In this lesson, we will learn about different types of javascript while loop. While loops are common types of loops that repeat a set of instructions depending on a condition. The code inside while statements will continue to run as long as the condition returns to true. These loops are used when the exact number of times a code needs to be executed is unknown.
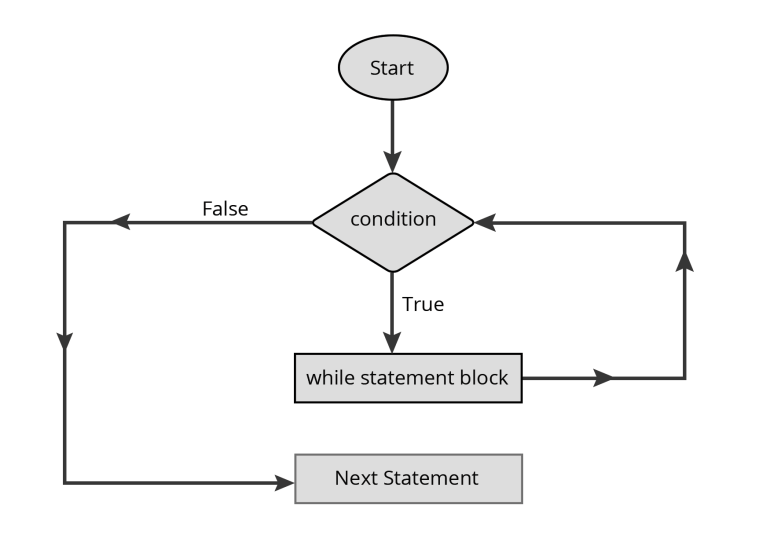
Types of Javascript While Loop
Simple While Loop
A simple while loop statement checks the condition before executing the statements inside the while block. These statements are executed as long as the condition returns true. The while loop is referred to as a pretest loop because it evaluates expressions before every iteration.
Syntax,
while (condition) {
// statements to be executed
}
Example,
let count = 1;
while (count < 4) {
console.log("Current Count : " , count);
count++;
}
Output:
Current Count : 1
Current Count : 2
Current Count : 3
Here, the while loop first evaluates the condition i.e. 1 < 4
which returns true so, executes the console.log command. This process is repeated until the condition returns false.
Do While Loop
The do-while loop is similar to the while loop statement except for the part where the statement evaluates the condition. The do-while loop evaluates the condition only after a statement is executed. This means a statement is executed at least once even do the condition returns false on the first evaluation.
Syntax,
do {
// statement to be executed
}while (condition);
Example,
let count = 1;
do {
console.log("Current Count : " , count);
count++;
} while (count < 4);
Output,
Current Count : 1
Current Count : 2
Current Count : 3
Here, the do-while loop logs the first value i.e. Current Count : 1
without evaluating the condition. The second statement is only executed if the condition returns true.
Conclusion
In this article, we learn about different types of javascript while loops available with the help of some examples. To learn more about javascript visit here.