In this lesson, we will learn about javascript break statement and how to use it. Break statements terminate the current loop, or switch, statement, and transfer control to the statement following the terminated statement. Syntax,
break [label];
Here, the label
is optional which denotes a label name of the statement. The labeled break statement is rarely used in javascript.
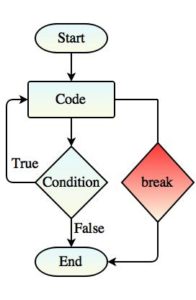
Example 1: Break in while loop
let i = 1;
while ( i < = 5 ) {
if (i == 3) {
break;
}
console.log("Value of i: " , i ) ;
i ++;
}
In the above example, the while loop will be terminated when the value of i is equals to 3
. The output will be as shown below:
Value of i: 1
Value of i: 2
Example 2: Break in for loop
for (let i = 1; i <= 5; i++) {
// break condition
if (i == 3) {
break;
}
console.log("Value of i: " , i ) ;
}
In the above example, the for loop will be terminated when the value of i is equals to 3
because the break statement is executed when the value of i will be 3, The output will be
Value of i: 1
Value of i: 2
Example 3: Break in switch case
var technology = 'JavaScript';
switch (technology) {
case 'SQL':
console.log('TechOnTheNet SQL');
break;
case 'JavaScript':
console.log('TechOnTheNet JavaScript');
break;
default:
console.log('Other TechOnTheNet technologies');
}
In the above switch statement example, the break statement is used to end the switch statement once a match is found.
Conclusion
In this article, we learn about the javascript break statement and how to use it with the help of some examples. To learn more about javascript visit here.