In this article, we will learn about how to use transactions in DynamoDB. NoSQL refers to a non-SQL or a non-relational database that provides a mechanism for the storage and retrieval of data modeled in other than tabular relations(unlike relational databases). DynamoDB is a fully managed proprietary NoSQL database service that supports key-value and document data structures. in the DynamoDB table, each item is uniquely identified by a primary key. DynamoDB supports two types of primary keys: a simple primary key made up of just a partition key, and a composite primary key made up of a partition key and a sort key.
Transactions in DynamoDB allow you to perform multiple database-related actions together as a single all-or-nothing operation. The transaction operations are useful when dealing with mission-critical data like currency, item stocks, etc. Imagine a scenario, when sending money from account A to B. This scenario involves subtracting money from account A and adding it to account B. However, if your application crashes before completing both operations. This will create corrupt data on the database and a portion of the money would be lost. With the help of transactions, we can prevent this scenario from happening. The transactions return success only if both operations are completed and return an error if any one or more operations return an error.
Prerequisites
Transactions in DynamoDB
let’s initialize the SAM project using the following steps:
sam init
## for template source choose -> 1 - AWS Quick Start Templates
## for package type -> 1 - Zip (artifact is a zip uploaded to S3)
## for run time choose -> 1 - nodejs14.x (as the article is made for node)
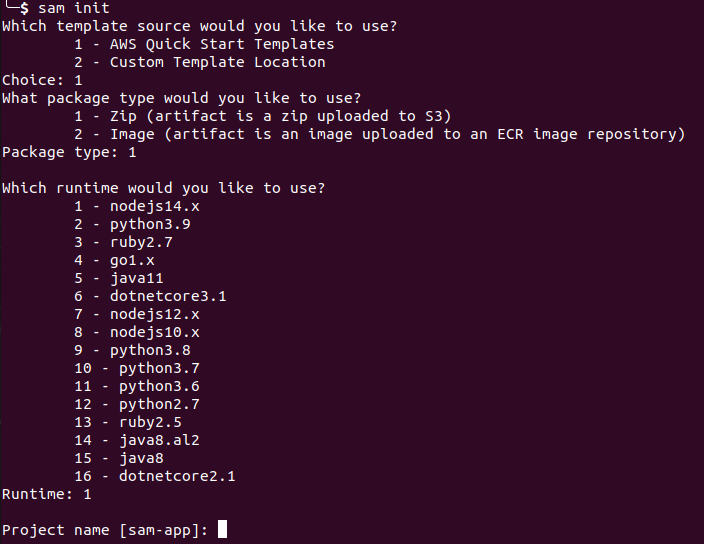
Enter the name you want for your project. We will use dynamo-transaction
for this article. Then let’s choose option 1 (Hello World Example) for this article.
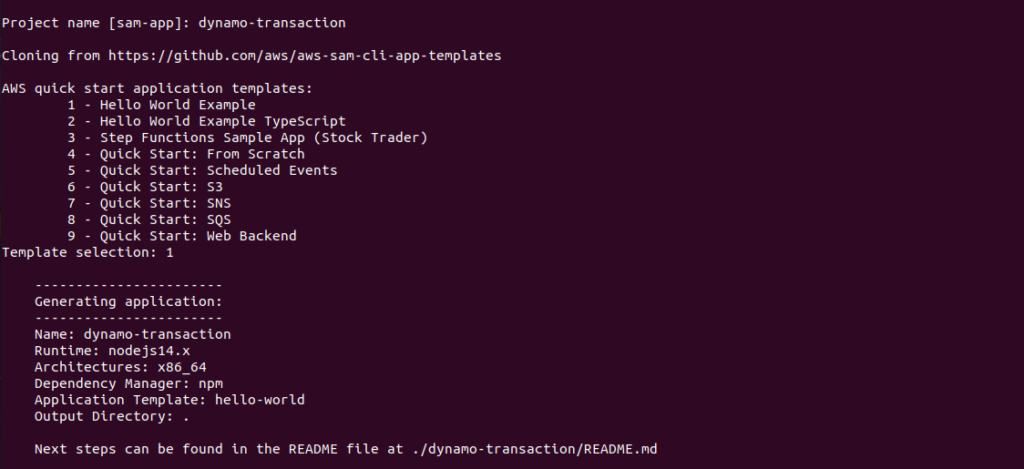
This will generate a basic SAM folder structure as in the image below.
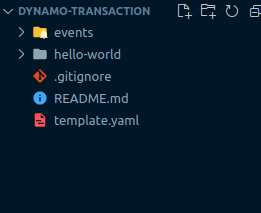
Now let’s install the AWS package inside the hello-world
folder. In lambda, each module is independent of the other so, all the dependent packages should be installed in each and every module.
npm i aws-sdk
Now let’s modify our app.js
file so that we can perform the database transaction operation.
#app.js
const AWS = require("aws-sdk");
const dynamoDB = new AWS.DynamoDB.DocumentClient({ region: 'us-east-1' });
exports.lambdaHandler = async (event, context) => {
try {
const params = {
TransactItems: [
{
Put: {
TableName: "User",
Item: {
id: '777',
name: 'Dummy User'
}
},
},
{
Put: {
TableName: "Role",
Item: {
id: '123',
name: 'Admin'
}
},
},
],
};
await dynamoDB.transactWrite(params).promise();
return {
'statusCode': 200,
'body': JSON.stringify({
message: "User data created successfully",
})
}
} catch (err) {
console.log(err);
return err;
}
};
Here, the params
variable consists of the transaction operation to be performed on the database. Then the await dynamoDB.transactWrite(params).promise();
statement will create user and role related data in the respective table if both operations are successfully completed. After the completion of the transaction operation success, the response will be returned to the user.
The following action can be performed on transactionWrite operation.
- Put – Insert an item into the table.
- Update – Updates an item from the table.
- Delete – Deletes an item from the table.
- ConditionCheck – Checks that an item exists or checks the condition of specific attributes of the item.
Among the above actions, we can group multiple actions and perform the transaction as a single transaction operation.
There are some scenarios where DynamoDB cancels the transactWrite
operation. Some are:
- If the parameters provided are in invalid format.
- A table in the
request is in a different account or region.transactWrite
- There is an insufficient provisioned capacity for the transaction to be completed.
- An item size becomes too large (larger than 400 KB), or a local secondary index (LSI) becomes too large.
Conclusion
In this article, we’ve learned how to implement transactions in dynamodb using AWS SAM.