exporting data
Let’s create an export class that will contain the data to be exported and the data modification code.
php artisan make:export UsersExport
This will create a UsersExport.php
file inside the Exports
folder. Update the file content with the following code.
<?php
namespace App\Exports;
use App\Models\User;
use Maatwebsite\Excel\Concerns\FromCollection;
use Maatwebsite\Excel\Concerns\WithHeadings;
class UsersExport implements FromCollection, WithHeadings
{
/**
* @return \Illuminate\Support\Collection
*/
public function collection()
{
return User::select("id", "name", "email")->get();
}
/**
* Write code on Method
*
* @return response()
*/
public function headings(): array
{
return ["ID", "Name", "Email"]; //Heading of the file downloaded
}
}
Here, the collection
function will return id
, name
and email
of the user and the headings
function will return the heading displayed in the exported file. Similarly,
Now, let’s create a controller to access the export class and export the file.
php artisan make:controller UserController
The above artisan command will create a UserController
file inside the controller folder. Now, let’s create a function that will be used to export the user data.
<?php
namespace App\Http\Controllers;
use App\Exports\UsersExport;
use Maatwebsite\Excel\Facades\Excel;
class UserController extends Controller
{
/**
* @return \Illuminate\Support\Collection
*/
public function export()
{
return Excel::download(new UsersExport, 'users.xlsx'); //download xlsx format file
}
}
Here, the downloaded file will be named users.xlsx
. Similarly, we can also export the user data into CSV file format by replacing the above download command with following
return Excel::download(new UsersExport, 'users.csv'); //download csv format file
We can also directly store the file in the storage without downloading the file.
return Excel::store(new UsersExport, 'users.xlsx', 's3');
The above code will store the users.xlsx
file directly to the s3 storage without the need to download the file to our local machine.
Now, let’s create a route using which we will be able to access the export function and download the user data.
<?php
use Illuminate\Support\Facades\Route;
Route::get('/export', [App\Http\Controllers\UserController::class, 'export'])->name('export');
After creating the above route, we can export the file using the route localhost:8000/export
. To be able to access this route first we have to run the Laravel application which can be done using the following command.
php artisan serve
The export file will look similar to the following image.
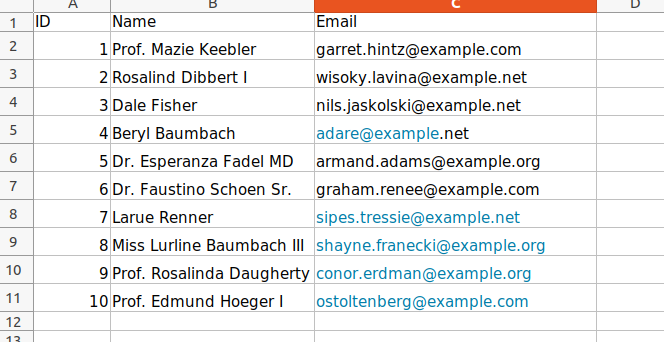
Conclusion
In this article, we have learned how to export files in Laravel 9 using the Laravel Excel package. Similarly, we can use the Laravel Excel package to import the data in Laravel. If you want to attach the excel file in an email you can use this link. If you are searching for exporting files in other languages like Node js you can follow this link.