From this article, you would be able to learn how to setup MongoDB and Node.js in Linux (Ubuntu)
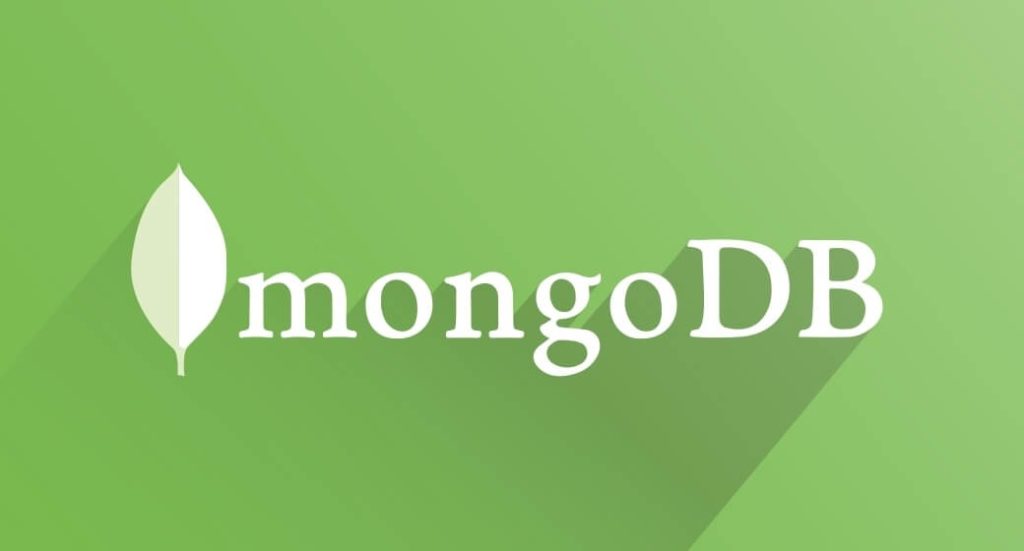
Installing MongoDB
MongoDB is a cross-platform document-oriented database. Classified as a NoSQL database program, MongoDB uses JSON-like documents. It uses the BSON data format and puts data in collections that are present inside databases. Schemas define a collection. The MongoDB is created and maintained by MongoDB Inc.
Step 1: Setup
Add public key which contains packages for installing MongoDB
sudo apt install gnupg
wget -qO - https://www.mongodb.org/static/pgp/server-5.0.asc | sudo apt-key add -
Create a list file for MongoDB
echo "deb [ arch=amd64,arm64 ] https://repo.mongodb.org/apt/ubuntu focal/mongodb-org/5.0 multiverse" | sudo tee /etc/apt/sources.list.d/mongodb-org-5.0.list
Reload local packages
sudo apt update
Installing MongoDB’s latest (stable version)
sudo apt-get install -y mongodb-org
For troubleshooting problems, see the MongoDB troubleshooting guide.
Running MongoDB community edition
sudo systemctl start mongod
## verify if the MongoDB has started successfully
sudo systemctl status mongod
## want to start MongoDB on system reboot
sudo systemctl enable mongod
Stopping and restarting MongoDB
## stop
sudo systemctl stop mongod
## restart
sudo systemctl restart mongod
Step 2: Using MongoDB
## connect to the MongoDB using mongosh *connects to the default port 27017.
mongosh
Now we are getting to the local MongoDB on default port 27017.
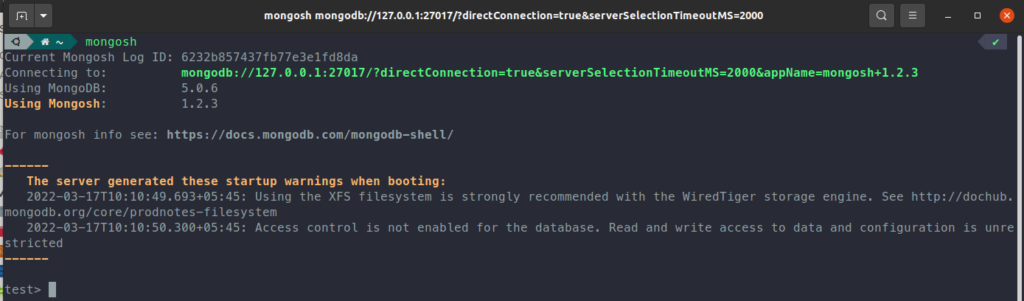
Testing the Database by running some queries
## creating a database
use scanskill
## creating a collection named user
db.createCollection("user");
## inserting a data to the collection user
db.user.insertOne({name: "Hello World", age: 22, position: "dev"});
## finding all data present inside the user collection
db.user.find({});
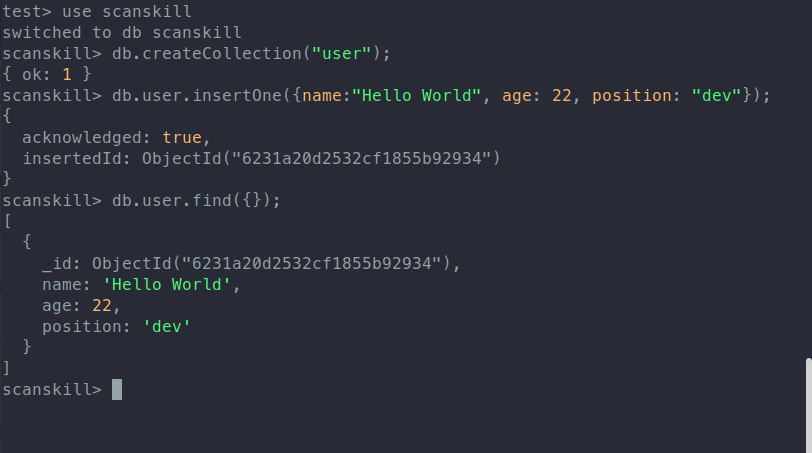
Testing the database setup, which was successful.
As we can see, inserting data, retrieving, and visualization as a whole becomes boring while looking at it from the shell. If you want a graphical interface you can install MongoDB compass, if not you can skip the following step.
Step 3: MongoDB Compass Download and Installation
Download compass by going to the following link: MongoDB Compass
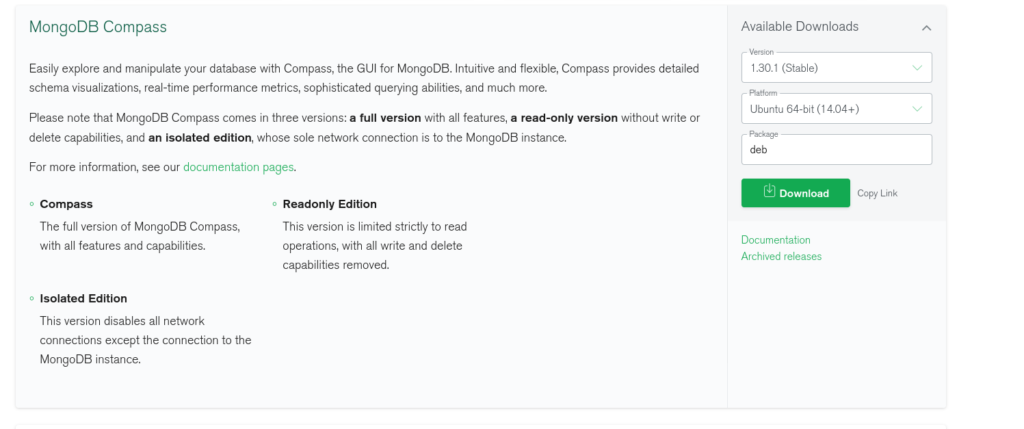
Install the deb package
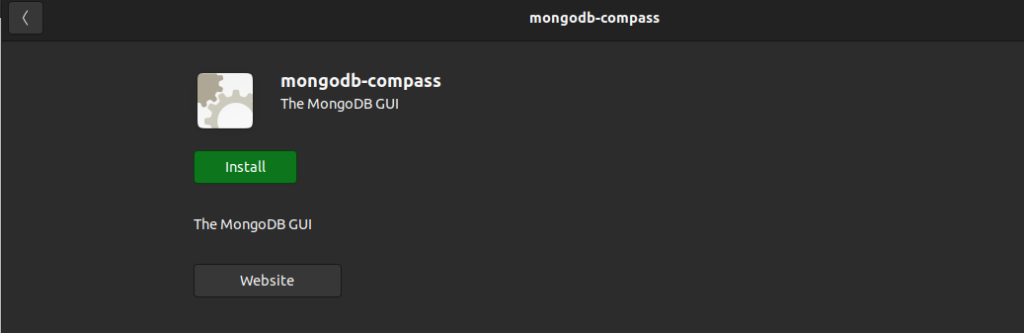
After installation open the compass application
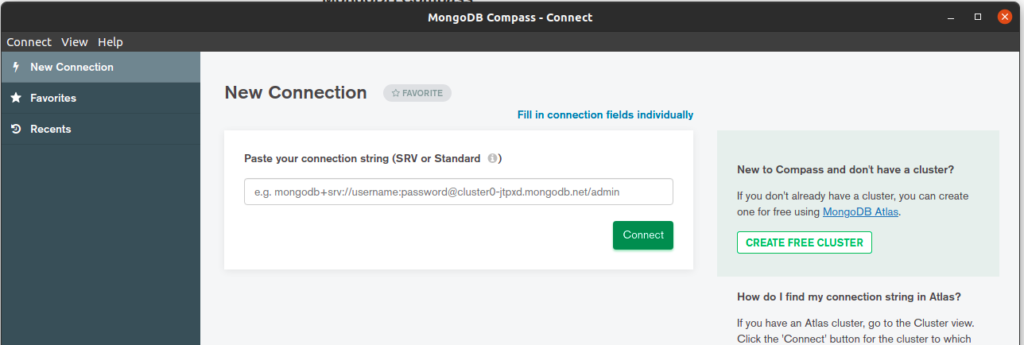
Now open your terminal and type mongosh
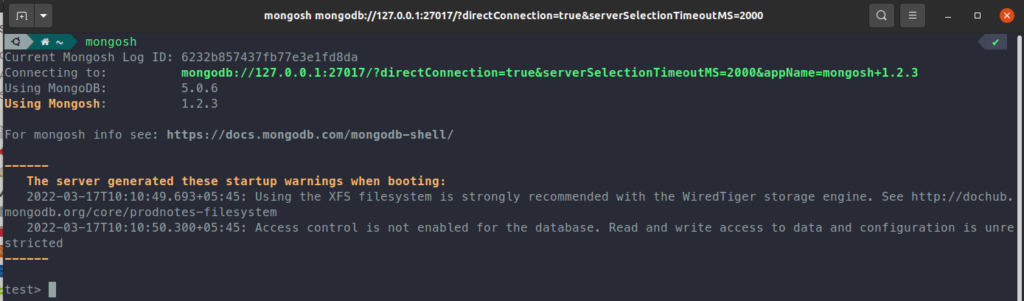
Not put the above obtained connecting to: mongodb://***
link in the MongoDB Compass.
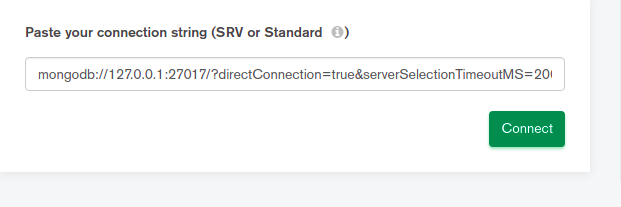
There you have it you are successfully connected to the MongoDB through the compass application. Now you can interact with your MongoDB database through the graphical user interface.
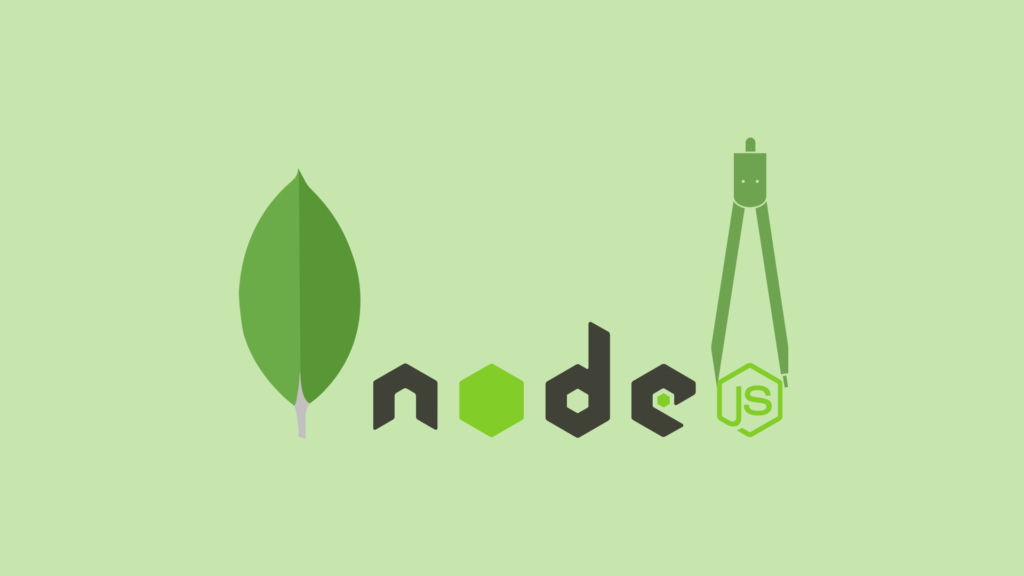
Installing Node.js
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes Javascript code outside the browser. Node.js assists in writing code that runs on the server side, making it possible for running JavaScript on the server.
Step 1: Setup
Although Ubuntu provides Node.js in its default repository, it is not the latest stable version. So, for setting up the latest stable version of the node we install it through the node source.
# Using Ubuntu
curl -fsSL https://deb.nodesource.com/setup_17.x | sudo -E bash -
sudo apt-get install -y nodejs
The latest version as of now is 17. For releases look at the node source repository, it also comes with detailed installation guide for other operating systems.
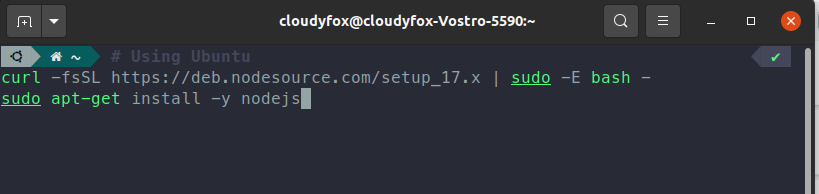
After installation
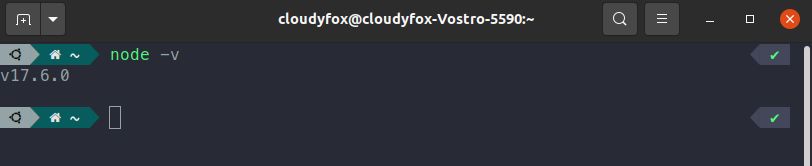
Now that node is successfully installed on your machine, we go through the next step which is optional. As we have installed both MongoDB and Node.js, why not perform a basic operation from Node.js to the MongoDB database. As this is optional you skip it.
Querying MongoDB Through Node.js
Step 1: Create a Node.js project
## Creating and going into the file
md MongoNode && cd MongoNode
## creating a node project
npm init -y
## creating a index.js file
touch index.js
## installing needed dependencies
npm i mongoose
## opening with your favourie code editor
code .
We use Mongoose as it is a MongoDB object modeling tool designed to work in an asynchronous environment. Mongoose supports both promises and callbacks. We use mongoose instead of mongodb which is the official driver for MongoDB.
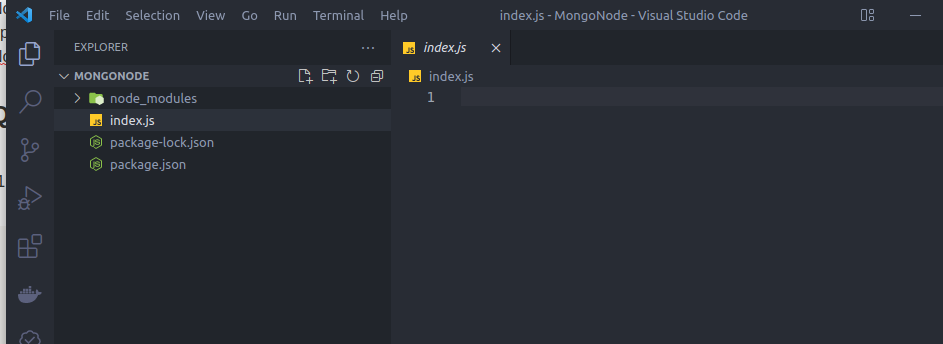
Step 2: Coding
Connecting to the database
const mongoose = require("mongoose");
//* this url can be recieved by mongosh connecting to: ${string} trough the terminal
const mongodbUrl = `mongodb://127.0.0.1:27017/?directConnection=true&serverSelectionTimeoutMS=2000&appName=mongosh+1.2.3`;
//* connecting to the mongodb database
function dbConnect() {
mongoose.connect(mongodbUrl, (err) => {
if (!err) {
console.log("Connected to the Mongo Database.");
} else {
console.log(
`[${err}, Connection with the MongoDB could not be established.]`
);
}
});
}
dbConnect();
Creating a schema for user collection
//* creating a collection
const schema = new mongoose.Schema(
{
_id: mongoose.Schema.Types.ObjectId,
name: {
type: String,
},
email: {
required: true,
type: String,
},
password: {
required: true,
type: String,
},
},
{ versionKey: false },
{ strict: false }
);
const User = mongoose.model("User", schema);
In the above schema, the collection User is made. The user has keys _id which is the default uuid, name which is a String, email is string and is required, it’s the same with the password.
Inserting data
//* Inserting a data to the database
function insertNewUser() {
const newUser = {
_id: new mongoose.Types.ObjectId,
name: "Hello World!",
email: "hello@scanskill.com",
password: "veryStrongP@ssword123"
};
const user = new User(newUser);
//* Saves the data to the database
const dataSaved = user.save();
if(dataSaved) {
console.log(newUser);
}
};
insertNewUser();
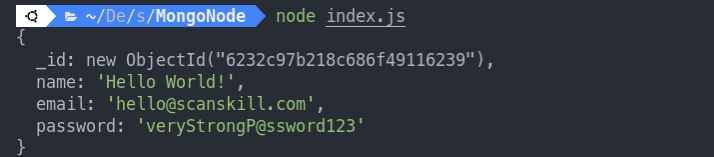
Looking data from the compass:
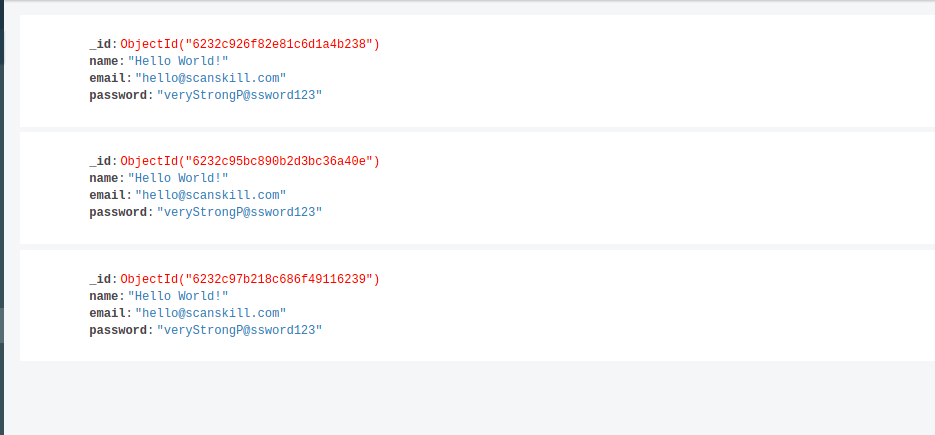
Fetching data from the database
//* Fetching all data from the database
function getAllUsers() {
User.find((err, result) => {
if(!err) {
console.log(result);
}
});
}
getAllUsers();
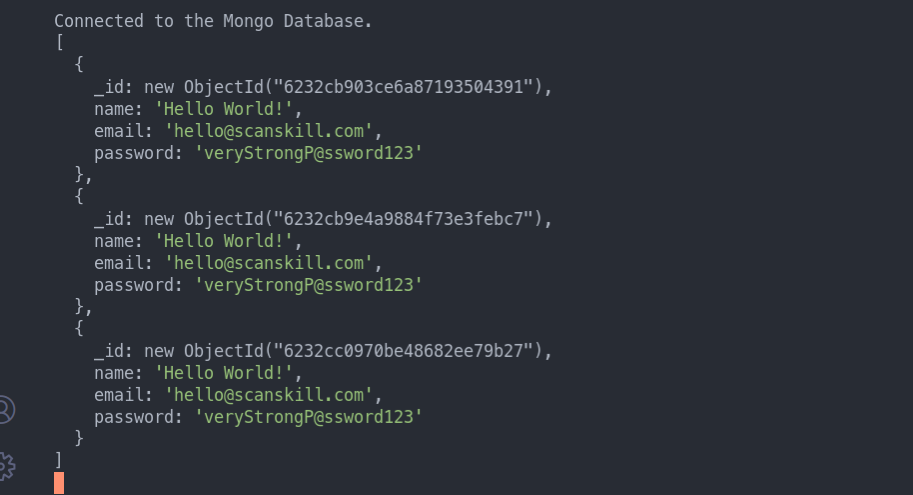
Conclusion
Congratulations! you have installed MongoDB successfully, MongoDB Compass, and Node.js on Linux. In this article, we looked into installing MongoDB, Compass, and Node.js.