In this lesson, we will learn about the if-else statement available in javascript with the help of examples. Basically, the if-else statements are used when we want to write commands that handle different decisions in your code. The if-else statement will perform some action for the specific condition. If the condition meets then the particular block of action will be executed otherwise it will execute another block of action that satisfies that particular condition. The if-else statement is also known as a conditional statement. There are different conditional statements available in javascript. They are:
- if statement
- if-else statement
- if-else if statement
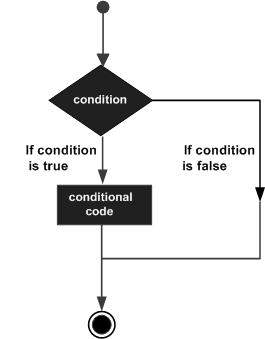
if statement
In JavaScript, the if statement
is the most fundamental control statement that allows decisions to be made and statements to be executed conditionally.
Syntax,
if (condition) {
// Statements to execute if condition is true
}
Here, the statements inside the if block will be executed only if the condition returns true.
Example,
// check if the number is positive
const number = prompt("Enter a number: ");
// check if number is greater than 0
if (number > 0) {
// the body of the if statement
console.log("The number is positive");
}
console.log("The if statement is easy");
Here, if the value of the number is greater than 0 the output will be
The number is positive
The if statement is easy
if-else statement
The if-else statement
execute a statement under the if block if the condition is true and executes a statement under the else block if the condition returns false.
Syntax,
if (condition) {
// Statements to execute if condition is true
}else {
// Statements to execute if condition is false
}
Here, the statements inside the if block will be executed if the condition returns true else the statements inside the else block will be executed.
Example,
const age = prompt("Enter a age: ");
if (age >= 18) {
console.log('You can sign up.');
} else {
console.log('You must be at least 18 to sign up.');
}
Here, if the user enters the age whose value is greater than or equal to 18 the output will be You can sign up.
else You must be at least 18 to sign up.
if-else if statement
A if-else if statement
is an advanced version of an if-else statement that allows JavaScript to decide a correct path based on several conditions.
Syntax,
if (condition 1) {
Statement(s) to be executed if condition 1 is true
} else if (condition 2) {
Statement(s) to be executed if condition 2 is true
} else if (condition 3) {
Statement(s) to be executed if condition 3 is true
} else {
Statement(s) to be executed if no condition is true
}
Here, the statements inside the first if block will be executed if condition 1 returns true, the statements inside the second if block will be executed if condition 2 returns true, and so on. if no condition returns true the statements inside the else block will be executed.
Example,
const number = prompt("Enter a number: ");
if (number > 0) {
console.log("The number is positive");
} else if ( number === 0){
console.log("The number is 0");
} else{
console.log("The number is negative");
}
Here, if the value of the number is greater than 0 the output will be The number is positive
, if the number is equal to 0 the output will be The number is 0
else the output will be The number is negative
Conclusion
In this lesson, we learn about the Javascript if-else statements with some examples.