Express is a Node.js web application framework that provides a robust set of features for building web applications. Despite being a framework itself, there are many others based on it. Some of the famous ones are:
- ItemsAPI: Search backend for web and mobile applications built on Express and Elasticsearch.
- Kraken: Secure and scalable layer that extends Express by providing structure and convention.
- Sails: MVC-based framework for Node.js for building practical, and applications ready for production.
- Hydra-Express: Hydra-Express is a lightweight library that facilitates building Node.js Microservices using ExpressJS.
- graphql-yoga: Fully-featured, yet simple and lightweight GraphQL server.
- Express Gateway: Fully-featured and extensible API Gateway using Express as a foundation.
- NestJs: A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications on top of TypeScript & JavaScript (ES6, ES7, ES8).
Express-validator
Express-validator is a set of express.js middlewares that wraps validator.js validator and sanitizer functions. A middleware is a function that has access to the request and response object before the called method runs. We will follow some steps while taking a look at different aspects of validation:
- Writing validation rules.
- How it works.
- Writing custom validation messages.
Step 1: Initializing an express application
Create a project and install the required packages
## initializing a application
mkdir express_validator && cd express_validator
npm init -y
## installing required dependencies
npm i express express-validator
## open on your favorite code editor
code .
express application (basic user login and register functions)
const express = require("express");
const PORT = process.env.PORT || 3000;
const app = express();
app.use(express.json());
app.post("/register", (req, res) => {
let { name, phone, email, password } = req.body;
res.status(200).json({
name: name,
phone: phone,
email: email,
password: password
});
});
app.post("/login", (req, res) => {
const { email, password } = req.body;
res.status(200).json({
email: email,
password: password
});
});
app.listen(PORT, console.log(`Server started on PORT -> ${PORT}`));
This basic code shows the values needed to add login and register functions to an application.
Step 2: Writing first validation.
We will write some rules for the register function and those are:
password
: 1 special, 1 capital, 1 small, 1 capital character, 1 number, and required.email
: should be an email and is necessary.name
: is necessaryphone
: not required
Let’s see how it looks on code and how it can be made by using express-validator
as middleware, we modify the above code
Modified code
const { body, validationResult } = require("express-validator");
//* validation rules
const registerUserValidationRules = () => {
return [
//* name is required and must be string
body("name").isString(),
//* email is required and must be a valid email
body("email").isEmail(),
//* password is required and must be strong password
body("password").isStrongPassword({
minLength: 8,
minLowercase: 1,
minUppercase: 1,
minNumbers: 1,
minSymbols: 1,
}),
//* phone is optional is must be a number
body("phone").isNumeric().optional(),
];
};
//* validated the variables present inside req.body according to above validation rules
const validateRegisterUser = (req, res, next) => {
const errors = validationResult(req);
if (errors.isEmpty()) {
return next(); // if no errors are found it proceeds to the next function
}
const extractedErrors = [];
errors.array().map((err) => extractedErrors.push({ [err.param]: err.msg })); // maps the error inside the extractedErrors array
return res.status(422).json({
errors: extractedErrors,
});
};
app.post("/register",registerUserValidationRules(), validateRegisterUser ,(req, res) => {
let { name, phone, email, password } = req.body;
console.log(
res.status(200).json({
name: name,
phone: phone,
email: email,
password: password
});
});
Explanation of the code
In the above code the registerUserValidationRules()
contains the validation rules that is to be looked at. Secondly the validateRegisterUser()
validates and checks if there are any errors present if errors are not present then it goes forward to the next()
which is the inside the app.post("/register")
route. app.post("/register",registerUserValidationRules(), validateRegisterUser ,(req, res)=>{});
, Here the validation rules and validate function are kept as middleware and run before code inside the register
route works;
Output
ERROR
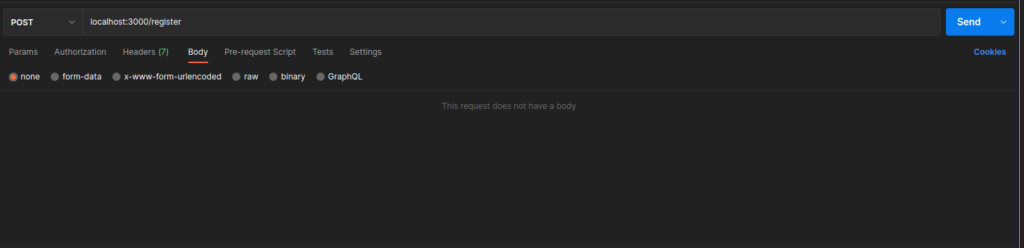
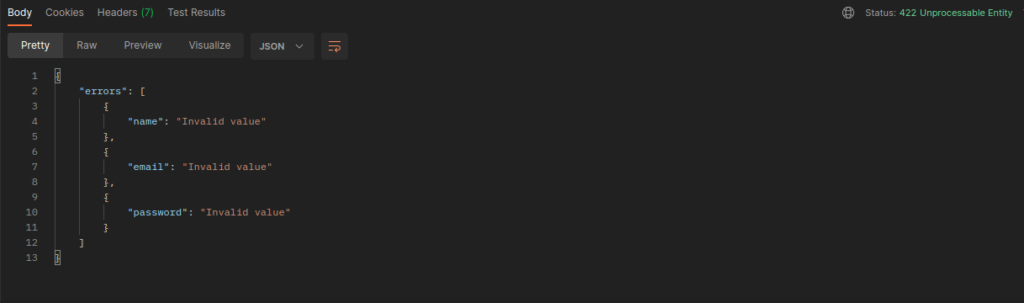
SUCCESS
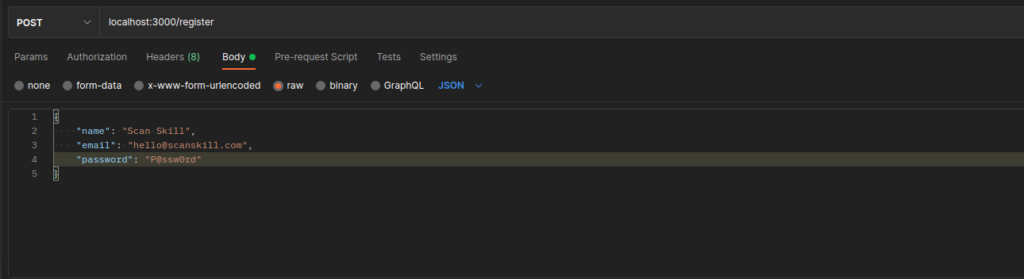

Step 4: Writing custom message for validation
For this, we chain withMessage()
at the end of every validation and write custom messages.
Code
const registerUserValidationRules = () => {
return [
//* name is required and must be string
body("name").isString().withMessage("Please provide a name."), //* on error this message is sent.
//* email is required and must be a valid email
body("email").isEmail().withMessage("Email is absolutely required."),
//* password is required and must be strong password
body("password").isStrongPassword({
minLength: 8,
minLowercase: 1,
minUppercase: 1,
minNumbers: 1,
minSymbols: 1,
}).withMessage("Not a valid password."),
//* phone is optional is must be a number
body("phone").isNumeric().optional(),
];
};
Output
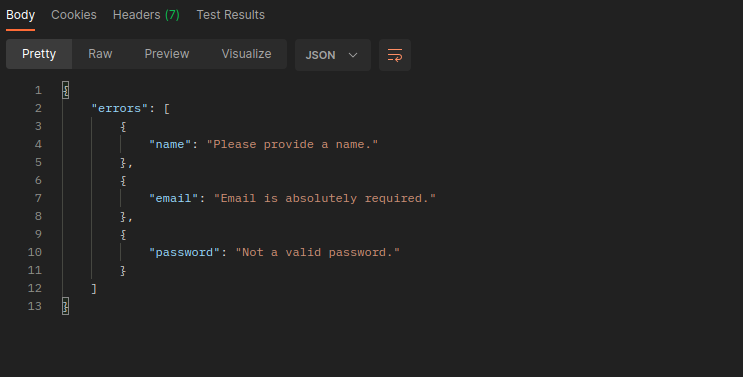
Invalid value
Conclusion
The article viewed how the express-validator
is used side by side with the express application. Also, the article viewed how to use express-validator on express. application. We also implemented validation in the register route and examined how to write custom validation messages.